Exposing Component Functions to Parent Components in React using useImperativeHandle
-
Introduction
In the world of ReactJS, component communication plays a vital role in building complex and interactive applications. As your React application grows, you may encounter situations where a parent component needs to access specific functions or methods of its child components directly. To address this need, React provides the useImperativeHandle hook, which allows you to expose component functions to parent components. In this blog, we will explore the useImperativeHandle hook in detail, understand its basic usage and syntax, learn how to expose component functions, discuss best practices and caveats, and explore real-world examples and use cases.
1.1 Understanding the useImperativeHandle Hook
The useImperativeHandle hook is a powerful tool provided by React that enables you to control the functions exposed by a child component to its parent component. By using this hook, you can specify which functions should be accessible to the parent component. This approach helps maintain encapsulation and separation of concerns while allowing parent components to interact with specific functionalities of their child components.
1.2 Basic Usage and Syntax
To start using the useImperativeHandle hook, you need to import it from the ‘react’ package:
import { useImperativeHandle, useRef } from 'react';
The hook accepts two parameters: a ref object and a callback function. The ref object can be created using the useRef hook. The callback function defines the functions that will be exposed to the parent component.
Here’s an example that demonstrates the basic usage of useImperativeHandle:
import { useImperativeHandle, useRef } from 'react'; const ChildComponent = forwardRef((props, ref) => { const childRef = useRef(); useImperativeHandle(ref, () => ({ exposedFunction: () => { // Function logic here } })); // Rest of the component code return ( // JSX for the child component ); });
In the above example, we create a child component called ChildComponent. Inside this component, we create a childRef using the useRef hook. The useImperativeHandle hook is then used to expose the exposedFunction to the parent component. This function can be called by the parent component to interact with the child component.
1.3 Exposing Component Functions
The useImperativeHandle hook provides a straightforward way to expose component functions to the parent component. By selectively exposing only the required functions, you can maintain a clear and concise API for interaction between parent and child components.
Let’s take a closer look at how to expose component functions using the useImperativeHandle hook:
import { useImperativeHandle, useRef } from 'react'; const ChildComponent = forwardRef((props, ref) => { const childRef = useRef(); const internalFunction = () => { // Function logic here } useImperativeHandle(ref, () => ({ exposedFunction: () => { internalFunction(); } })); // Rest of the component code return ( // JSX for the child component ); });
In this example, we define an internalFunction within the child component. The useImperativeHandle hook is then used to expose the exposedFunction, which internally calls the internalFunction. By doing so, the parent component can invoke exposedFunction to indirectly trigger internalFunction within the child component.
1.4 Caveats and Best Practices
While useImperativeHandle offers great flexibility in exposing component functions, it’s important to be aware of certain caveats and follow best practices to ensure effective usage.
Limit the exposure of functions: It’s recommended to expose only the necessary functions from child components to parent components. This helps maintain encapsulation and prevents excessive coupling between components.
Avoid excessive usage: The useImperativeHandle hook should be used sparingly and only when direct access to child component functions is genuinely required. Consider alternative approaches, such as passing callback functions as props, for simpler cases.
Handle component updates: Be cautious when using useImperativeHandle in components that undergo frequent updates. When a component re-renders, the ref object passed to useImperativeHandle is replaced, potentially causing unexpected behavior. To mitigate this, consider using the useCallback hook to memoize functions that are passed to the parent component.
-
Real-World Examples and Use Cases
2.1 Form Validation
Form validation is a common use case where exposing component functions can greatly simplify the interaction between a parent component and its child form components.
import { useImperativeHandle, useRef } from 'react'; const FormInput = forwardRef((props, ref) => { const inputRef = useRef(); const validate = () => { // Validation logic here }; useImperativeHandle(ref, () => ({ validateInput: () => { validate(); } })); // Rest of the component code return ( // JSX for the form input component ); }); // Parent component const ParentComponent = () => { const formInputRef = useRef(); const handleValidation = () => { formInputRef.current.validateInput(); }; return ( <div> <FormInput ref={formInputRef} /> <button onClick={handleValidation}>Validate</button> </div> ); };
In this example, the FormInput component exposes the validateInput function to the parent component. When the parent component’s “Validate” button is clicked, it invokes the handleValidation function, which calls the validateInput function of the FormInput component. This approach allows the parent component to trigger form validation in the child component directly.
