Managing setInterval in Functional Components with React JS useInterval hook
ReactJS has gained immense popularity for building dynamic and interactive web applications. With the shift towards functional components, developers have been exploring new ways to handle common tasks traditionally associated with class components. One such task is managing intervals, which is typically done using the setInterval function. In this blog post, we’ll delve into the useInterval hook, a powerful tool that simplifies and enhances the management of intervals in functional components.
1. Understanding setInterval in JavaScript
Before we dive into the useInterval hook, let’s briefly recap the setInterval function in JavaScript. This native function allows you to execute a given callback function repeatedly at a specified interval. It’s commonly used for scenarios like polling for data updates, animating elements, or creating timers. However, using setInterval in ReactJS can lead to potential issues, such as memory leaks or inconsistent component updates. This is where the useInterval hook comes into play.
1.1 Introducing the useInterval hook
React hooks revolutionized how we handle state and side effects in functional components. Custom hooks allow us to extract and reuse common logic across different components. The useInterval hook, a popular custom hook, provides a simple and efficient way to incorporate setInterval functionality into functional components.
To start using the useInterval hook, you need to import it into your project. You can either create your own custom useInterval hook or use third-party libraries like react-use or use-interval. Once imported, you can seamlessly integrate the useInterval hook into your functional components.
1.2 Implementing useInterval in functional components
Using the useInterval hook is straightforward. Let’s go through a step-by-step guide on how to integrate it into your functional components.
- Defining the interval duration: Start by specifying the duration or interval at which you want your callback function to be executed.
- Handling the interval logic: Define the callback function that will be executed at each interval. This function can include any code or state updates you need.
- Clearing the interval: Finally, ensure that you clean up the interval when the component unmounts to avoid memory leaks or unwanted side effects.
Let’s explore an example to illustrate the use of the useInterval hook:
import React, { useState } from 'react'; import useInterval from 'use-interval'; const Counter = () => { const [count, setCount] = useState(0); useInterval(() => { setCount(count + 1); }, 1000); return <div>{count}</div>; }; export default Counter;
In this example, we’re using the useInterval hook to increment the count state variable by 1 every second. As a result, the component renders and displays the updated count value on the screen.
1.3 Managing state with useInterval
One of the key advantages of using the useInterval hook is the seamless integration with React’s state management. You can easily update state variables within the interval callback function, enabling dynamic and reactive behavior in your components. This allows you to build real-time features like live updates, progress bars, or countdown timers with ease.
import React, { useState } from 'react'; import useInterval from 'use-interval'; const Timer = () => { const [seconds, setSeconds] = useState(0); useInterval(() => { setSeconds(seconds + 1); }, 1000); return <div>{seconds} seconds elapsed.</div>; }; export default Timer;
In this example, the useInterval hook increments the seconds state variable every second, providing a live timer that updates in real-time.
1.4 Handling cleanup with useInterval
Proper cleanup is crucial in React to prevent memory leaks and unexpected behavior. The useInterval hook takes care of clearing the interval automatically when the component unmounts. This ensures that no unnecessary processing or updates occur after the component is no longer rendered.
import React, { useState } from 'react'; import useInterval from 'use-interval'; const ComponentWithCleanup = () => { const [value, setValue] = useState(0); useInterval(() => { setValue(value + 1); }, 1000); return <div>{value}</div>; }; export default ComponentWithCleanup;
In this example, the useInterval hook automatically clears the interval when the component is unmounted, preventing any potential issues.
1.5 Advanced use cases and considerations
The useInterval hook provides advanced features and considerations to handle various scenarios. Here are a few examples:
- Pausing and resuming the interval: You can implement logic to pause or resume the interval based on user interactions or specific conditions.
- Conditional usage of the interval: You can conditionally enable or disable the interval based on specific criteria or user actions.
- Performance considerations with useInterval: Be mindful of the performance impact of frequent interval updates and consider optimization techniques like debouncing or throttling.
2. Step-by-Step Guide to Using the useInterval Hook
The useInterval hook provides a straightforward way to incorporate interval functionality into your functional components. Let’s explore each step in detail:
Step 1: Defining the interval duration
The first step is to specify the duration or interval at which you want your callback function to be executed. This duration is specified in milliseconds.
useInterval(callback, delay);
In the useInterval hook, the callback parameter represents the function that will be executed at each interval, and the delay parameter represents the interval duration.
Step 2: Handling the interval logic
In this step, you define the callback function that will be executed at each interval. This function can include any code or state updates you need. It will run repeatedly based on the specified interval duration.
useInterval(() => { // Interval logic here }, delay);
Inside the callback function, you can perform tasks such as updating state variables, making API calls, manipulating the DOM, or any other necessary operations.
Let’s consider an example where we use the useInterval hook to update a count state variable every second:
import React, { useState } from 'react'; import useInterval from 'use-interval'; const Counter = () => { const [count, setCount] = useState(0); useInterval(() => { setCount(count + 1); }, 1000); return <div>{count}</div>; }; export default Counter;
In this example, the Counter component renders a <div> element that displays the updated count value. The useInterval hook increments the count state variable by 1 every second. As a result, the component renders and displays the updated count value on the screen.
