Syncing ReactJS State with Local Storage using the useLocalStorage Hook
ReactJS has become one of the most popular JavaScript libraries for building modern user interfaces. When developing React applications, managing state is a crucial aspect. One common requirement is to persist state data even when the user refreshes the page or closes the browser. This is where local storage comes in handy.
Introduction to ReactJS and Local Storage
What is ReactJS?
ReactJS is a JavaScript library for building user interfaces. It allows developers to create reusable UI components that efficiently update and render in response to changes in application state. React follows a component-based architecture, making it easy to build complex web applications.
Understanding Local Storage
Local storage is a web API provided by modern browsers that allows data to be stored locally on the user’s device. It provides a simple key-value storage mechanism, where data is persisted even after the browser is closed or the page is refreshed. Local storage is widely supported and offers a convenient way to store small amounts of data.
Using the useLocalStorage Hook
React provides hooks as a way to reuse stateful logic across components. The useLocalStorage hook is a custom hook that combines the functionality of React’s useState hook with local storage, allowing state to be synced and persisted.
Exploring the useLocalStorage Hook
The useLocalStorage hook is designed to simplify the process of syncing state with local storage. It takes an initial value and a key as parameters and returns an array with the current value and a setter function. The hook internally handles reading from and writing to local storage.
import { useState, useEffect } from 'react'; function useLocalStorage(key, initialValue) { const [value, setValue] = useState(() => { const storedValue = localStorage.getItem(key); return storedValue !== null ? JSON.parse(storedValue) : initialValue; }); useEffect(() => { localStorage.setItem(key, JSON.stringify(value)); }, [key, value]); return [value, setValue]; }
How to Implement the useLocalStorage Hook
To implement the useLocalStorage hook in your React component, you can follow these steps:
Import the useLocalStorage hook from the appropriate file.
Declare a state variable using the hook and provide a key and an initial value.
Use the state variable and the setter function in your component as needed.
import React from 'react'; import useLocalStorage from './useLocalStorage'; function App() { const [name, setName] = useLocalStorage('name', ''); const handleChange = (event) => { setName(event.target.value); }; return ( <div> <input type="text" value={name} onChange={handleChange} /> <p>Hello, {name}!</p> </div> ); } export default App;
This is just a basic example, but you can use the useLocalStorage hook to sync and persist any kind of state in your React application.Advantages and Use Cases of Syncing State with Local Storage
Syncing state with local storage using the useLocalStorage hook offers several advantages in React applications. Let’s explore some of these benefits and use cases.
Advantages of Syncing State with Local Storage
Persistent Data: By syncing state with local storage, you ensure that the data remains available even after a page refresh or browser restart. This persistence allows users to have a seamless experience without losing their data.
Shared State: Local storage allows for sharing state data across multiple instances of the same application. Users can open the application in different browser tabs or windows and still have access to the same data.
User Preferences: Storing user preferences, such as theme settings, language preferences, or user-specific configurations, becomes easier with local storage. Users can customize their experience and have their preferences remembered across sessions.
Use Cases for Syncing State with Local Storage
Form Persistence: When dealing with forms, syncing state with local storage can be beneficial. For example, consider a multi-step form where users might need to navigate away or refresh the page. With local storage, the form data can be automatically restored, allowing users to pick up where they left off.
User Authentication: Syncing user authentication state with local storage ensures that users remain authenticated even if they close and reopen the application. This eliminates the need for users to log in repeatedly.
Now, let’s dive deeper into some code examples to illustrate how the useLocalStorage hook can be used in different scenarios.
Example 1: Storing User Preferences
Imagine an application that allows users to customize their theme. We can utilize the useLocalStorage hook to store and sync the chosen theme preference.
import React from 'react'; import useLocalStorage from './useLocalStorage'; function ThemeSelector() { const [theme, setTheme] = useLocalStorage('theme', 'light'); const handleThemeChange = (event) => { setTheme(event.target.value); }; return ( <div> <label htmlFor="theme-select">Select Theme:</label> <select id="theme-select" value={theme} onChange={handleThemeChange}> <option value="light">Light</option> <option value="dark">Dark</option> <option value="blue">Blue</option> </select> </div> ); } export default ThemeSelector;
In this example, the useLocalStorage hook is used to sync the theme state with the local storage, ensuring that the selected theme is persisted.
