Reference Simplified: Unifying Multiple Refs with the useMergedRef Hook in ReactJS
This introduction highlights the importance of handling different user interactions, including long press events, in providing a more intuitive user experience in web development. It brings focus on useLongPress, a method within the ReactJS library that allows for simple and effective handling of these events. The blog post promises to explore the ReactJS landscape, understand the role of events, discover the importance of long press events, and delve into the workings of useLongPress. It aims to offer practical insights and tips for both beginners and experienced developers, with the goal of enhancing coding skills and bringing more interactivity to ReactJS projects.
Overview of ReactJS
The content provides an overview of ReactJS, an open-source JavaScript library used for creating dynamic and interactive user interfaces for single-page applications. React, developed by Facebook and a strong developer community, stands out for its focus on user interface, offering a component-based architecture for reusable code and more efficient state management. It uses a virtual DOM to update only the parts of the UI that change, making it fast and efficient. It also supports JSX, a syntax extension for JavaScript that simplifies the coding process. ReactJS is widely adopted due to its efficiency, flexibility, and strong community support, and is instrumental in handling long press events in components using the useLongPress feature, thus enhancing user interactions and simplifying development.
Understanding Events in ReactJS
This content explains the concept of events in ReactJS, which are actions detected by a web application and are critical for creating an interactive user experience. These can include a variety of user interactions like button clicks, form inputs, scrolling, and more. React uses its own event system, Synthetic Events, which is compatible with the W3C object model, providing a consistent API across all browsers.
Common types of events in ReactJS include:
- User Interface Events: These include load, unload, scroll, select, and error events.
- Form Events: Such as submit, change, focus, and blur.
- Keyboard Events: For instance, keypress, keydown, and keyup.
- Mouse Events: Such as click, double-click, mouseenter, mouseleave, and mouseover.
A less common but useful event type is the long press event, which is triggered when a user clicks and holds an element for an extended period. React’s ‘useLongPress’ simplifies handling these long press events, enabling more intricate user interactions.
The Need for Handling Long Press Events in Components
The content emphasizes the rising need for handling complex user interactions, such as long press events, in modern web development. Long press events can greatly enhance user experience and functionality by creating nuanced interactions, distinguishing between a regular click and a deliberate action by the user. However, managing these events in ReactJS can be complex due to the challenges of differentiating between simple clicks and long presses, and dealing with native browser events and JavaScript timers. React’s useLongPress feature simplifies this process by encapsulating these complexities into an easy-to-use hook, enabling developers to focus on app development instead of intricate event handling.
Introduction to useLongPress
The content introduces `useLongPress`, a custom hook in ReactJS designed to handle long press events effectively. This hook encapsulates the logic needed to distinguish between a simple click and a long press event by using JavaScript timers to measure the user’s interaction duration. By incorporating `useLongPress`, developers can enhance user interactions by differentiating between short press and long press actions, thus adding depth to their application’s UI without complicating their code with complex event handling. Furthermore, `useLongPress` offers flexibility as it can be applied to any component requiring user interaction, making it a versatile tool for developers. The subsequent sections promise to provide a detailed guide on implementing `useLongPress` and exploring advanced usage scenarios.
How to use useLongPress
Implementing `useLongPress` in your React project involves a few essential steps:
Step 1: Installation
If `useLongPress` is part of a library (like `react-use`), you first need to install the library using npm or yarn. If it’s a custom hook, ensure the hook is correctly defined in your project.
Step 2: Importing useLongPress
After installation, import the `useLongPress` hook into your component file.
import { useLongPress } from 'react-use';
Step 3: Define Actions
Next, define what should happen when a short click and a long press occur. These will be functions that `useLongPress` will trigger based on the user’s interaction.
const onShortPress = () => { console.log('short press'); }; const onLongPress = () => { console.log('long press'); };
Step 4: Implement useLongPress
Implement `useLongPress` by providing the short press and long press actions as parameters. You can also specify the duration that distinguishes between a short press and a long press (default is usually around 500ms).
const longPressEventHandlers = useLongPress(onShortPress, onLongPress, 500);
Step 5: Apply the Event Handlers
Finally, apply the `longPressEventHandlers` to the component where you want the long press interaction to be active.