Step 3: Clearing the interval
To avoid memory leaks and unwanted side effects, it’s crucial to clean up the interval when the component unmounts. Fortunately, the useInterval hook takes care of this automatically.
useInterval(() => { // Interval logic here }, delay);
By using the useInterval hook, the interval is cleared when the component is unmounted, ensuring proper cleanup.
Let’s consider an example where we use the useInterval hook and ensure the interval is cleared on component unmount:
import React, { useState } from 'react'; import useInterval from 'use-interval'; const ComponentWithCleanup = () => { const [value, setValue] = useState(0); useInterval(() => { setValue(value + 1); }, 1000); return <div>{value}</div>; }; export default ComponentWithCleanup;
In this example, the ComponentWithCleanup component renders a <div> element that displays the updated value. The useInterval hook increments the value state variable by 1 every second. The interval is automatically cleared when the component unmounts, preventing any potential issues.
By following this step-by-step guide, you can effectively use the useInterval hook to manage intervals in your functional components. Remember to define the interval duration, handle the interval logic inside the callback function, and let the hook handle the cleanup by clearing the interval on component unmount.
4. Comparing useInterval with setInterval in Class Components
Traditionally, class components in React have been used to handle complex state and side effects. When it comes to managing intervals, the setInterval function is commonly utilized. However, using setInterval in class components can introduce certain challenges and complexities. The useInterval hook, on the other hand, provides a more straightforward and efficient approach to interval management in functional components. Let’s explore the key differences and advantages of using the useInterval hook over setInterval in class components.
4.1 Ease of Use and Code Readability:
In class components, using setInterval involves manually setting up and clearing the interval. The interval is typically set up in the componentDidMount lifecycle method and cleared in the componentWillUnmount method. This can lead to scattered code and increased complexity.
With the useInterval hook, the interval setup and cleanup are abstracted away, making the code more concise and readable. The hook automatically handles the interval setup when the component mounts and clears it when the component unmounts. This simplifies the code structure and improves overall code maintainability.
4.2 Integration with Functional Components:
The useInterval hook is designed specifically for functional components, which have become the preferred approach in React. It seamlessly integrates with the functional component paradigm, allowing you to incorporate interval functionality without the need for class components.
In contrast, setInterval is primarily used in class components, and integrating it with functional components requires additional workarounds or boilerplate code.
4.3 State Management:
Managing state within the interval callback is crucial for building dynamic and interactive applications. The useInterval hook integrates seamlessly with React’s state management, allowing you to update state variables directly within the interval callback function. This enables real-time updates and dynamic behavior in your components.
In class components, managing state within setInterval requires accessing the this.setState method, which can be cumbersome and less intuitive compared to functional components with hooks.
4.4 Cleanup and Memory Leaks
Proper cleanup is essential to prevent memory leaks and unwanted side effects. The useInterval hook takes care of automatically clearing the interval when the component unmounts. This ensures that no unnecessary processing or updates occur after the component is no longer rendered.
In class components, manually clearing the interval in componentWillUnmount is required to avoid memory leaks. Forgetting to clear the interval can result in potential issues, such as continuous function executions even after the component is unmounted.
4.5 Code Reusability and Customization
Custom hooks, including the useInterval hook, promo code reusability and abstraction. You can easily extract the useInterval logic into a separate custom hook and reuse it across multiple functional components. This improves code modularity and reduces duplication.
In class components, interval setup and cleanup code need to be duplicated or handled through higher-order components (HOCs) or render props, which can be more cumbersome and less intuitive.
Overall, the useInterval hook offers a more intuitive, concise, and efficient way to manage intervals in functional components compared to using setInterval in class components. It simplifies the code structure, integrates seamlessly with state management, automates cleanup, and promotes code reusability. As functional components and hooks become the recommended approach in React, the useInterval hook aligns perfectly with the modern React ecosystem.
5. Conclusion
The useInterval hook is a valuable tool in the ReactJS ecosystem, especially for managing intervals in functional components. It simplifies the implementation of intervals, enables seamless state updates, and handles cleanup automatically. By incorporating the useInterval hook into your projects, you can ensure efficient and reliable interval management while harnessing the power of functional components in ReactJS. Experiment with the useInterval hook and explore its possibilities to enhance your React development experience.
Table of Contents
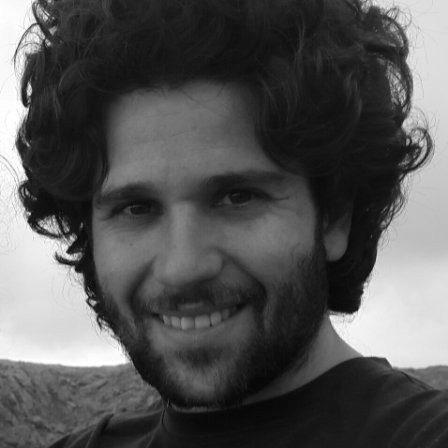
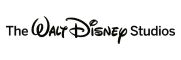