Shaping User Journey: Regulating Component Focus with ReactJS’s useFocus Hook
Welcome to a comprehensive guide on ReactJS’s `useFocus`, a powerful hook for managing component focus state. It streamlines focus management, contributing significantly to interactivity and user-friendliness, thereby enhancing user experience and accessibility. In this guide, you’ll delve into `useFocus` basics, practical implementation, benefits, and use cases. You’ll also learn to compare it with other focus management methods in ReactJS, understand best practices, and navigate common challenges. Whether you’re an experienced developer or a ReactJS beginner, this guide will enhance your skills in managing component focus state effectively, leading to the creation of dynamic, user-friendly, and accessible web applications.
Understanding ReactJS useFocus
ReactJS’s `useFocus` is a custom hook that simplifies managing component focus state within your applications. It encapsulates the functionality needed to assign and manipulate focus, an essential part of making an application interactive and user-friendly.
In essence, `useFocus` operates by providing a reference (`ref`) to the component that needs to receive focus. This `ref` can then be assigned to the `ref` attribute of the component in your JSX code. When your application runs, React will automatically ensure that this component receives focus when necessary.
The use of `useFocus` is most evident in situations where you need to programmatically control the focus. For example, when a modal dialog opens, setting focus to it aids in accessibility, particularly for keyboard and screen reader users.
In its simplest form, the `useFocus` hook looks something like this:
const [ref, focused] = useFocus();
Here, `useFocus()` returns two items – `ref`, which you assign to the JSX component that needs to receive focus, and `focused`, a boolean value indicating whether the component currently has focus.
In subsequent sections of this guide, we’ll delve deeper into how to use `useFocus`, and we’ll provide examples and scenarios where `useFocus` comes in handy. We’ll also address the benefits and best practices of using this hook, along with solutions to some common challenges you may encounter.
Deep Dive into useFocus
To fully leverage the potential of the `useFocus` hook in ReactJS, let’s dig deeper into its intricacies.
The `useFocus` hook is essentially a function that returns an array of two items: `ref` and `focused`. `ref` is a reference to the component you want to receive focus. `focused` is a boolean that tells you whether the component is currently focused.
Here’s a simple usage example:
import React from 'react'; import useFocus from './useFocus'; // assuming useFocus is defined in this file function MyComponent() { const [inputRef, isFocused] = useFocus(); return ( <input ref={inputRef} type="text" /> ); } export default MyComponent;
In the above code, `useFocus` is used to manage the focus state of the input field. We first destructured the array returned by `useFocus` into `inputRef` and `isFocused`. The `inputRef` is then assigned to the `ref` attribute of the input component. The `isFocused` variable can be used anywhere within the component to check if the input field is currently focused.
Internally, `useFocus` uses the `useRef` and `useEffect` hooks provided by ReactJS to control focus state. `useRef` is used to create the `ref` for the component, and `useEffect` is used to update the focus state when the component is mounted or updated.
The beauty of `useFocus` lies in its ability to encapsulate focus management logic, freeing developers from manually handling focus states and enabling them to focus on building other functionalities of the application.
It’s important to note that `useFocus` is not a built-in React hook, and thus you may need to create it yourself or find a custom implementation online. In later sections, we’ll walk you through the step-by-step process of creating this hook and provide some best practices to follow when using `useFocus`.
Benefits of using useFocus
`useFocus` in ReactJS offers numerous advantages, such as improving the user interface and experience, simplifying focus management, and enhancing accessibility. It facilitates programmable focus control, essential for interactive elements like modals and menus. It makes focus management more straightforward compared to traditional methods, leading to cleaner code. `useFocus` significantly contributes to accessibility, easing focus control, especially beneficial for assistive technology users.
This hook promotes code reusability and provides greater control by enabling both the setting and tracking of component focus. By integrating `useFocus` into your React applications, you can develop more interactive, user-friendly, and accessible web apps.
Comparing useFocus with other ways to manage focus in ReactJS
ReactJS offers multiple methods to manage focus, and while `useFocus` brings simplicity and reusability to the table, it’s useful to understand how it compares to other approaches. Let’s discuss a few:
- Using Refs and Imperative Focus Methods: Before the introduction of hooks, managing focus in React involved creating a ref using `React.createRef()` or callback refs, and then using the imperative `focus()` method to set focus. While this method provides direct control over the focus, it tends to be verbose and can lead to cluttered code in complex applications. Comparatively, `useFocus` provides a cleaner, more streamlined approach.
- Using Third-Party Libraries: Libraries like `react-focus-lock` or `focus-trap-react` provide robust focus management, especially for complex components such as modals or dropdowns. These libraries are powerful and can handle more complex focus scenarios, but they may be overkill for simpler needs. `useFocus`, on the other hand, is a lighter solution that could be sufficient for less complex focus management tasks.
- Using the tabIndex Attribute: The `tabIndex` attribute can control the order of element focus. While it’s a native HTML attribute and works without additional code, using it excessively or incorrectly can disrupt the natural tab order and harm accessibility. `useFocus` doesn’t affect tab order, and instead provides programmatic control over focus, which is a more reliable and accessible approach.