Example 2: Remembering Form Data
Consider a scenario where users are filling out a complex form with multiple fields. To enhance the user experience, we can utilize the useLocalStorage hook to remember and restore their form input.
import React from 'react'; import useLocalStorage from './useLocalStorage'; function Form() { const [formData, setFormData] = useLocalStorage('form-data', { name: '', email: '', message: '', }); const handleChange = (event) => { setFormData({ ...formData, [event.target.name]: event.target.value, }); }; return ( <form> <label htmlFor="name-input">Name:</label> <input type="text" id="name-input" name="name" value={formData.name} onChange={handleChange} /> <label htmlFor="email-input">Email:</label> <input type="email" id="email-input" name="email" value={formData.email} onChange={handleChange} /> <label htmlFor="message-textarea">Message:</label> <textarea id="message-textarea" name="message" value={formData.message} onChange={handleChange} ></textarea> </form> ); } export default Form;
In this example, the useLocalStorage hook is used to sync the formData state with the local storage. This ensures that the user’s input is preserved, even if they navigate away from the page or refresh it.
Best Practices and Considerations
When using the useLocalStorage hook to sync state with local storage in React applications, it’s essential to keep certain best practices and considerations in mind. Let’s explore some of them:
Best Practices for Using useLocalStorage
Serialize and Deserialize Data: Local storage can only store strings, so it’s important to serialize complex data structures into strings before storing them. Conversely, when retrieving data from local storage, deserialize the stored string back into the original data structure. JSON.stringify and JSON.parse can be used for serialization and deserialization, respectively.
Fallback Values: It’s a good practice to provide fallback values when reading from local storage. If the stored value doesn’t exist or encounters any errors during deserialization, having a fallback value ensures that your application can handle such scenarios gracefully.
Clearing Stale Data: Consider implementing a mechanism to clear stale data from local storage. If the structure or format of the stored data changes over time, you might encounter compatibility issues. By periodically clearing or updating the stored data, you can ensure a smooth experience for your users.
Considerations and Limitations
Security: Keep in mind that local storage is accessible by JavaScript running in the same domain. Avoid storing sensitive or confidential data in local storage, as it can be vulnerable to cross-site scripting (XSS) attacks.
Storage Limitations: Local storage has a limited capacity (usually around 5-10MB) depending on the browser. Be cautious when storing large amounts of data, as it can impact the performance of your application.
Browser Support: Local storage is supported by modern browsers, but it’s always a good idea to verify the compatibility with older versions or specific browsers if your target audience requires it.
Now that we’ve covered some best practices and considerations, let’s explore a few more code examples to illustrate how the useLocalStorage hook can be used in different scenarios.
Example 3: Remembering Collapsible State
In some cases, you might have collapsible sections or accordions in your application. You can use the useLocalStorage hook to remember the state of the collapsible sections and restore them when the user revisits the page.
import React from 'react'; import useLocalStorage from './useLocalStorage'; function CollapsibleSection({ title, content }) { const [isCollapsed, setCollapsed] = useLocalStorage( `collapsible_${title}`, false ); const toggleCollapse = () => { setCollapsed(!isCollapsed); }; return ( <div> <h3 onClick={toggleCollapse}>{title}</h3> {!isCollapsed && <p>{content}</p>} </div> ); } export default CollapsibleSection;
In this example, each collapsible section has a unique key based on the title prop. This ensures that the state of each section is stored separately in local storage.
