Next-Level State Management: Fusing useReducer and useContext with ReactJS’s useReducerWithContext Hook
React’s `useReducer` and `useContext` are both hooks that allow developers to handle state and share it across components without “prop drilling”.
useReducer
`useReducer` is typically preferable when you have complex state logic that involves multiple sub-values. It allows you to manage the state of your application similar to how you would with Redux, but without needing to include an additional library in your project.
The `useReducer` hook uses a reducer function to determine changes to the state. This function accepts the current state and an action, and returns the new state based on those inputs.
useContext
`useContext` lets you share a state, and any functionality related to that state, to any nested child components without passing props down manually at every level.
The Concept of useReducerWithContext
`useReducerWithContext` is not an official React hook but a pattern where we use these two hooks together to manage global state. It involves creating a context with `createContext` that will provide the state and dispatch function from `useReducer` to the component tree.
Let’s walk through how to implement the `useReducerWithContext` pattern in a React application.
Setting up useReducerWithContext
To kick off, we’ll define our state and create an initial state object:
const initialState = { user: null, posts: [], };
Next, we’ll define the reducer function. This function listens to dispatched actions and returns the new state:
const reducer = (state, action) => { switch (action.type) { case 'SET_USER': return { ...state, user: action.payload }; case 'SET_POSTS': return { ...state, posts: action.payload }; default: return state; } };
The next step is to create a context using `createContext`. This context will provide our state and dispatch function to any nested components that need it:
import { createContext } from 'react'; export const StateContext = createContext();
Now, we’ll use `useReducer` within a context provider component. This provider component will wrap around any other components that need access to the state:
import { useReducer } from 'react'; import { StateContext } from './StateContext'; export const StateProvider = ({ children }) => { const [state, dispatch] = useReducer(reducer, initialState); return ( <StateContext.Provider value={{ state, dispatch }}> {children} </StateContext.Provider> ); };
Lastly, we’ll use the `StateProvider` to wrap our application in the root component:
import { StateProvider } from './StateProvider'; function App() { return ( <StateProvider> {/* other components */} </StateProvider> ); } export default App;
Using State in Components
To use the state in a component, we’ll call `useContext` and pass in our `StateContext`. This will give us access to the state and the dispatch function:
import React, { useContext } from 'react'; import { StateContext } from './StateContext'; const MyComponent = () => { const { state, dispatch } = useContext(StateContext); // we can now use state and dispatch in our component };
We can now dispatch actions to update the state:
dispatch({ type: 'SET_USER', payload: { name: 'John Doe', age: 30 } });
And we can access the state values:
console.log(state.user); // { name: 'John Doe', age: 30 }
Conclusion
The `useReducerWithContext` pattern provides a simple, effective, and “React-ish” way to manage global state in your application. This pattern allows for a cleaner, more organized, and testable codebase that’s easier to understand and maintain.
The power of `useReducerWithContext` lies in its simplicity and flexibility, harnessing the power of React’s built-in hooks to deliver a lightweight, robust state management solution. While libraries like Redux offer additional features and tools, they may be overkill for many applications. The `useReducerWithContext` pattern offers a middle ground solution that is both powerful and simple to implement.
Remember that each application is different, and the best state management solution depends on the specific needs of your project. As always, the key to mastering state management, or any other aspect of development, is practice.
Happy coding!
Table of Contents
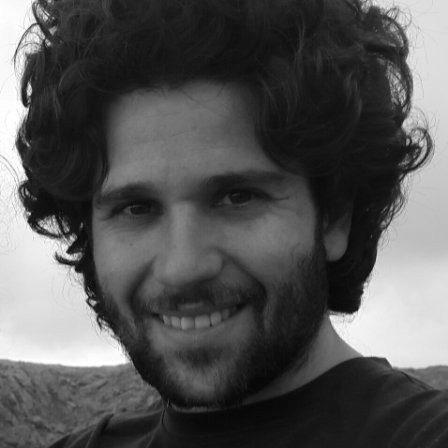
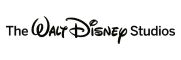