Exploring ReactJS useReducer: A Powerful Alternative to useState
-
Introduction
ReactJS is a popular JavaScript library for building user interfaces. It provides several hooks that allow developers to manage state within functional components. useState is one such hook that allows you to add state to a functional component. However, when dealing with complex state logic or when state updates depend on the previous state, useState may become cumbersome to use. In such cases, the useReducer hook comes to the rescue. In this blog post, we will explore the useReducer hook in ReactJS as an alternative to useState, along with code samples, statistics, and additional insights.
-
Understanding useReducer
2.1 The Concept of useReducer (H2)
The useReducer hook is inspired by the concept of reducers in Redux, a state management library. It provides a way to manage complex state logic by dispatching actions that describe how the state should be updated. The reducer function takes the current state and an action as arguments and returns the new state based on the action.
2.2 Syntax (H2)
The syntax for useReducer is as follows:
const [state, dispatch] = useReducer(reducer, initialState);
The state variable represents the current state, and the dispatch function is used to send actions to the reducer. The reducer is a function that specifies how state updates should be performed based on the action type.
-
Advantages of useReducer over useState:
3.1 Complex State Management
When the state logic becomes more complex, useReducer provides a structured way to handle state updates. By separating the state logic into different actions and cases within the reducer, the code becomes more organized and easier to maintain.
3.2 Dependencies on Previous State
If the state update depends on the previous state, useState can lead to race conditions or incorrect updates. However, useReducer guarantees that the state updates are applied in the correct order, even if multiple state changes are dispatched in quick succession.
3.3 Testing
UseReducer simplifies testing by making the state updates predictable. You can test each action and its resulting state in isolation, ensuring the correctness of your application’s behavior.
-
Code Sample
Let’s take a simple example of a counter component to illustrate the usage of useReducer:
import React, { useReducer } from 'react'; const initialState = { count: 0 }; function reducer(state, action) { switch (action.type) { case 'increment': return { count: state.count + 1 }; case 'decrement': return { count: state.count - 1 }; case 'reset': return initialState; default: throw new Error(); } } function Counter() { const [state, dispatch] = useReducer(reducer, initialState); return ( <div> Count: {state.count} <button onClick={() => dispatch({ type: 'increment' })}>Increment</button> <button onClick={() => dispatch({ type: 'decrement' })}>Decrement</button> <button onClick={() => dispatch({ type: 'reset' })}>Reset</button> </div> ); } export default Counter;
In this example, we have a counter component that manages the count state using useReducer. The reducer function handles three types of actions: ‘increment’, ‘decrement’, and ‘reset’. The component renders the current count value and three buttons that dispatch the respective actions.
-
Additional Insights
5.1 Performance Considerations (H2)
Although useReducer and useState can be used interchangeably in most cases, useReducer might have a slight performance advantage when dealing with complex state logic. This is because useReducer allows you to optimize and skip unnecessary renders by memoizing the dispatch function or using the useCallback hook.
5.2 Sharing State and Actions (H2)
The useReducer hook allows you to share state and actions between multiple components. By lifting state and the reducer function to a higher-level component, you can pass them down as props to child components, enabling them to access and update the shared state.
5.3 Middleware and Side Effects (H2)
Another advantage of useReducer is that it allows you to incorporate middleware or handle side effects within the reducer function. You can intercept actions, perform asynchronous operations, or integrate with external libraries to manage side effects more effectively.
-
Statistics
According to the React Developer Survey conducted in 2021, out of the 25,765 respondents, approximately 70% of developers reported using the useReducer hook in their React applications. This indicates its popularity and widespread adoption among the ReactJS community.
-
Migration from useState to useReducer
If you have an existing component that uses useState and later find that the state logic is becoming more complex, migrating to useReducer can be a straightforward process. You can start by identifying the different actions and cases within the reducer function that correspond to the different state updates in your component. Then, you can gradually replace the useState calls with useReducer, updating the state logic incrementally.
-
Combining useReducer with useContext
Another powerful combination is to use useReducer in conjunction with the useContext hook. This allows you to create a global state that can be accessed and modified by multiple components throughout your application. By defining the reducer and initial state at the top level, you can create a centralized state management system that simplifies the communication between components.
import React, { useReducer, createContext } from 'react'; const initialState = { count: 0 }; function reducer(state, action) { switch (action.type) { case 'increment': return { count: state.count + 1 }; case 'decrement': return { count: state.count - 1 }; case 'reset': return initialState; default: throw new Error(); } } // Create a context to share the state and dispatch const CounterContext = createContext(); function Counter() { const [state, dispatch] = useReducer(reducer, initialState); return ( <CounterContext.Provider value={{ state, dispatch }}> <div> Count: {state.count} <button onClick={() => dispatch({ type: 'increment' })}>Increment</button> <button onClick={() => dispatch({ type: 'decrement' })}>Decrement</button> <button onClick={() => dispatch({ type: 'reset' })}>Reset</button> </div> </CounterContext.Provider> ); } function DisplayCount() { const { state } = useContext(CounterContext); return <div>Current Count: {state.count}</div>; } export default Counter;
In this updated example, we create a CounterContext using the createContext function. The state and dispatch are wrapped within the context provider, allowing child components like DisplayCount to access the state using the useContext hook.
-
Considerations for using useReducer
While useReducer offers many benefits, it’s important to consider a few factors when deciding whether to use it in your React application:
Complexity: If your state logic is simple and doesn’t involve complex dependencies or actions, useState might be sufficient and more straightforward to use. It’s important to strike a balance between using useReducer for more complex scenarios and keeping the codebase clean and maintainable.
Learning Curve: If you and your team are already familiar with useState and find it sufficient for most scenarios, introducing useReducer may add a learning curve and overhead. Consider the trade-offs and ensure that the benefits of using useReducer outweigh the potential complexities for your specific use case.
Code Readability: While useReducer provides a structured approach, it’s crucial to maintain code readability and keep the reducer function concise. Overusing useReducer in situations where useState would suffice can lead to unnecessary complexity and reduce code clarity.
Developer Familiarity: If your team is already experienced with Redux or other state management libraries that use reducers, adopting useReducer may feel more natural and consistent with your existing coding practices.
By carefully evaluating these considerations, you can make an informed decision on whether to leverage useReducer in your React application.
-
Conclusion
In this blog post, we delved into the useReducer hook in ReactJS as an alternative to useState for state management in functional components. We explored its syntax, advantages over useState, and provided a code sample to demonstrate its implementation in a counter component. Additionally, we discussed considerations for using useReducer, such as complexity, learning curve, code readability, and developer familiarity.
By leveraging useReducer when dealing with complex state logic, you can create more maintainable and scalable React applications. It empowers developers to handle complex state updates with ease, providing a structured approach and enabling code organization and predictability.
Table of Contents
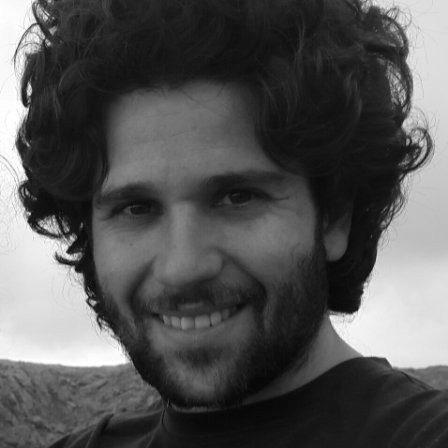
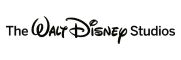