Creating Responsive Components Based on Screen Size with ReactJS and useMediaQuery
Welcome to the world of responsive web development with ReactJS! In this blog post, we will explore the powerful capabilities of the ReactJS useMediaQuery hook for building responsive components based on screen size. In today’s digital landscape, where users access websites and applications from a wide range of devices, it’s crucial to ensure a seamless and optimized experience across various screen sizes.
Responsive design allows us to create user interfaces that adapt and adjust dynamically to different screen resolutions, ensuring that our content is easily accessible and visually appealing on smartphones, tablets, and desktop computers alike. With ReactJS, a popular JavaScript library for building user interfaces, we can leverage the useMediaQuery hook to achieve responsive behavior in our components effortlessly.
To get started, let’s briefly delve into what responsive design is and why it’s essential in modern web development.
1. What is Responsive Design?
Responsive design is an approach to web development that aims to create user interfaces that adapt and respond to different screen sizes and devices. The goal is to provide an optimal viewing experience, ensuring that users can easily navigate and interact with the content regardless of the device they are using.
One of the key principles of responsive design is fluidity. Instead of designing fixed-width layouts, responsive design uses flexible grids and elements that can adjust their size and position based on the screen’s dimensions. This flexibility allows the content to rearrange itself dynamically, optimizing the layout for the available space.
Media queries play a crucial role in responsive design. They are CSS rules that apply specific styles based on the characteristics of the device or screen size. By using media queries, we can selectively modify the appearance and behavior of our components to accommodate different devices, such as smartphones, tablets, and desktops.
In ReactJS, the useMediaQuery hook provides a convenient way to implement responsive behavior in our components. This hook allows us to dynamically detect the screen size and conditionally render different content or apply specific styles based on the media query rules we define.
Let’s explore how we can utilize the useMediaQuery hook to create responsive components in ReactJS. But before that, let’s briefly understand the basics of ReactJS and the useMediaQuery hook itself.
Code sample (Media query example in CSS):
/* Media query example */ @media screen and (max-width: 768px) { /* Styles applied for screens with a maximum width of 768 pixels */ .responsive-element { font-size: 16px; padding: 10px; } }
In the above code snippet, the styles within the media query will be applied only when the screen width is 768 pixels or less. This allows us to customize the appearance of elements specifically for smaller screens, improving the overall user experience.
Now that we have a basic understanding of responsive design and media queries, let’s dive deeper into ReactJS and the useMediaQuery hook to build responsive components effectively.
2. Importance of Responsive Components
In today’s mobile-first era, where users access websites and applications on a wide range of devices, building responsive components has become more critical than ever. Responsive components play a pivotal role in delivering a seamless user experience across different screen sizes, ensuring that your content is accessible and visually appealing on any device.
Here are a few reasons highlighting the importance of responsive components in your ReactJS applications:
Enhanced User Experience: Responsive components adapt to the screen size, providing an optimal viewing experience for users. By adjusting the layout, font sizes, and spacing dynamically, you can ensure that your content remains readable and visually appealing, regardless of the device used.
Increased Reach: With the proliferation of smartphones and tablets, it’s crucial to cater to users on various devices. By building responsive components, you can reach a broader audience and provide a consistent experience across different platforms, boosting user engagement and satisfaction.
Improved Performance: Responsive components allow you to optimize the loading and rendering of content based on the device’s capabilities. By selectively loading or hiding certain elements, images, or scripts, you can reduce bandwidth usage and enhance performance, resulting in faster page load times.
Future-Proofing: As new devices and screen sizes emerge, responsive components provide a flexible foundation for adapting to future technological advancements. By designing components that can adjust to different breakpoints, you can future-proof your applications and ensure they remain compatible with upcoming devices.
Now that we understand the importance of responsive components, let’s explore how we can implement them using the useMediaQuery hook in ReactJS.
3. Understanding ReactJS useMediaQuery
Before we delve into implementing responsive components with ReactJS, let’s gain a better understanding of the useMediaQuery hook. The useMediaQuery hook is a built-in hook provided by the ReactJS library that enables us to conditionally render components or apply specific styles based on media query rules.
To use the useMediaQuery hook, we need to import it from the ReactJS library:
import { useMediaQuery } from 'react-responsive';
The useMediaQuery hook accepts a media query string as its parameter. This string follows the same syntax as media queries in CSS. We can define the media query rules based on different screen sizes or other characteristics of the device.
Here’s an example of using the useMediaQuery hook to detect a specific screen width:
const MyComponent = () => { const isMobile = useMediaQuery('(max-width: 768px)'); return ( <div> {isMobile ? ( <p>This is a mobile view</p> ) : ( <p>This is a desktop view</p> )} </div> ); };
In the above code snippet, we define a variable isMobile using the useMediaQuery hook with the media query (max-width: 768px). Depending on the screen width, the value of isMobile will be true or false. We can then conditionally render different content based on this value.
