Staying in Sync with User Activity: Implementing Inactivity Detection in Components using ReactJS’s useIdle Hook
In today’s dynamic web applications, recognizing user inactivity or idle time is crucial to enhance user experience and optimize resource usage. The useIdle hook in ReactJS is a powerful tool for detecting and managing these idle periods, leading to smarter, performant, and user-friendly apps. In this blog post, we delve into the mechanics of the useIdle hook, its implementation, practical uses, and best practices for effective use in your React applications.
Understanding User Inactivity
User inactivity, in the digital context, is a period with no user interactions such as mouse movements, clicks, keystrokes, or screen touches on an application or website. Identifying such inactive periods is crucial, as it can aid security measures like automatic logout in banking apps, or pause resource-heavy processes like media playback or continuous data streaming. Not managing user inactivity can lead to negative impacts, such as overuse of network and CPU resources. Tools like the useIdle hook in ReactJS make it easier for developers to handle these scenarios, resulting in smarter, more efficient web applications with enhanced user experiences.
ReactJS Hooks: A Quick Recap
React Hooks, a feature available since React 16.8, allows developers to use state and other React features in function components without needing to write a class. Essential hooks include useState for adding state, useEffect for executing side effects, useContext for subscribing to React context, useReducer as an alternative to useState, useRef for accessing mutable ref objects, and useMemo and useCallback for performance optimization. The useIdle hook, while not built-in, applies these same principles to detect and handle user inactivity in a React application. Understanding these hooks will facilitate better utilization of tools like useIdle.
The useIdle Hook
The useIdle hook in ReactJS, typically available in libraries like react-use, effectively detects user inactivity. It monitors user events like mouse movements, clicks, keyboard inputs, and touchscreen interactions, then returns a boolean value reflecting the user’s idle or active state. The duration of inactivity required to deem a user idle is adjustable. Internally, the useIdle hook employs event listeners for various user actions, resetting an internal timer with each activity. If this timer exceeds the configured idle period without any event resetting it, the hook interprets the user as idle.
Here’s a simple example of the useIdle hook:
import { useIdle } from 'react-use'; function ExampleComponent() { const isIdle = useIdle(3000); // User is considered idle after 3 seconds return ( <div> {isIdle ? 'User is idle' : 'User is active'} </div> ); }
In this code, the useIdle hook checks if the user has been inactive for 3 seconds. Depending on the user’s activity, the component displays either ‘User is idle’ or ‘User is active’.
The useIdle hook is an incredibly useful tool for enhancing application performance and user experience by enabling more intelligent handling of user activity states. It’s a perfect example of how React hooks allow you to write clean, intuitive, and functional code.
Implementing useIdle Hook in Your React Application
The useIdle hook isn’t included by default in ReactJS, but you can find it in third-party libraries like `react-use`. Let’s see how to install this library and then use the useIdle hook in your application.
First, install the `react-use` library in your project by running this command in your terminal:
npm install react-use
Once you have installed `react-use`, you can start using the useIdle hook. Here’s a step-by-step guide on how to implement it:
Step 1: Import useIdle Hook
Import the `useIdle` hook from the `react-use` library at the top of your React component file.
import { useIdle } from 'react-use';
Step 2: Use the useIdle Hook
Inside your component, call the `useIdle` hook and pass the duration (in milliseconds) for which a user should be inactive to be considered idle.
const isIdle = useIdle(5000); // 5 seconds
Step 3: Use the Returned Value
The `useIdle` hook returns a boolean value. Use this value in your component to display different content or perform different actions based on whether the user is idle or not.
return ( <div> {isIdle ? 'User is idle' : 'User is active'} </div> );
So the whole component may look like this:
import React from 'react'; import { useIdle } from 'react-use'; function ExampleComponent() { const isIdle = useIdle(5000); // User is considered idle after 5 seconds return ( <div> {isIdle ? 'User is idle' : 'User is active'} </div> ); } export default ExampleComponent;
In this example, the `ExampleComponent` will display ‘User is idle’ if no user activity has been detected for 5 seconds. Otherwise, it will display ‘User is active’. This approach could be adapted to perform other actions, like triggering alerts, logging out, saving progress, or any other functionality that would benefit from knowing when the user is inactive.
