Handling keyboard events in ReactJS componants with useKeyPress Hooks
Keyboard events play a vital role in web development, enhancing user interaction across various applications, from form inputs to interactive games. Managing these events can be challenging, but ReactJS, a widely-used JavaScript library, makes this task easier with its useKeyPress Hook. This Hook, part of React’s Hooks system, allows for efficient handling of keyboard events within functional components.
This blog post will provide a comprehensive guide to using the useKeyPress Hook, comparing traditional JavaScript methods to ReactJS, explaining the setup, exploring practical applications, and discussing best practices to elevate your React applications.
Understanding Keyboard Events in JavaScript
Keyboard events, triggered when users interact with the keyboard, are vital for creating responsive and accessible web applications. These events allow developers to effectively manage user inputs.
JavaScript mainly categorizes keyboard events into three types:
- `keydown`: Triggered when any key, including function and special keys, is pressed down.
- `keyup`: Fires when any key is released.
- `keypress`: Activated only for keys that produce a character value and for each character insertion when the key is held down.
Each keyboard event offers an event object containing information about the triggering key, including its value, code, and the state of modifier keys. Properly handling these keyboard events enables developers to create more interactive and user-friendly websites and applications, from simple tasks like form submission to complex tasks like implementing keyboard shortcuts or game controls.
Handling Keyboard Events in JavaScript vs ReactJS
Handling keyboard events in JavaScript and ReactJS are similar to some extent because ReactJS is a JavaScript library after all. However, React adds an extra layer of abstraction to make the process more efficient and intuitive.
In JavaScript:
Handling keyboard events in vanilla JavaScript typically involves attaching event listeners to DOM elements. Here’s a simple example of how you might handle a keyboard event:
document.addEventListener('keydown', function(event) { console.log(`You pressed ${event.key}`); });
In this case, a function is attached as an event listener to the ‘keydown’ event on the entire document. Whenever a key is pressed, the function logs the pressed key to the console.
In ReactJS:
In contrast, ReactJS uses a system called Synthetic Events, which wraps around the browser’s native events to provide consistency across different browsers. Instead of attaching event listeners to DOM elements directly, you define event handlers as component methods. Here’s how you might handle a keyboard event in a React component:
class ExampleComponent extends React.Component { handleKeyDown(event) { console.log(`You pressed ${event.key}`); } render() { return <div onKeyDown={this.handleKeyDown}>Press a key</div>; } }
ReactJS offers several advantages for keyboard event handling:
- Consistency: React’s Synthetic Event system ensures uniformity in event handling across different browsers.
- Performance: React enhances performance by using event delegation, attaching a single event listener to the document root rather than individual nodes, thus saving memory.
- Integration with React’s State: React facilitates more interactive and responsive UIs by allowing event handling functions to connect with the component’s state.
- Ease of Use: By defining event handlers as component methods, React simplifies the management of complex event-related logic.
Introduction to the useKeyPress Hook in ReactJS
React Hooks, introduced in React 16.8, are functions that let you use state and other React features without writing a class. Among these hooks, there is a useful, but unofficial, custom hook called useKeyPress. This hook provides a more straightforward and intuitive way to handle keyboard events within functional components.
The useKeyPress hook is not part of React’s standard library, meaning it doesn’t come bundled with React. Instead, it’s a pattern that developers have created by combining some of the built-in hooks like `useState` and `useEffect`.
Here’s a simple example of what the useKeyPress hook might look like:
import { useState, useEffect } from 'react'; function useKeyPress(targetKey) { const [keyPressed, setKeyPressed] = useState(false); function downHandler({ key }) { if (key === targetKey) { setKeyPressed(true); } } const upHandler = ({ key }) => { if (key === targetKey) { setKeyPressed(false); } }; useEffect(() => { window.addEventListener('keydown', downHandler); window.addEventListener('keyup', upHandler); return () => { window.removeEventListener('keydown', downHandler); window.removeEventListener('keyup', upHandler); }; }, []); return keyPressed; }
In the code above, the `useKeyPress` hook is defining what happens when a specific key is pressed (`keydown`) and released (`keyup`). When you press the target key, it sets `keyPressed` to true, and when you release it, it sets `keyPressed` to false. The `useEffect` hook is used to add and clean up the event listeners.
With useKeyPress, you can create more interactive components in a clean and understandable way. In the upcoming sections, we’ll dive deeper into implementing this hook in a React component and explore its practical use cases.
A step-by-step guide to using useKeyPress Hook in ReactJS
Certainly, here’s a simplified step-by-step guide on how to use the `useKeyPress` hook in a ReactJS application:
- Initial Setup: Make sure your project is set up to work with React and that you’re using a version that supports Hooks (16.8 and above).