Each of these methods has its place depending on the specific requirements of your project. `useFocus` stands out for its simplicity, reusability, and how it abstracts away the complexities of focus management, making it an excellent tool for developers. However, it’s crucial to assess the needs of your application before deciding which approach to take.
Best Practices for using useFocus
Just like any tool or method in web development, it’s essential to understand and follow best practices while using the `useFocus` hook in ReactJS. Here are a few key practices:
- Use for Complex and Compound Components: `useFocus` is extremely useful for managing focus in complex or compound components, such as modals, dropdowns, or custom select boxes. Using `useFocus` can help you to make these components more accessible and user-friendly.
- Maintain Natural Tab Order: Although `useFocus` can programmatically control focus, it’s essential to maintain a logical tab order for keyboard navigation. Use `useFocus` to enhance the user experience, not to override the natural tab order unless it’s necessary for a specific interaction.
- Ensure Accessibility: When using `useFocus`, always remember the broader goal – to improve accessibility. Make sure that the focus is visible and that it’s directed to the correct component at the right time. This is especially important when creating interactive components like modals. When a modal opens, the focus should move to the modal, and when it closes, the focus should return to the element that triggered it.
- Test Focus Behavior: It’s important to test the focus behavior in your ReactJS applications. Ensure that the focus is behaving as expected in different scenarios, particularly when dealing with dynamic content or complex interactions. This will help catch any accessibility issues early in the development process.
- Don’t Overuse: Although `useFocus` can simplify focus management, it’s essential not to overuse it. Not all elements need to be programmatically focusable. Elements like buttons, links, and form controls are naturally focusable. Overuse of `useFocus` can lead to confusing interactions.
By following these best practices, you can effectively use `useFocus` to enhance the usability and accessibility of your ReactJS applications, creating a better experience for all users.
Common Challenges and Solutions
While using `useFocus` simplifies focus management in ReactJS applications, developers may still encounter some challenges. Let’s discuss these challenges and their possible solutions:
- Managing Focus with Multiple Input Elements: In forms with multiple input elements, it’s crucial to ensure that the focus transitions logically from one input to the next. While `useFocus` can be used on individual elements, managing the focus order may still be challenging. In such cases, consider maintaining a natural tab order and using `useFocus` to handle focus on form entry or exit, rather than on every individual element.
- Ensuring Correct Focus When Dynamic Content Is Loaded: For dynamically loaded content, such as a list loaded on a button click, it might be challenging to manage focus using `useFocus` as the elements to be focused aren’t available during the initial render. To handle this, you could use the `useEffect` hook to monitor changes in the dynamic content and then apply `useFocus` accordingly.
- Keeping Track of Focus State in Complex Components: In complex components like modals or custom dropdowns, tracking the focus state could become complicated. This could be especially tricky when these components have multiple interactive child elements. One way to handle this is by using a combination of `useFocus` and context to manage and track the focus state throughout the component hierarchy.
- Preventing Unnecessary Focus: Sometimes, you might want to prevent an element from gaining focus under certain conditions, such as a disabled button. However, `useFocus` could still cause these elements to be focusable. A solution is to conditionally apply `useFocus` based on the state of the element.
- Browser Inconsistencies: Browsers can sometimes handle focus behavior inconsistently, particularly when dealing with complex scenarios like focus trapping or managing focus in iframes. These inconsistencies may require additional logic or workarounds when using `useFocus`. Always thoroughly test focus behavior across different browsers to ensure a consistent user experience.
While these challenges can make focus management seem daunting, they can be navigated effectively with a good understanding of `useFocus` and how focus works in the web platform. The result is a more accessible and user-friendly application.
Conclusion
In conclusion, `useFocus` is a vital tool in ReactJS for managing component focus state, offering a simpler, reusable method to enhance user interfaces and experiences. This guide explored `useFocus` comprehensively, its benefits, comparisons with other methods, best practices, and solutions to common challenges, equipping you with the knowledge to manage component focus state effectively. It’s essential to use `useFocus` wisely to enhance natural focus behavior, maintain accessibility, and conduct thorough testing. Whether you’re an experienced developer or new to ReactJS, `useFocus` is a powerful addition to your toolkit, aiding you in creating more interactive, user-friendly, and accessible web applications.
Table of Contents
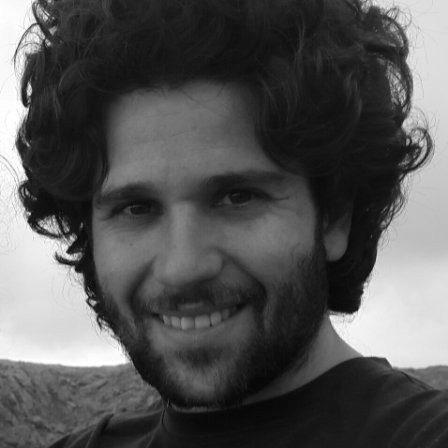
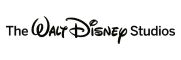