Example 4: Remembering Tab Selection
Tabbed interfaces are a common UI pattern. You can use the useLocalStorage hook to remember the selected tab and restore it when the user returns to the page.
import React from 'react'; import useLocalStorage from './useLocalStorage'; function TabbedInterface({ tabs }) { const [selectedTab, setSelectedTab] = useLocalStorage('selected_tab', ''); const handleTabClick = (tab) => { setSelectedTab(tab); }; return ( <div> <ul> {tabs.map((tab) => ( <li key={tab} className={selectedTab === tab ? 'active' : ''} onClick={() => handleTabClick(tab)} > {tab} </li> ))} </ul> <div> {/* Render the content based on the selectedTab */} </div> </div> ); } export default TabbedInterface;
In this example, the selectedTab state is synced with local storage. When the user selects a tab, the selected tab is stored in local storage, allowing the application to remember the selected tab even after a page reload.
Troubleshooting and Common Issues
While using the useLocalStorage hook to sync state with local storage in React applications, you might encounter some common issues. Let’s explore how to troubleshoot these issues and handle them effectively.
Debugging Local Storage Syncing Problems
Check Key Names: Ensure that the key used for storing and retrieving data from local storage is consistent across your application. Mismatched key names can lead to unexpected behavior or data not being synced properly.
Inspect Local Storage: Use browser developer tools to inspect the local storage and verify if the data is being stored correctly. Check the key-value pairs and their values to ensure they align with your expectations.
Clear Local Storage: If you encounter issues with stale or conflicting data in local storage, clearing the local storage entirely or selectively can help resolve those issues. Be cautious when using this approach, as it will remove all data stored in the local storage for the given domain.
Handling Edge Cases and Data Validation
Invalid Data: When reading from local storage, handle cases where the stored data might be invalid or corrupted. Perform data validation and consider fallback values or error handling to prevent unexpected crashes or application behavior.
Data Structure Changes: If you modify the structure or format of the stored data in your application, ensure backward compatibility. Consider implementing versioning or migration logic to handle scenarios where the structure of the stored data needs to be updated.
Now, let’s explore alternative approaches to syncing state with local storage in React applications.
Alternatives to useLocalStorage
While the useLocalStorage hook provides a convenient way to sync state with local storage, there are alternative libraries and approaches you can consider based on your project’s requirements.
Redux and Redux Persist: If your application uses Redux for state management, you can leverage Redux Persist. It provides an integration with local storage, allowing you to persist and rehydrate the Redux store.
Custom Storage Solutions: Depending on your specific needs, you might consider implementing custom storage solutions using IndexedDB or other client-side storage options. This allows for more fine-grained control over data persistence and can handle larger amounts of data.
Now that we’ve explored troubleshooting, alternative approaches, and best practices, let’s summarize the benefits and considerations of syncing state with local storage using the useLocalStorage hook.
Recap of Benefits and Considerations
Syncing state with local storage using the useLocalStorage hook offers several benefits:
Persistence: Data remains available even after a page refresh or browser restart.
Shared State: State can be shared across multiple instances of the same application.
User Preferences: User settings and preferences can be remembered across sessions.
However, there are some considerations and limitations to keep in mind:
Security: Avoid storing sensitive or confidential data in local storage.
Storage Limitations: Local storage has a limited capacity, so be mindful of storing large amounts of data.
Browser Support: Verify compatibility with older browsers or specific browser versions, if necessary.
Examples and Code Implementation
To further illustrate the practical implementation of the useLocalStorage hook in React applications, let’s explore a couple of examples that demonstrate its usage in different scenarios.
Example 1: To-Do List with Local Storage
A common use case for syncing state with local storage is managing a to-do list. Let’s create a simple to-do list application that persists the to-do items using the useLocalStorage hook.
