ReactJS with useHover to create interactive hover effects in your components
This introduction discusses the role of ReactJS in web development, focusing on its powerful component-based design that simplifies building complex user interfaces (UIs). As the demand for more intricate and engaging UIs increases, the need for improved interaction capabilities such as hover effects also rises. Hover effects enhance user experience by providing interactive elements and visual feedback.
This blog post centers around useHover, a React hook that manages hover states in components, making it easier to implement these hover effects. The guide is designed to provide practical insights into using useHover to build and improve hover effects in ReactJS components, serving both beginners and experienced developers.
Table of Contents
Basics of ReactJS and Component-Based Design
This passage provides an overview of ReactJS, a JavaScript library developed by Facebook for building interactive user interfaces. Unlike comprehensive frameworks like
Angular, ReactJS focuses solely on UI development, offering a fast and scalable solution. React’s key feature is its component-based architecture, where each component is a self-contained module managing its own state and rendering its UI. This design allows for code reusability and maintains code cleanliness.
React components have lifecycles managed through lifecycle methods such as `componentDidMount` and `componentWillUnmount`, granting control over a component’s behavior throughout its existence in the DOM. React also introduces `props` and `state` to manage data flow and changes in data respectively.
To enhance user interaction and responsiveness, React offers Hooks, special functions that allow you to utilize React’s state and lifecycle features in functional components. The useHover hook, to be discussed further, facilitates the implementation of hover effects in components.
Introduction to the useHover Hook
This excerpt introduces React Hooks, which are a significant enhancement in React 16.8 for establishing stateful logic and side effects in functional components. They enable developers to utilize state and other React features without writing a class. The `useHover` hook, a custom hook, is highlighted for its role in enhancing UI interactivity.
The `useHover` hook simplifies handling hover states in a React component. Hover effects are critical as they provide immediate visual cues to users when they hover over interactive elements, making the application more intuitive and aesthetically pleasing.
Implementing the `useHover` hook eliminates the need for a separate state variable to track the hover status or manually adding and removing event listeners, as the hook handles these tasks, simplifying component logic.
It’s important to note that `useHover` is a custom hook, meaning it’s developed by developers to address a specific need not covered by existing React hooks. The subsequent sections will discuss its implementation, provide advanced tips, and practical examples.
How to Implement useHover in a React Component
Implementing the `useHover` hook in your React components can be accomplished in a few straightforward steps. Let’s start with the creation of our `useHover` custom hook:
import { useRef, useState, useEffect } from "react"; function useHover() { const ref = useRef(); const [isHovered, setIsHovered] = useState(false); const enter = () => setIsHovered(true); const leave = () => setIsHovered(false); useEffect(() => { const refCopy = ref; refCopy.current.addEventListener("mouseenter", enter); refCopy.current.addEventListener("mouseleave", leave); return () => { refCopy.current.removeEventListener("mouseenter", enter); refCopy.current.removeEventListener("mouseleave", leave); }; }, []); return [ref, isHovered]; }
In this hook, `ref` is a reference to the element we want to detect hover events on. `isHovered` is a state variable that keeps track of whether the element is being hovered over. We add event listeners for `mouseenter` and `mouseleave` events in the `useEffect` hook.
Now that we have our `useHover` hook, we can use it in our component:
import React from "react"; import useHover from "./useHover"; function MyComponent() { const [hoverRef, isHovered] = useHover(); return ( <div ref={hoverRef}> {isHovered ? "Hovering over me!" : "Hover over me!"} </div> ); } export default MyComponent;
In this example, we use the `useHover` hook in `MyComponent`. We pass `hoverRef` as a `ref` prop to our `div`. If `isHovered` is `true`, we display the text “Hovering over me!”. If `isHovered` is `false`, we display the text “Hover over me!”.
The key advantage of using a `useHover` hook is that it encapsulates the logic for handling hover events, making your component code much cleaner and easier to understand. Additionally, since it’s a hook, you can reuse it in multiple components across your application.
In the next section, we’ll explore more advanced use cases of the `useHover` hook and learn how to customize hover effects with CSS.
Advanced Tips for Using useHover
While the `useHover` hook simplifies the creation of hover effects, here are some advanced tips to further leverage its capabilities and address potential issues:
- Combining with CSS for Customized Effects
In many cases, simple hover effects such as changing color or showing text can be implemented directly via CSS. However, when paired with the `useHover` hook, you can create much more dynamic effects. For instance, triggering animations, manipulating complex states, or loading data when the user hovers over a component.
