ReactJS’s useOnlineStatus hook for detecting user connectivity status in web applications
In today’s digital world, maintaining online connectivity and understanding user’s online status are critical for developers in crafting reliable web applications. Such a feature enhances user experience by managing situations of intermittent internet connection, offering pertinent feedback, and providing smooth offline-to-online transitions.
Online status monitoring is key in many modern web applications, such as real-time data syncing in apps, managing downloads in streaming services, or saving drafts on blogging platforms.
ReactJS, a widely-used JavaScript library for constructing user interfaces, is not only known for its efficient rendering and component-based architecture, but also for its tools like the useOnlineStatus hook that aid in tracking user connectivity status. This tool allows for better resilience and user-friendliness in applications.
Join us as we explore the useOnlineStatus hook and how it can amplify the capabilities of our ReactJS applications.
What is ReactJS?
ReactJS, also known as React, is a renowned JavaScript library developed by Facebook in 2013 for creating dynamic user interfaces. It’s well-received for its adaptability, efficiency, and powerful features, with a broad range of tech companies globally using it in their production.
React’s hallmark is its component-based architecture, which allows developers to build self-sufficient components that manage their state. These components can then be combined to construct intricate user interfaces, promoting reusability and simplifying large application development.
Additionally, React employs the Virtual DOM, optimizing performance by only updating components in the actual DOM that differ from the Virtual DOM.
React caters to both web (ReactJS) and mobile (React Native) application development, standing out due to its user-friendly nature, superior performance, and expansive ecosystem.
Understanding User Connectivity Status
User connectivity status, referring to the online or offline state of a user’s internet connection, is integral to building dynamic and user-friendly web applications. Modern web technologies allow apps to detect network alterations and respond effectively to varying connectivity situations.
For example, if a user loses internet connection, an application might transition to an offline mode, storing user modifications locally until connectivity is restored. The application can then sync these local changes with the server when back online, thereby enhancing user experience.
User connectivity status can also assist in postponing non-essential operations during offline periods or offering low-bandwidth alternatives during weak internet connections.
In today’s age of progressive web apps (PWAs) and widespread mobile devices, handling user connectivity status has become increasingly important. Users anticipate applications to function irrespective of their connectivity status.
Next, we’ll delve into how JavaScript and ReactJS, specifically the useOnlineStatus hook, can effectively detect and manage changes in user connectivity status.
Detecting User Connectivity in JavaScript
Detecting user connectivity in JavaScript is made possible by a property of the window’s `Navigator` object called `onLine`. The `Navigator.onLine` property provides a boolean value that signifies whether the system is online or offline.
Here’s a simple JavaScript snippet demonstrating how to use it:
if (navigator.onLine) { console.log("You are online!"); } else { console.log("Oops, you are offline."); }
While this API provides a quick way to determine the connectivity status, it’s not without its limitations. The `Navigator.onLine` property only tells you if the system is capable of network communication. It does not guarantee that the system can actually reach the internet. For example, a device could be connected to a network, but the network might not have internet access. In such a case, `navigator.onLine` will still return `true`.
Additionally, the `Navigator.onLine` property does not provide any information about the quality of the connection. A slow or unstable connection might be almost as problematic as no connection at all, but `Navigator.onLine` won’t differentiate.
One common workaround to these limitations is to make a simple fetch request to a reliable server and see if it succeeds. However, this can add unnecessary overhead, especially if done frequently.
In the next section, we’ll look at the `useOnlineStatus` hook in ReactJS, which provides a more nuanced approach to handling connectivity status in a web application.
Introduction to useOnlineStatus Hook in ReactJS
In ReactJS, a Hook is a special function that allows you to “hook into” React’s features. Hooks were introduced in React 16.8, allowing you to use state and other features of React without having to write a class.
The `useOnlineStatus` hook is not a built-in hook provided by React, but it’s a common example of a custom hook that developers create to work with the user’s online status. Its job is to provide you with a simple and efficient way to monitor the online/offline status of a user in a React component.
Here’s a basic implementation of a `useOnlineStatus` hook:
import { useState, useEffect } from 'react'; function useOnlineStatus() { const [isOnline, setIsOnline] = useState(navigator.onLine); const updateOnlineStatus = () => { setIsOnline(navigator.onLine); }; useEffect(() => { window.addEventListener('online', updateOnlineStatus); window.addEventListener('offline', updateOnlineStatus); return () => { window.removeEventListener('online', updateOnlineStatus); window.removeEventListener('offline', updateOnlineStatus); }; }, []); return isOnline; }
In this hook, we first create a state variable `isOnline` using the `useState` hook, initializing it with the current online status (`navigator.onLine`). We then define a function `updateOnlineStatus` that updates this state whenever the online status changes.
We use the `useEffect` hook to add event listeners for the `online` and `offline` events, which will call our `updateOnlineStatus` function whenever the user goes online or offline. We also return a cleanup function that removes these event listeners when the component unmounts.
Finally, the `useOnlineStatus` hook returns the current online status (`isOnline`), which you can use in your components to react to changes in the user’s online status.
In the next section, we’ll go deeper into how to implement and use this `useOnlineStatus` hook in a ReactJS application.
Implementing useOnlineStatus Hook
Implementing the `useOnlineStatus` hook involves encapsulating the logic for monitoring online/offline status into a custom hook, which can then be used by any component in your React application. Here’s a step-by-step guide on how to do this:
Step 1: Create a new JavaScript file for your custom hook, such as `useOnlineStatus.js`.