<button {...longPressEventHandlers}>Press me</button>
In this example, when the button is clicked and released quickly, ‘short press’ will be logged to the console. If the button is clicked and held for more than 500 milliseconds, ‘long press’ will be logged to the console instead. This is a simple demonstration, but you can replace the console.log calls with any actions you need.
By following these steps, you can integrate `useLongPress` into your React components. Next, let’s explore some advanced uses of `useLongPress` to truly leverage its capabilities.
Advanced uses of useLongPress
`useLongPress` is a powerful tool that offers more than just distinguishing between short and long press events. It can be tailored to fit a variety of advanced use cases to further enhance your application’s interactivity.
1. Customizing Duration
The time that defines a long press can be adjusted based on the specific needs of your application. For instance, if you want a long press event to trigger after a full second, you can set the delay parameter to 1000 milliseconds.
const longPressEventHandlers = useLongPress(onShortPress, onLongPress, 1000);
2. Handling Continuous Long Presses
`useLongPress` can be used to handle continuous long presses, where an action repeats while the user keeps pressing. This is useful for scenarios like increasing or decreasing a value continuously as the user presses.
const onLongPress = () => { setInterval(() => { console.log('continuously pressing'); }, 500); }; const longPressEventHandlers = useLongPress(onShortPress, onLongPress, 500);
3. Leveraging Different Events
`useLongPress` can be used with different types of events, not just onClick. This means you can leverage it to handle touch events for mobile-first applications or even events associated with keyboard keys or mouse actions.
const longPressKeyEventHandlers = useLongPress(onShortPress, onLongPress, 500); const longPressTouchEventHandlers = useLongPress(onShortPress, onLongPress, 500); <button {...longPressKeyEventHandlers} onKeyDown={longPressKeyEventHandlers}>Key Press</button> <div {...longPressTouchEventHandlers} onTouchStart={longPressTouchEventHandlers}>Touch Press</div>
4. Nested Components
`useLongPress` can be used in nested components to provide different long press interactions based on the specific element being pressed. This can add depth to your application’s interactivity.
Remember that while these are some advanced uses of `useLongPress`, the possibilities are endless, and it’s all about how creatively you leverage this hook in your applications.
Tips and Best Practices
When using `useLongPress` in your ReactJS projects, the following tips and best practices can help you achieve optimal results:
- Avoid Complex Operations in Handlers: Keep `onShortPress` and `onLongPress` handlers simple to prevent delayed response times and improve user experience.
- Test Across Different Devices: Ensure consistent user experience by testing long press functionality across various devices and screen sizes.
- Provide User Feedback: Implement visual indicators to signify when a long press action is triggered.
- Use Appropriate Duration: Set thoughtful durations for distinguishing between a short click and a long press to prevent unintentional triggers or frustrating user experiences.
- Combine with Other Events: Use `useLongPress` with other events such as keyboard or mouse events for a more comprehensive interaction model.
- Manage Component Unmounting: Clear any timers or intervals when unmounting the component to avoid potential memory leaks.
These tips can guide developers to effectively use `useLongPress`, leading to more dynamic and interactive user interfaces.
Conclusion
This blog post summarizes the importance of handling long press events in ReactJS using the `useLongPress` hook. This feature offers considerable advantages, including differentiating between short clicks and long presses and providing nuanced user interactions.
The post delved into the specifics of ReactJS and its events, particularly long press events. Readers were guided through how to use `useLongPress`, its advanced usage, and shared practical tips to maximize its benefits. Implementing long press events with `useLongPress` can significantly enhance user experience by adding sophistication and interactivity to applications, thus empowering developers to take their projects to new levels.
Table of Contents
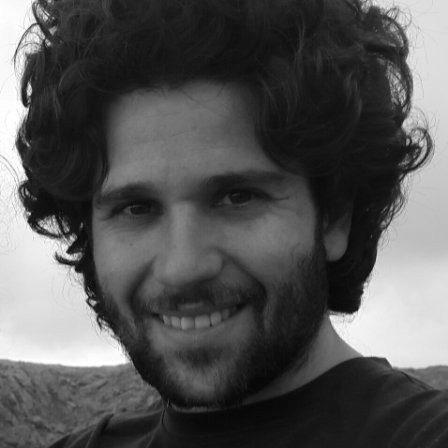
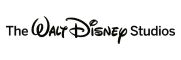