2.2 Animation Control
Another practical scenario for using useImperativeHandle is controlling animations in a parent component based on user interactions or events triggered by child components.
import { useImperativeHandle, useRef } from 'react'; const AnimationComponent = forwardRef((props, ref) => { const animationRef = useRef(); const startAnimation = () => { // Animation logic here }; const stopAnimation = () => { // Stop animation logic here }; useImperativeHandle(ref, () => ({ start: () => { startAnimation(); }, stop: () => { stopAnimation(); } })); // Rest of the component code return ( // JSX for the animation component ); }); // Parent component const ParentComponent = () => { const animationRef = useRef(); const handleStartAnimation = () => { animationRef.current.start(); }; const handleStopAnimation = () => { animationRef.current.stop(); }; return ( <div> <AnimationComponent ref={animationRef} /> <button onClick={handleStartAnimation}>Start Animation</button> <button onClick={handleStopAnimation}>Stop Animation</button> </div> ); };
In this example, the AnimationComponent exposes the start and stop functions to the parent component. The parent component’s buttons trigger the corresponding functions of the child component using the animationRef.current syntax. This allows the parent component to control the animation state of the child component.
-
Handling Component Updates and Re-renders
While using the useImperativeHandle hook, it’s important to consider how component updates and re-renders can affect the exposed component functions. By following certain guidelines, you can ensure that the exposed functions remain consistent and reliable.
3.1 Memoizing Functions with useCallback
To handle component updates and prevent unnecessary re-renders, you can utilize the useCallback hook to memoize functions that are passed to the parent component.
import { useImperativeHandle, useRef, useCallback } from 'react'; const ChildComponent = forwardRef((props, ref) => { const childRef = useRef(); const handleClick = useCallback(() => { // Function logic here }, []); useImperativeHandle(ref, () => ({ exposedFunction: handleClick })); // Rest of the component code return ( // JSX for the child component ); });
In this example, the handleClick function is memoized using useCallback with an empty dependency array. This ensures that the function remains the same across component re-renders, preventing unnecessary updates to the exposed function in the parent component.
3.2 Updating Exposed Functions with useEffect
In some cases, you may need to update the exposed functions based on certain conditions or dependencies. You can achieve this by using the useEffect hook in combination with useImperativeHandle.
import { useImperativeHandle, useRef, useEffect } from 'react'; const ChildComponent = forwardRef((props, ref) => { const childRef = useRef(); const [data, setData] = useState(null); const fetchData = useCallback(() => { // Function logic to fetch data }, []); useEffect(() => { fetchData(); }, [fetchData]); useImperativeHandle(ref, () => ({ exposedFunction: () => { // Function logic that uses the fetched data } })); // Rest of the component code return ( // JSX for the child component ); });
In this example, the fetchData function is memoized using useCallback to ensure consistent references. The useEffect hook is used to fetch the data when the component mounts or when the fetchData function changes. The useImperativeHandle hook exposes the exposedFunction, which can utilize the fetched data. By combining these hooks, you can update the exposed function whenever the necessary data or conditions change.
-
Conclusion
The useImperativeHandle hook in ReactJS provides a powerful way to expose specific component functions to parent components, enabling enhanced communication and interaction between components. By understanding the basic usage and syntax, as well as following best practices and considering potential caveats, you can effectively utilize this feature in your React applications.
We explored real-world examples, such as form validation and animation control, to showcase practical use cases of useImperativeHandle. Additionally, we discussed how to handle component updates and re-renders by memoizing functions with useCallback and updating exposed functions with useEffect.
By incorporating the useImperativeHandle hook into your React components, you can establish a clear and controlled interface between parent and child components, improving code organization and promoting reusability. Experiment with this powerful feature and leverage its capabilities to create robust and interactive React applications.
Table of Contents
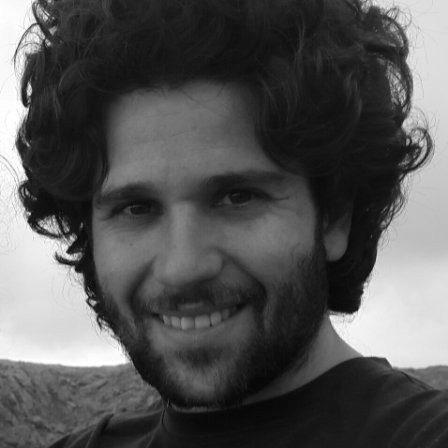
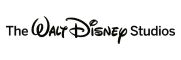