Step 2: Import the necessary hooks from React. We will need `useState` and `useEffect`.
import { useState, useEffect } from 'react';
Step 3: Define the `useOnlineStatus` function.
In this function, we’ll first create a state variable `isOnline` that will hold the current online status. We’ll initialize this with the current value of `navigator.onLine`.
We then define a function `updateOnlineStatus` that sets `isOnline` based on the current value of `navigator.onLine`.
function useOnlineStatus() { const [isOnline, setIsOnline] = useState(navigator.onLine); const updateOnlineStatus = () => { setIsOnline(navigator.onLine); }; // ... more to come … }
Step 4: Add event listeners for the `online` and `offline` events.
We’ll do this inside a `useEffect` hook, which runs after every render. When the `online` or `offline` event is fired, our `updateOnlineStatus` function will be called, updating our `isOnline` state.
In the cleanup function returned by `useEffect`, we remove the event listeners.
useEffect(() => { window.addEventListener('online', updateOnlineStatus); window.addEventListener('offline', updateOnlineStatus); return () => { window.removeEventListener('online', updateOnlineStatus); window.removeEventListener('offline', updateOnlineStatus); }; }, []);
Step 5: Finally, our `useOnlineStatus` function returns the current `isOnline` status.
return isOnline;
Step 6: Export the `useOnlineStatus` function as a default export from the file.
export default useOnlineStatus;
Step 6: Export the `useOnlineStatus` function as a default export from the file.
export default useOnlineStatus;
That’s it! We now have a custom `useOnlineStatus` hook.
You can now import and use this hook in any of your components like this:
import useOnlineStatus from './useOnlineStatus'; function MyComponent() { const isOnline = useOnlineStatus(); return <div>{isOnline ? "You're online!" : "You're offline."}</div>; }
This component will display “You’re online!” when the user is online and “You’re offline.” when they’re not. It will automatically update whenever the user’s online status changes.
Advantages of Using useOnlineStatus Hook
Using the `useOnlineStatus` hook in your React applications can bring several advantages:
The `useOnlineStatus` hook in ReactJS presents several advantages:
- Code Reusability and Encapsulation:
It encloses the logic for online status tracking, promoting code reuse across various components without code duplication, thus fostering cleaner and more maintainable code.
- Automatic Updates:
React automatically updates any components using the `useOnlineStatus` hook whenever a user’s online status changes, facilitating the creation of dynamic interfaces responsive to online status changes.
- Simplified Code:
By using hooks, components can remain as functional components instead of class components, resulting in simpler, more readable, and consistent code.
- Enhanced User Experience:
By appropriately responding to online status (such as local data saving when offline or user warnings), user experience is significantly improved.
- Easy Integration:
Hooks integrate easily with other React features and hooks. For instance, the `useOnlineStatus` hook can be paired with the `useEffect` hook to perform actions only when the user is online.
These advantages make `useOnlineStatus` an invaluable tool when dealing with user connectivity in React applications.
Limitations and Possible Workarounds for useOnlineStatus Hook
While the `useOnlineStatus` hook is a powerful tool, it’s not without its limitations:
- Limited Information:
The `navigator.onLine` property that the hook relies on can only provide binary online/offline information. It doesn’t offer any insights into the quality or speed of the user’s connection.
Workaround:
To handle this, you could implement a separate function that periodically tests the actual connection speed by fetching a small resource from your server and measuring the time it takes.
- Not Always Accurate:
The `navigator.onLine` property may return `true` even when the user’s device is connected to a network but doesn’t have internet access (like a captive portal or a network issue).
Workaround:
As a workaround, you can occasionally ping a server endpoint to verify that the user not only has a network connection but also has actual internet access.
- Dependency on Window Object:
The hook depends on the window object and its events, so it won’t work in environments where this isn’t available, like server-side rendering (SSR) or React Native.
Workaround:
For server-side rendering, you could design your components to assume they’re online by default and then adjust once they mount in the client’s browser. For React Native, you could use the `NetInfo` API instead.
Remember, the purpose of the `useOnlineStatus` hook is to improve your app’s user experience by gracefully handling changes in the user’s online status. While it’s not perfect, with a few tweaks and careful usage, it can be a highly valuable tool for modern web applications.
Conclusion
In summary, the `useOnlineStatus` hook provides an efficient way to handle user connectivity status in ReactJS applications. Its implementation can improve user experience, support code reusability, and simplify your codebase. While there are limitations, such as a lack of detailed connectivity information and reliance on the window object, strategic workarounds can mitigate these. By leveraging `useOnlineStatus` and other ReactJS features, developers can create more dynamic, reliable, and user-centric web applications, ready for the realities of today’s unpredictable internet connectivity environments.
Table of Contents
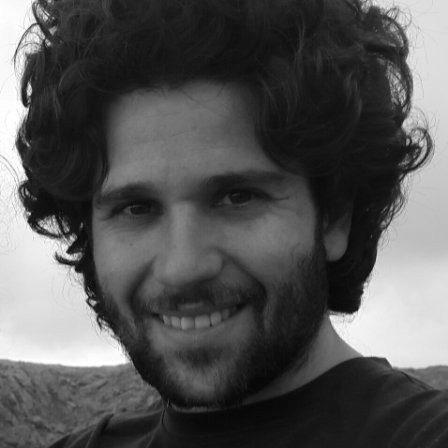
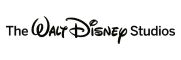