- Define the useKeyPress Hook: Here is a simple version of the `useKeyPress` hook:
import { useState, useEffect } from 'react'; function useKeyPress(targetKey) { const [keyPressed, setKeyPressed] = useState(false); function downHandler({ key }) { if (key === targetKey) { setKeyPressed(true); } } const upHandler = ({ key }) => { if (key === targetKey) { setKeyPressed(false); } }; useEffect(() => { window.addEventListener('keydown', downHandler); window.addEventListener('keyup', upHandler); return () => { window.removeEventListener('keydown', downHandler); window.removeEventListener('keyup', upHandler); }; }, []); return keyPressed; }
3. Implement the Hook in a Component: Now that you’ve defined the `useKeyPress` hook, you can use it in a functional component. For example:
import React from 'react'; import useKeyPress from './useKeyPress'; // Import the hook function ExampleComponent() { const keyPressed = useKeyPress('a'); return ( <div> {keyPressed ? 'You pressed the "a" key!' : 'Press the "a" key'} </div> ); } export default ExampleComponent;
In the `ExampleComponent` above, the `useKeyPress` hook is invoked with ‘a’ as the target key. When the ‘a’ key is pressed, `keyPressed` will be true, and the component will render ‘You pressed the “a” key!’. When the ‘a’ key is not being pressed, `keyPressed` will be false, and the component will render ‘Press the “a” key’.
Please note that these are simplified examples. In a real-world application, you might need more complex logic and additional error handling. But this should give you a solid starting point for using the `useKeyPress` hook in React.
Advanced applications of useKeyPress Hook
The useKeyPress hook in React provides a way to add complex interactivity to your components based on keyboard inputs. Here are some advanced applications:
- Keyboard Shortcuts: If your web application uses keyboard shortcuts, useKeyPress can be a convenient way to manage them. By assigning different hooks to different keys, you can trigger different functions based on the key pressed.
Example: Let’s say you want to add a shortcut to save a form when the ‘s’ key is pressed:
const savePressed = useKeyPress('s'); useEffect(() => { if (savePressed) { // Function to save the form saveForm(); } }, [savePressed]);
- Navigation through Keyboard Arrows: You can use useKeyPress to navigate a list or move an object in a game using the arrow keys.
Example: Let’s say you have a list of items, and you want to navigate through them using the arrow keys:
const downPress = useKeyPress('ArrowDown'); const upPress = useKeyPress('ArrowUp'); const [cursor, setCursor] = useState(0); useEffect(() => { if (downPress) { setCursor(prevCursor => prevCursor < items.length - 1 ? prevCursor + 1 : prevCursor); } }, [downPress]); useEffect(() => { if (upPress) { setCursor(prevCursor => prevCursor > 0 ? prevCursor - 1 : prevCursor); } }, [upPress]);
3. Handling Multiple Keypresses: The useKeyPress hook can also help in handling complex actions involving multiple keys pressed simultaneously. For example, in a game, you might allow a user to move faster by holding down the shift key while pressing a direction key.
const shiftPress = useKeyPress('Shift'); const leftPress = useKeyPress('ArrowLeft'); useEffect(() => { if (shiftPress && leftPress) { // Function to move faster to the left fastMoveLeft(); } else if (leftPress) { // Function to move to the left moveLeft(); } }, [shiftPress, leftPress]);
These are just a few examples of the possibilities opened up by the useKeyPress hook. By handling keyboard inputs effectively, you can create a more interactive and user-friendly experience on your website or application.
Best Practices when Using the useKeyPress Hook
When using the `useKeyPress` hook in ReactJS, adhere to these key best practices for an efficient and user-friendly application:
- Event Listener Clean-up: Remove event listeners when components unmount to prevent memory leaks.
- Accessibility: Ensure your application is usable without keyboard input, treating keyboard inputs as enhancements.
- Shortcut Conflicts: Avoid creating shortcuts that conflict with common browser and operating system shortcuts.
- Throttling and Debouncing: Limit frequency of heavy function calls triggered by keyboard events for improved performance.
- Cross-Browser Testing: Keyboard events can vary across browsers and devices, so comprehensive testing is essential.
- Visual Feedback: Offer visual cues to users when keyboard inputs trigger actions on the page.
- Documentation: Document your keyboard interactions clearly and make them easy for users to discover.
Following these guidelines while using the `useKeyPress` hook can optimize your application and enhance user experience.
Conclusion
Handling keyboard events is a critical part of interactive web applications, which becomes simpler and more efficient with the `useKeyPress` hook in ReactJS. This hook streamlines keyboard interactions in functional components, enhancing user experience.
We’ve explored how `useKeyPress` outperforms traditional JavaScript methods, its setup in a React component, advanced applications, and best practices. However, it’s essential to remember that `useKeyPress` is one of many tools available. Choose those that best suit your application and audience, and remember the importance of testing and iteration in developing a user-friendly web application.
Whether you’re an experienced React developer or a beginner, we hope this guide has deepened your understanding of `useKeyPress` and its role in improving React applications.
Happy coding!
Table of Contents
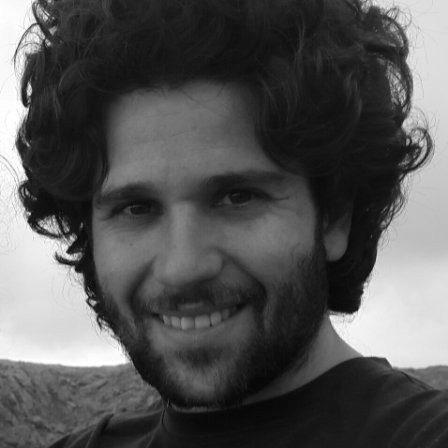
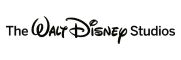