Leverage ReactJS’s useGeoLocation hook to access and utilize user geolocation data in your components
ReactJS, also simply known as React, is an open-source JavaScript library developed by Facebook for building dynamic user interfaces. Since its inception in 2013, it has revolutionized the way we build web applications, offering a component-based architecture that enables developers to create large web applications which can update and render efficiently in response to data changes.
In this blog post, we will explore how to harness the power of geolocation in your React applications using the useGeoLocation hook. We’ll walk you through the setup process, how to incorporate geolocation in a React component, handle permissions, and error scenarios. Whether you’re a novice or an experienced developer, by the end of this post, you’ll be well-equipped to enhance your React apps with geolocation capabilities.
Explanation of Geolocation and Its Importance
Geolocation is a technology used to identify the geographical location of a device or a user. It can provide the latitude and longitude coordinates, and in more precise instances, data such as altitude, speed, or the bearing can also be determined.
In the digital age, geolocation plays a crucial role due to its ability to provide context-specific services based on a user’s location. Whether it’s for showing the nearest cafes in a food delivery app, tailoring content to local languages and interests, offering region-specific discounts in ecommerce platforms, or enabling real-time tracking in logistics, geolocation brings a new dimension of user personalization and business intelligence to the table.
Accessing Geolocation Data in the Browser
The most common way to access geolocation data in the browser is through the Geolocation API, a standard set by the W3C. This API is integrated into most modern web browsers and allows websites to request a user’s geographical location. The process is quite straightforward:
- First, the application checks if the Geolocation API is available by testing `if (“geolocation” in navigator)`.
- If the API is available, the application can then call the `navigator.geolocation.getCurrentPosition()` method. This method will prompt the user to allow or deny location access.
- If the user allows access, the method will return an object containing the latitude and longitude of the user’s position.
Remember, accessing geolocation data requires explicit permission from the user to respect privacy norms and regulations. If a user denies the access request, the application should be designed to degrade gracefully or provide alternate functionality.
Introduction to useGeoLocation in ReactJS
As you may know, ReactJS, unlike traditional libraries or frameworks, utilizes a concept called hooks. Hooks are a new addition to React that lets you use state and other React features without writing a class. They make it possible to take a React function component and add state to it, or hook into lifecycle methods like componentDidMount or componentDidUpdate.
Now, when we talk about geolocation, the `useGeoLocation` is not a built-in hook in React, unlike `useState` or `useEffect`. Instead, it’s a custom hook that’s designed specifically to integrate the browser’s Geolocation API with a React component. By abstracting the logic for accessing geolocation data into a reusable function, we can keep our components lean and easy to understand.
This hook simplifies the process of getting the user’s geolocation information, and can be used in any functional component. It also takes care of the necessary browser API checks, handles permissions, and provides a seamless way to update your component whenever the geolocation data changes.
In the upcoming sections, we will learn how to set up your React environment, create a `useGeoLocation` custom hook, and use it to access and utilize user’s geolocation data in a React application.
Setting Up the ReactJS Environment
To set up a ReactJS environment, you will first need to have Node.js and npm (Node Package Manager) installed on your computer. Here’s a brief guide on how to do this:
Step 1: Install Node.js and npm
- Visit the official Node.js website (https://nodejs.org/) and download the latest stable version of Node.js.
- Once downloaded, run the installer and follow the prompts to install Node.js and npm.
Step 2: Install Create React App
The Create React App tool is a comfortable way to set up a new React application. Install it globally by running the following command in your terminal:
npm install -g create-react-app
Step 3: Create a New React Application
Now, you can create a new React application using the Create React App tool. Navigate to the directory where you want your project to live, then run the following command (replace `my-app` with the name of your app):
npm install -g create-react-app
Step 4: Navigate to Your React App’s Directory
Change your current directory to the new app’s directory using the command:
cd my-app
Step 5: Start Your React App
You can start your new React application by running:
npm start
After running this command, your app should be live at http://localhost:3000 in your web browser.
There you have it! You’ve successfully set up your ReactJS environment and created a new application. You’re now ready to begin developing with React.
Using useGeoLocation in a ReactJS Application
Before we proceed, it’s important to note that `useGeoLocation` is not a built-in React hook. It’s a custom hook, which means we need to define it ourselves. The following is a guide to creating a `useGeoLocation` hook and using it in a React component:
Step 1: Creating the useGeoLocation Hook
In your React project, create a new file called `useGeoLocation.js` and define the `useGeoLocation` hook:
import { useState, useEffect } from 'react'; const useGeoLocation = () => { const [location, setLocation] = useState({ loaded: false, coordinates: { lat: "", lng: "" }, }); const onSuccess = (location) => { setLocation coordinates: { lat({ loaded: true, : location.coords.latitude, lng: location.coords.longitude, }, }); }; const onError = (error) => { setLocation({ loaded: true, error: { code: error.code, message: error.message, }, }); }; useEffect(() => { if (!("geolocation" in navigator)) { onError({ code: 0, message: "Geolocation not supported", }); } navigator.geolocation.getCurrentPosition(onSuccess, onError); }, []); return location; }; export default useGeoLocation;
The `useGeoLocation` hook uses the Geolocation API’s `getCurrentPosition` method to get the user’s location. It manages the location data and any potential errors with `useState` and updates them with the `onSuccess` and `onError` functions.