Case Study: Detecting User Inactivity in a Sample Web App
Let’s consider a case study where the useIdle hook is applied in a real-world application. We’ll use a hypothetical news aggregator website, “ReactNews”, as our example.
ReactNews: A User-Centric News Aggregator
ReactNews provides users with a feed of news articles from various sources. To keep the feed fresh and updated, the application continually fetches new articles while the user is active.
However, constantly fetching data can be resource-intensive and unnecessary when the user is not actively interacting with the application. To optimize performance, ReactNews uses the useIdle hook to detect user inactivity and pause data fetching during idle periods.
Implementation
Firstly, `react-use` is installed in the project. The useIdle hook is then utilized within the main NewsFeed component:
import React from 'react'; import { useIdle } from 'react-use'; import { fetchNewArticles } from './api'; function NewsFeed() { const isIdle = useIdle(60000); // User is considered idle after 1 minute useEffect(() => { if (!isIdle) { const interval = setInterval(() => { fetchNewArticles(); }, 5000); // Fetch new articles every 5 seconds return () => clearInterval(interval); // Clean up interval on unmount } }, [isIdle]); // Effect depends on isIdle // Rest of the component… }
In this implementation, a new set of articles is fetched every 5 seconds, but only while the user is active. The useIdle hook is configured to consider the user as idle if there’s no activity for 60 seconds.
Impact
By using the useIdle hook, ReactNews efficiently controls its resource usage. Data fetching and updating the feed are paused when the user is idle, conserving network and CPU resources. When the user interacts with the application again, the feed resumes updating. This not only enhances performance but also improves the overall user experience by ensuring fresh content is available when the user is active.
Common Pitfalls and Best Practices when Using useIdle Hook
Using the useIdle hook can greatly enhance the efficiency of your React applications, but like any tool, there are pitfalls to be aware of and best practices to follow.
Common Pitfalls:
- Not considering different types of user interactions: Remember that users can interact with your application in various ways – via mouse movements, keyboard strokes, scrolling, and touch events on mobile devices. The useIdle hook takes care of all these events, so make sure you aren’t overriding this functionality or using additional, unnecessary event listeners.
- Overcomplicating with manual event listeners: With the useIdle hook, you don’t have to manually set up and clean up event listeners to detect user activity. Avoid the pitfall of complicating your components with manual event handlers for this purpose.
- Setting overly aggressive or lenient idle periods: If the idle time is set too short, users may be marked as idle while they’re still reading or watching content. Conversely, if the idle time is set too long, the application might keep consuming resources unnecessarily. Find a balanced value that suits your application’s needs.
Best Practices:
- Use the useIdle hook wisely: Not all applications or components need to monitor user activity. Use the useIdle hook judiciously, where it can really benefit the user experience or app performance.
- Test on different devices: Ensure your idle detection works as expected on different devices and browsers. Remember, touch events are important on mobile devices, while mouse and keyboard events might be more prominent on desktops.
- Combine useIdle with other hooks for powerful effects: Consider using the useIdle hook alongside other hooks. For example, useEffect can react to changes in the idle state, triggering different side effects based on user activity.
By being mindful of these potential pitfalls and following best practices, you can get the most out of the useIdle hook, enhancing your React applications’ efficiency and user experience.
Conclusion
The useIdle hook is an efficient tool for handling user inactivity in ReactJS applications, enhancing user experience and app performance. It is versatile, catering to simple scenarios like UI updates to complex tasks like pausing resource-heavy processes during idle times. Successful usage depends on understanding its function, recognizing potential pitfalls, and following best practices. As web applications evolve, the useIdle hook will become an increasingly vital part of the ReactJS toolkit.
Table of Contents
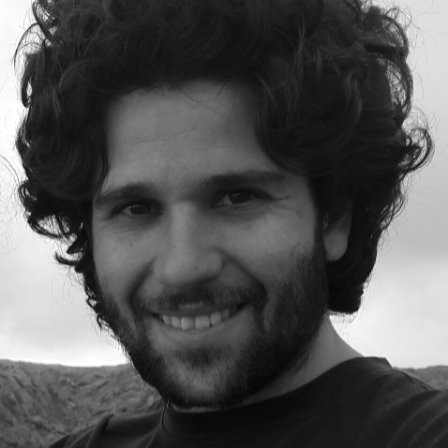
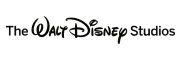