The useMediaQuery hook provides a straightforward and efficient way to create responsive components in ReactJS. In the next section, we’ll explore how to implement responsive components by utilizing the useMediaQuery hook and building upon the example above.
4. Implementing Responsive Components in ReactJS
Now that we have a grasp of the useMediaQuery hook, let’s dive into implementing responsive components in ReactJS. In this section, we’ll cover the necessary steps to create components that adapt to different screen sizes using the useMediaQuery hook.
4.1. Setting Up ReactJS and the useMediaQuery Hook
Before we begin, ensure that you have ReactJS installed in your project. If not, you can set up a new React project by following the official React documentation.
To use the useMediaQuery hook, we need to import it from the react-responsive package. Install it in your project by running the following command:
npm install react-responsive
Now, import the useMediaQuery hook in your component:
import { useMediaQuery } from 'react-responsive';
4.2. Creating a Responsive Component
Let’s say we want to create a responsive navigation menu that changes its layout and behavior based on the screen size. We’ll start by defining our component and using the useMediaQuery hook to detect the screen width:
import React from 'react'; import { useMediaQuery } from 'react-responsive'; const NavigationMenu = () => { const isMobile = useMediaQuery({ query: '(max-width: 768px)' }); return ( <nav> {isMobile ? ( <MobileMenu /> ) : ( <DesktopMenu /> )} </nav> ); }; const MobileMenu = () => { // Render mobile menu JSX here }; const DesktopMenu = () => { // Render desktop menu JSX here };
In the above code snippet, we define the NavigationMenu component. We use the useMediaQuery hook with the media query (max-width: 768px) to determine if the screen is mobile or not. Based on the result, we conditionally render either the MobileMenu or DesktopMenu component.
4.3. Styling Responsive Components
To create visually appealing responsive components, we can apply specific styles based on the screen size. We can utilize CSS-in-JS libraries like styled-components or inline styles to achieve this.
import React from 'react'; import { useMediaQuery } from 'react-responsive'; import styled from 'styled-components'; const NavigationMenu = () => { const isMobile = useMediaQuery({ query: '(max-width: 768px)' }); return ( <NavContainer isMobile={isMobile}> {isMobile ? ( <MobileMenu /> ) : ( <DesktopMenu /> )} </NavContainer> ); }; const NavContainer = styled.nav` /* Common styles for both mobile and desktop menu */ padding: 20px; /* Responsive styles */ background-color: ${props => props.isMobile ? 'lightblue' : 'lightgreen'}; `; const MobileMenu = styled.div` /* Styles for mobile menu */ `; const DesktopMenu = styled.div` /* Styles for desktop menu */ `;
In the code above, we use the styled-components library to define styles for our components. The NavContainer component applies common styles for both mobile and desktop menus, while also conditionally changing the background color based on the isMobile prop.
By utilizing the useMediaQuery hook and customizing the rendering and styling based on the screen size, we can create versatile and responsive components in ReactJS.
In the next section, we’ll discuss best practices for responsive component development to ensure a smooth and consistent user experience.
5. Best Practices for Responsive Component Development
When it comes to developing responsive components in ReactJS, it’s important to follow best practices to ensure a smooth and consistent user experience across different screen sizes. Let’s explore some key best practices to consider:
Define Component Breakpoints: Identify specific screen sizes or breakpoints where the component’s layout or behavior needs to change. By defining breakpoints, you can design your components to adapt gracefully to different screen sizes. For example, you might have different layouts for mobile, tablet, and desktop screens.
Utilize CSS Grid or Flexbox: CSS Grid and Flexbox provide powerful layout capabilities that simplify responsive design. Leverage these CSS features to create flexible and responsive grids or layouts for your components. They offer built-in mechanisms for handling different screen sizes without the need for complex calculations.
Use Responsive Images: Optimize image loading and display based on the screen size. Implement techniques like lazy loading or conditional rendering of images to ensure that only the necessary images are loaded for the current screen size. This improves performance and reduces bandwidth usage.
Test and Debug Responsiveness: Thoroughly test your components on different devices and screen sizes to ensure they behave as expected. Use browser developer tools to simulate various screen sizes and test for responsiveness. Debug any layout or styling issues that may arise and make necessary adjustments.
Here’s an example of implementing a responsive component with defined breakpoints using the useMediaQuery hook and styled-components:
import React from 'react'; import { useMediaQuery } from 'react-responsive'; import styled from 'styled-components'; const ResponsiveComponent = () => { const isSmallScreen = useMediaQuery({ query: '(max-width: 768px)' }); const isMediumScreen = useMediaQuery({ query: '(min-width: 769px) and (max-width: 1024px)' }); const isLargeScreen = useMediaQuery({ query: '(min-width: 1025px)' }); return ( <Container> {isSmallScreen && <SmallComponent />} {isMediumScreen && <MediumComponent />} {isLargeScreen && <LargeComponent />} </Container> ); }; const Container = styled.div` /* Container styles */ `; const SmallComponent = styled.div` /* Styles for small screen */ `; const MediumComponent = styled.div` /* Styles for medium screen */ `; const LargeComponent = styled.div` /* Styles for large screen */ `;
In the above code snippet, we define the ResponsiveComponent that adapts its rendering based on different screen sizes. We define media queries for small, medium, and large screens and conditionally render the corresponding components.