Consider applying the hover state to a CSS class, and using that class to apply styles when `isHovered` is true:
function MyComponent() { const [hoverRef, isHovered] = useHover(); return ( <div ref={hoverRef} className={isHovered ? "hovered" : ""}> Hover over me! </div> ); }
Then in your CSS:
.hovered { background-color: blue; transition: background-color 0.5s ease; }
- Using with a Delay
Sometimes, you may want to delay the hover effect, especially for dropdown menus and tooltips. Here, a simple modification to the `useHover` hook can help:
function useHover(delay = 0) { const ref = useRef(); const [isHovered, setIsHovered] = useState(false); const timeoutRef = useRef(); const enter = () => { timeoutRef.current = setTimeout(() => setIsHovered(true), delay); }; const leave = () => { clearTimeout(timeoutRef.current); setIsHovered(false); }; // ... the rest of the hook remains the same ... }
This variant of `useHover` accepts a delay (in milliseconds), and only sets `isHovered` to `true` after that delay.
- Handling Complex Interactions
If you have nested elements that each use `useHover`, be aware that mouse events bubble up the DOM tree. You may need to use `event.stopPropagation()` in the event handlers to prevent an event on a child from triggering the parent’s event handler.
The `useHover` hook simplifies adding hover effects in your React applications. By understanding its mechanics and coupling it with these advanced techniques, you can create a rich, interactive user experience.
Use Case Examples of useHover in Action
Let’s explore a couple of practical examples where the `useHover` hook is used to enhance user interaction:
- Changing Image on Hover
Suppose you have a product image and you want to show a different angle or a zoomed-in image when the user hovers over it.
import React from 'react'; import useHover from './useHover'; function ProductImage() { const [hoverRef, isHovered] = useHover(); const imgSrc = isHovered ? '/images/product-zoomed.jpg' : '/images/product.jpg'; return ( <img ref={hoverRef} src={imgSrc} alt="Product" /> ); } export default ProductImage;
In this example, the `imgSrc` changes based on the hover state, displaying a different image when the user hovers over it.
- Showing Additional Information on Hover
Imagine you have a list of items, and you want to show additional details about each item when the user hovers over it.
import React from 'react'; import useHover from './useHover'; function Item({ name, details }) { const [hoverRef, isHovered] = useHover(); return ( <div ref={hoverRef}> <p>{name}</p> {isHovered && <p>{details}</p>} </div> ); } export default Item;
In this case, the details about each item only appear when the item is being hovered over.
These examples illustrate how `useHover` can be employed to provide dynamic, responsive, and engaging interfaces, ultimately enhancing the user experience. As always, keep in mind that the best user interfaces are not only interactive but also intuitive and user-friendly.
Conclusion and Future Prospects
This conclusion recaps the exploration of ReactJS’s component-based design and the useHover hook, a tool that greatly simplifies the implementation of hover effects in React components. It highlights the extensive potential use cases of the useHover hook, such as triggering animations, revealing hidden information, and providing visual feedback. The useHover hook becomes even more powerful when coupled with advanced techniques, making it a valuable tool in web development.
The conclusion emphasizes that the ever-evolving field of web development continuously presents opportunities for improved user interaction and experiences. Tools like useHover allow developers to capitalize on these opportunities and push the boundaries of web development. As React continues to grow and evolve, so will the available tools and techniques, encouraging developers to continue experimenting, innovating, and building engaging web experiences.
Table of Contents
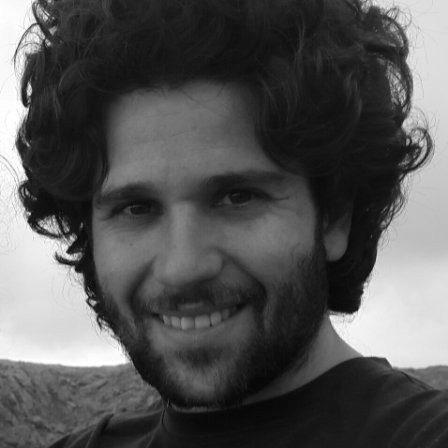
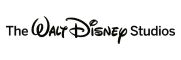