Step 2: Using the useGeoLocation Hook in a Component
Now, we can use the `useGeoLocation` hook in a component:
import React from 'react'; import useGeoLocation from './useGeoLocation'; const GeoLocatedComponent = () => { const location = useGeoLocation(); return ( <div> {location.loaded ? JSON.stringify(location) : "Location data not available yet"} </div> ); }; export default GeoLocatedComponent;
In this `GeoLocatedComponent`, we are importing and using our `useGeoLocation` hook. The component renders the location data once it’s loaded. If the data isn’t loaded yet, it displays a loading message.
And there you go! You’ve created a custom React hook to access geolocation data and used it in a component.
Practical Uses of useGeoLocation in ReactJS
The use of geolocation in web applications has skyrocketed in recent years, driven by the demand for more personalized user experiences. Here are some practical uses of the `useGeoLocation` hook in ReactJS:
- Location-Based Content
You can use geolocation to display content relevant to the user’s location. This could be local news, events, offers, or even language and cultural settings.
- Maps and Directions
If your application involves showing a map or giving directions, you can use geolocation to determine the user’s current position. This can be used in delivery apps, ride-hailing apps, or any app that includes navigation features.
- Location-Based Services
For apps offering services like food delivery, online shopping, or booking systems, you can use geolocation to show services available in the user’s location.
- Analytics and Marketing
Geolocation can provide valuable data for analytics and marketing. Understanding where your users are can help you understand user behavior and target your marketing efforts more effectively.
- Geofencing
Geofencing involves creating a virtual geographic boundary and sending notifications or alerts when a device enters or leaves this area. This could be useful for reminder apps, marketing notifications, security apps, and more.
Understanding Permissions and Handling Errors
When working with geolocation, it’s important to remember that accessing a user’s location is a sensitive action that requires explicit permission from the user. The browser will automatically prompt the user for permission when the `navigator.geolocation.getCurrentPosition()` method is called.
Handling Permissions
If the user grants permission, the browser will provide the location data. If the user denies permission, or if there are other issues like the user’s device not supporting geolocation, the browser will return an error.
Handling Errors
To handle these errors, the `getCurrentPosition()` method accepts a second callback function that will be executed if an error occurs. Here’s an example of how to use this error callback:
navigator.geolocation.getCurrentPosition(onSuccess, onError); function onSuccess(position) { // handle successful geolocation } function onError(error) { // handle errors switch(error.code) { case error.PERMISSION_DENIED: console.log("User denied the request for Geolocation.") break; case error.POSITION_UNAVAILABLE: console.log("Location information is unavailable.") Break; case error.TIMEOUT: console.log("The request to get user location timed out.") break; case error.UNKNOWN_ERROR: console.log("An unknown error occurred.") Break; } }
In this example, `onError` is a function that takes an error object as its argument. The error object has a `code` property that we can use to understand the nature of the error and handle it appropriately.
Remember, gracefully handling permission denials and errors is a crucial part of developing a great user experience. Your app should still function even if the user denies location access, and any error messages displayed to the user should be informative and easy to understand.
Testing the ReactJS Application
Testing is a crucial part of software development, ensuring your application behaves as expected. When it comes to testing a React application that uses geolocation, there are several key areas to focus on.
Step 1: Manual Testing
After you’ve implemented the `useGeoLocation` hook in your components, start with manual testing. Run your React application and check whether the geolocation data is being correctly fetched and displayed. Also, test how your application behaves when you deny the geolocation permission.
Step 2: Testing Geolocation Permission Prompt
Most modern browsers have developer tools that allow you to simulate various situations. For example, in Google Chrome, you can simulate different scenarios for location access in the Sensors tab (Developer Tools -> More tools -> Sensors). You can test how your application responds when the user grants or denies geolocation access, or when location information is unavailable.
Step 3: Unit Testing with Jest and React Testing Library
Jest, combined with React Testing Library, is a powerful tool for unit testing your components. You can mock the Geolocation API to simulate different responses and ensure your application handles them correctly.
Here’s an example of how to mock the Geolocation API in a Jest test.
global.navigator = { geolocation: { getCurrentPosition: jest.fn() .mockImplementationOnce((success) => Promise.resolve(success({ coords: { latitude: 51.1, longitude: 45.3 } }))) } };
With this setup, calling `navigator.geolocation.getCurrentPosition()` in your tests will return a fixed location.
Remember, testing your application thoroughly helps ensure a consistent and reliable user experience. It allows you to catch and fix bugs before they reach your users, and gives you confidence that your application is working correctly.
Conclusion
In this post, we’ve delved into the role of geolocation in React applications, demonstrating how to integrate this feature via the custom `useGeoLocation` hook. We’ve addressed user permissions, error handling, and testing, all critical for ensuring a secure, reliable, and user-friendly application. While integrating geolocation can significantly enhance personalization and usability, it’s vital to uphold ethical considerations and user privacy. The potential applications of geolocation are vast, opening exciting opportunities for developers. This tutorial aims to equip you with the necessary skills to leverage geolocation in your future React projects effectively.
Table of Contents
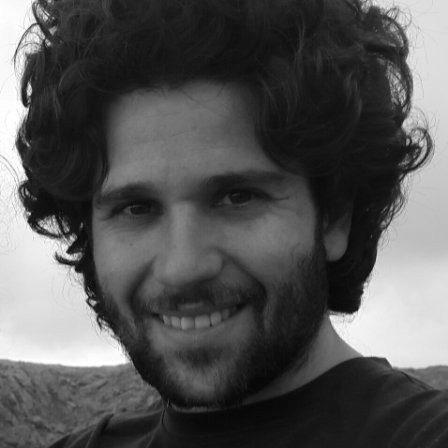
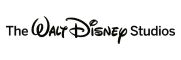