By following these best practices, you can create responsive components that provide an optimal user experience across various devices and screen sizes.
In the next section, we’ll conclude our discussion and recap the benefits of using responsive components with the ReactJS useMediaQuery hook.
6. Implementing Responsive Components in ReactJS
Now that we have a solid understanding of the ReactJS useMediaQuery hook and the importance of responsive components, let’s dive into implementing them in ReactJS. In this section, we will walk through the necessary steps to create responsive components that adapt to different screen sizes.
6.1. Setting Up ReactJS and useMediaQuery Hook
Make sure you have ReactJS installed in your project. If not, you can set up a new React project by following the official React documentation. To use the useMediaQuery hook, we need to import it from the ReactJS library:
import { useMediaQuery } from 'react-responsive';
6.2. Creating a Responsive Component
Let’s create a simple responsive component that renders different content based on the screen size. In this example, we will display a different message for mobile and desktop screens:
import React from 'react'; import { useMediaQuery } from 'react-responsive'; const ResponsiveComponent = () => { const isMobile = useMediaQuery({ query: '(max-width: 768px)' }); return ( <div> {isMobile ? ( <p>This is a mobile screen.</p> ) : ( <p>This is a desktop screen.</p> )} </div> ); }; export default ResponsiveComponent;
In the code above, we define the ResponsiveComponent functional component. We use the useMediaQuery hook to detect if the screen width is 768 pixels or less. Based on the result, we conditionally render different content.
6.3. Styling Responsive Components
To enhance the visual experience, we can apply specific styles to our responsive components based on the screen size. Let’s modify our previous example to style the messages differently for mobile and desktop screens:
import React from 'react'; import { useMediaQuery } from 'react-responsive'; const ResponsiveComponent = () => { const isMobile = useMediaQuery({ query: '(max-width: 768px)' }); return ( <div> <p style={isMobile ? mobileStyle : desktopStyle}> {isMobile ? 'This is a mobile screen.' : 'This is a desktop screen.'} </p> </div> ); }; const mobileStyle = { fontSize: '16px', color: 'blue', }; const desktopStyle = { fontSize: '20px', color: 'green', }; export default ResponsiveComponent;
In this updated code, we define two style objects, mobileStyle and desktopStyle, which contain different CSS properties for mobile and desktop screens. We apply these styles conditionally based on the isMobile variable.
By utilizing the ReactJS useMediaQuery hook and customizing the rendering and styling of components, we can create dynamic and responsive user interfaces that adapt gracefully to different screen sizes.
In the next section, we will discuss best practices for responsive component development to ensure a smooth and consistent user experience.
7. Best Practices for Responsive Component Development
Developing responsive components requires attention to detail and adherence to best practices. By following these guidelines, you can ensure a smooth and consistent user experience across different screen sizes. Let’s explore some best practices for responsive component development:
Define Breakpoints: Identify specific screen sizes where your component’s layout needs to adapt. Define breakpoints using media queries to handle layout changes effectively.
Use Flexibility and Grid Systems: Leverage CSS frameworks like Bootstrap or CSS features like Flexbox and CSS Grid to create flexible and responsive layouts.
Optimize Images: Implement techniques like lazy loading, responsive images, or srcset attributes to optimize image loading and ensure fast performance across devices.
Test Across Devices: Regularly test your responsive components on different devices and screen sizes to ensure they look and function as intended.
By following these best practices, you can create responsive components that deliver a seamless user experience on any device. In the next section, we’ll conclude our discussion and summarize the benefits of using responsive components with the ReactJS useMediaQuery hook.
Conclusion
In this blog post, we explored the ReactJS useMediaQuery hook and its capabilities for building responsive components. By leveraging this hook and following best practices, you can create dynamic and adaptable user interfaces that provide a consistent experience across devices. Embrace the power of responsive design and build engaging applications that cater to diverse screen sizes.
Table of Contents
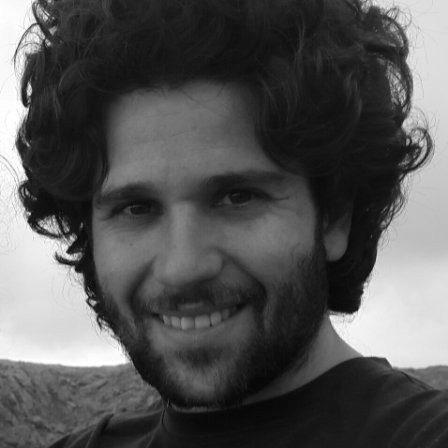
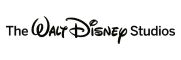