import React, { useState } from 'react'; import useLocalStorage from './useLocalStorage'; function TodoApp() { const [todos, setTodos] = useLocalStorage('todos', []); const addTodo = (text) => { const newTodo = { id: Date.now(), text }; setTodos([...todos, newTodo]); }; const removeTodo = (id) => { const updatedTodos = todos.filter((todo) => todo.id !== id); setTodos(updatedTodos); }; return ( <div> <h1>Todo App</h1> <TodoForm addTodo={addTodo} /> <TodoList todos={todos} removeTodo={removeTodo} /> </div> ); } function TodoForm({ addTodo }) { const [text, setText] = useState(''); const handleSubmit = (e) => { e.preventDefault(); if (text.trim()) { addTodo(text); setText(''); } }; return ( <form onSubmit={handleSubmit}> <input type="text" value={text} onChange={(e) => setText(e.target.value)} /> <button type="submit">Add Todo</button> </form> ); } function TodoList({ todos, removeTodo }) { return ( <ul> {todos.map((todo) => ( <li key={todo.id}> {todo.text} <button onClick={() => removeTodo(todo.id)}>Delete</button> </li> ))} </ul> ); } export default TodoApp;
In this example, the todos state is synced with local storage using the useLocalStorage hook. Each time a new to-do item is added or removed, the state is updated, and the changes are automatically persisted in the local storage.
Example 2: Dark Mode Toggle
Implementing a dark mode feature is another practical scenario where syncing state with local storage is beneficial. Let’s create a toggle button that switches between light and dark modes and remembers the user’s preference using the useLocalStorage hook.
import React from 'react'; import useLocalStorage from './useLocalStorage'; function DarkModeToggle() { const [isDarkMode, setIsDarkMode] = useLocalStorage('darkMode', false); const handleToggle = () => { setIsDarkMode(!isDarkMode); }; return ( <div> <button onClick={handleToggle}> {isDarkMode ? 'Light Mode' : 'Dark Mode'} </button> <div className={isDarkMode ? 'dark-mode' : 'light-mode'}> {/* Rest of the application */} </div> </div> ); } export default DarkModeToggle;
In this example, the isDarkMode state is synced with local storage. When the user toggles between light and dark mode, the state is updated, and the changes are stored in the local storage. Upon subsequent visits, the application remembers the user’s chosen mode and applies it accordingly.
Conclusion
In this blog post, we explored the concept of syncing state with local storage in React applications using the useLocalStorage hook. We discussed the benefits of persisting state data, such as maintaining data across page refreshes and providing a seamless user experience.
We started by understanding ReactJS as a powerful JavaScript library for building user interfaces. We also learned about local storage, a web API that allows us to store data on the user’s device.
The useLocalStorage hook proved to be a valuable tool for syncing React state with local storage. It simplified the process of reading from and writing to local storage, enabling us to create resilient applications.
Throughout the blog post, we covered various aspects related to the useLocalStorage hook. We discussed best practices, such as serializing and deserializing data, providing fallback values, and handling edge cases. We also explored common troubleshooting techniques and considered important security and browser compatibility considerations.
To solidify our understanding, we provided code examples that showcased the usage of the useLocalStorage hook in different scenarios. We built a to-do list application that stored tasks persistently, and we implemented a dark mode toggle that remembered the user’s preference.
Remember, the examples we provided were just a starting point, and you can further customize and expand upon them based on your specific application requirements.
By utilizing the useLocalStorage hook, React developers can enhance their applications by enabling state persistence and providing a better user experience. It offers flexibility and simplicity, allowing developers to focus on building robust and interactive interfaces.
As you continue to explore ReactJS and local storage, consider how the useLocalStorage hook can benefit your projects. Experiment with different use cases and explore additional ways to synchronize state with local storage to elevate your applications.
Thank you for joining us on this journey to understand the useLocalStorage hook and its usage in ReactJS applications. Happy coding!
Table of Contents
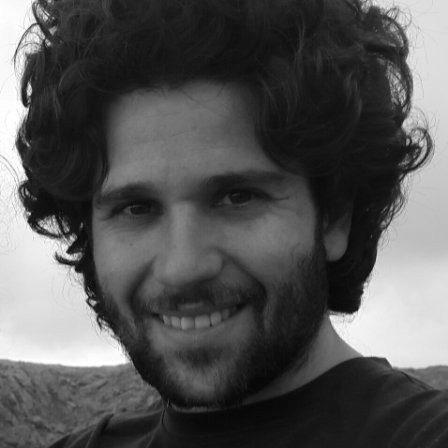
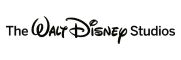