Embrace Efficiency in ReactJS with useMultiState for Multi-State Management
ReactJS has revolutionized the landscape of web development with its modular approach and effective management of data via state and props. One powerful feature is its use of hooks, empowering developers to access React state and lifecycle features from functional components.
However, handling multiple state variables in a component can be complex. As of my last update in September 2021, React doesn’t offer a built-in hook to manage multiple state variables. But this doesn’t limit experienced developers – like those you might hire as ReactJS developers – from creating custom hooks to simplify this process. One such custom hook we’ll explore here is `useMultiState`.
In this blog post, we will delve into creating and utilizing `useMultiState`, a custom hook designed to manage multiple state variables in a single ReactJS component efficiently. This way, whether you’re an aspiring coder or looking to hire ReactJS developers, you’ll gain valuable insights into simplifying state management in React.
What is `useMultiState`?
The `useMultiState` is a custom ReactJS hook that we will define. This hook is aimed to handle multiple state variables within a single hook, making the code cleaner and easier to manage.
Creating the `useMultiState` Hook
Let’s start by defining our custom hook. For the sake of simplicity, let’s create a hook that can manage two state variables.
```javascript import { useState } from 'react'; const useMultiState = (initialState1 = null, initialState2 = null) => { const [state1, setState1] = useState(initialState1); const [state2, setState2] = useState(initialState2); const setMultiState = (newState1, newState2) => { setState1(newState1); setState2(newState2); }; return [state1, state2, setMultiState]; }; ```
In the above code, we have defined our `useMultiState` hook, which takes two initial states as arguments and returns two state variables along with a setter function, `setMultiState`, that sets both state variables at once.
Using the `useMultiState` Hook
To use our `useMultiState` hook, let’s create a simple component that maintains two state variables: a count and a toggle.
```javascript import React from 'react'; import useMultiState from './useMultiState'; const MyComponent = () => { const [count, toggle, setMultiState] = useMultiState(0, false); const handleClick = () => { setMultiState(count + 1, !toggle); }; return ( <div> <p>Count: {count}</p> <p>Toggle: {toggle ? 'On' : 'Off'}</p> <button onClick={handleClick}>Click me</button> </div> ); }; export default MyComponent; ```
In this component, when the user clicks the button, the count will be increased by one, and the toggle state will be switched.
Creating a more generic `useMultiState`
While our first example of `useMultiState` works for two state variables, it’s not flexible enough to handle any number of state variables. So let’s create a more generic version.
```javascript import { useState } from 'react'; const useMultiState = (initialStates = {}) => { const [state, setState] = useState(initialStates); const setMultiState = newState => { setState(prevState => ({ ...prevState, ...newState, })); }; return [state, setMultiState]; }; ```
This version of `useMultiState` takes an object of initial states, which can have any number of properties, and returns a state object and a setter function. The setter function merges the new state with the current state, ensuring that all state variables are updated correctly.
Using the generic `useMultiState`
Let’s modify our component to use this new, more flexible `useMultiState`.
```javascript import React from 'react'; import useMultiState from './useMultiState'; const MyComponent = () => { const [state, setMultiState] = useMultiState({ count: 0, toggle: false }); const handleClick = () => { setMultiState({ count: state.count + 1, toggle: !state.toggle }); }; return ( <div> <p>Count: {state.count}</p> <p>Toggle: {state.toggle ? 'On' : 'Off'}</p> <button onClick={handleClick}>Click me</button> </div> ); }; export default MyComponent; ```
Now our `MyComponent` handles any number of state variables. When the user clicks the button, the `handleClick` function increments the count and flips the toggle state using the `setMultiState` function.
Conclusion
This blog post delves into the potential of managing multiple state variables in ReactJS using a custom hook – `useMultiState`. This powerful tool simplifies multi-state management, offering a cleaner, understandable coding process. It underscores the adaptability of ReactJS, highlighting how custom hooks can fill gaps when built-in tools don’t quite meet your needs. Remember, `useMultiState` may not fit all use cases, but it’s an excellent starting point that can be tailored to fit your requirements. Custom hooks follow certain rules which must be respected to ensure their successful implementation. As you continue your ReactJS journey, you will find more patterns and techniques to write efficient, maintainable code. For those seeking expert help, consider hiring ReactJS developers who are well-versed in creating and using hooks like `useMultiState`.
Table of Contents
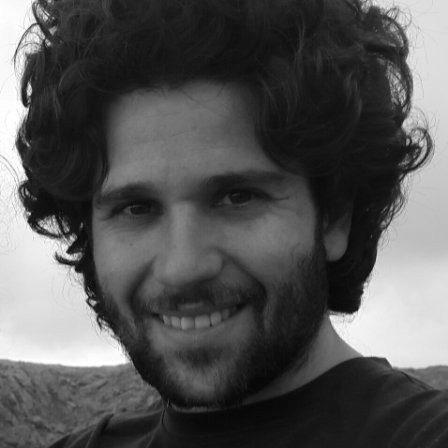
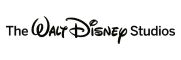