Tracking Scroll Position in React Components with the useScrollPosition Hook
Scroll position tracking is a crucial aspect of web development, especially when building dynamic and interactive user interfaces. In ReactJS, tracking the scroll position allows developers to create components that respond to the user’s scrolling behavior. Fortunately, React provides a powerful hook called useScrollPosition that simplifies the process of tracking and utilizing scroll position data. In this blog, we will explore the useScrollPosition hook, how to implement it in React components, and various advanced techniques and use cases.
1. What is Scroll Position Tracking?
Scroll position tracking refers to monitoring and capturing the vertical and horizontal position of the user’s viewport within a web page. This information is valuable for understanding user engagement, creating animations, lazy loading content, or triggering specific actions based on scroll position.
2. Why is Scroll Position Tracking Important in ReactJS?
ReactJS is a popular JavaScript library for building user interfaces, and scroll position tracking plays a significant role in enhancing the user experience. By tracking the scroll position, React components can dynamically update their appearance, load additional content as the user scrolls, or trigger animations based on the user’s interaction.
3. The useScrollPosition Hook
3.1 Overview of the useScrollPosition Hook
React provides a variety of built-in hooks, and one of them is the useScrollPosition hook. This hook allows developers to track the scroll position and access the scroll position data within functional components.
3.2 How to Install and Import the useScrollPosition Hook
Before using the useScrollPosition hook, you need to install the appropriate package. Open your terminal and run the following command:
npm install react-use-scroll-position
After installing the package, you can import the useScrollPosition hook into your React component:
import { useScrollPosition } from 'react-use-scroll-position';
3.3 Tracking Scroll Position Using the useScrollPosition Hook
To track the scroll position in your component, you can call the useScrollPosition hook within the component’s body. Here’s an example:
import { useScrollPosition } from 'react-use-scroll-position'; const MyComponent = () => { useScrollPosition(({ scrollX, scrollY }) => { // Access and utilize scroll position data console.log('Scroll position:', scrollX, scrollY); // Perform additional actions based on scroll position // ... }); return ( // JSX code for your component's rendering ); };
In the above example, the useScrollPosition hook takes a callback function as its argument. The callback function receives an object containing the scrollX and scrollY properties representing the current scroll position. Inside the callback, you can access and utilize the scroll position data for various purposes.
4. Implementing Scroll Position Tracking in React Components
4.1 Creating a ScrollPositionTracker Component
Let’s create a reusable component called ScrollPositionTracker that encapsulates the scroll position tracking functionality. This component will allow us to easily integrate scroll position tracking into any other React component.
import { useScrollPosition } from 'react-use-scroll-position'; const ScrollPositionTracker = ({ children }) => { useScrollPosition(({ scrollX, scrollY }) => { // Access and utilize scroll position data console.log('Scroll position:', scrollX, scrollY); // Perform additional actions based on scroll position // ... }); return <>{children}</>; }; export default ScrollPositionTracker;
4.2 Adding the useScrollPosition Hook to the Component
Now, let’s integrate the useScrollPosition hook into a sample component, MyComponent, using the ScrollPositionTracker component we created earlier.
import ScrollPositionTracker from './ScrollPositionTracker'; const MyComponent = () => { return ( <ScrollPositionTracker> {/* JSX code for your component's rendering */} </ScrollPositionTracker> ); }; export default MyComponent;
By wrapping MyComponent with the ScrollPositionTracker component, we enable scroll position tracking within MyComponent.
4.3 Accessing the Scroll Position Data
Inside MyComponent, you can access the scroll position data and utilize it according to your needs. For example:
import { useState } from 'react'; import ScrollPositionTracker from './ScrollPositionTracker'; const MyComponent = () => { const [scrollTop, setScrollTop] = useState(0); useScrollPosition(({ scrollY }) => { // Update the scroll top state setScrollTop(scrollY); }); return ( <ScrollPositionTracker> <div> <h1>Scroll Position: {scrollTop}</h1> {/* JSX code for the rest of your component */} </div> </ScrollPositionTracker> ); }; export default MyComponent;
In the above example, we use the useState hook to maintain the scrollTop state. The useScrollPosition hook updates the scrollTop state whenever the scroll position changes. This allows us to display the current scroll position in the rendered component.
5. Advanced Techniques and Use Cases
5.1 Debouncing Scroll Events
In some cases, it may be necessary to debounce scroll events to optimize performance and prevent excessive updates. Debouncing ensures that a function is only executed after a certain period of inactivity. We can achieve this by using a debounce function from a utility library like Lodash or by implementing our own debounce logic.
Here’s an example of debouncing scroll events when using the useScrollPosition hook:
import { useState, useEffect } from 'react'; import { debounce } from 'lodash'; import { useScrollPosition } from 'react-use-scroll-position'; const MyComponent = () => { const [scrollTop, setScrollTop] = useState(0); const handleScroll = debounce(({ scrollY }) => { setScrollTop(scrollY); }, 100); // Debounce with a 100ms delay useEffect(() => { return () => { handleScroll.cancel(); // Cancel the debounced scroll handler on unmount }; }, [handleScroll]); useScrollPosition(handleScroll); return ( // JSX code for your component's rendering ); }; export default MyComponent;
In this example, we use the debounce function from the Lodash library to create a debounced version of the scroll handler function. The debounced handleScroll function is called with a delay of 100ms, ensuring that it will only be executed once after a period of inactivity. The debounced function is also canceled when the component unmounts to avoid memory leaks.
5.2 Using Scroll Position for Animations
Scroll position tracking can be leveraged to create captivating animations that respond to the user’s scrolling. By adjusting CSS properties or applying CSS classes based on the scroll position, we can create visually appealing effects.
Here’s an example of animating an element’s opacity based on the scroll position:
import { useState } from 'react'; import { useScrollPosition } from 'react-use-scroll-position'; const MyComponent = () => { const [opacity, setOpacity] = useState(0); useScrollPosition(({ scrollY }) => { const newOpacity = Math.min(scrollY / 500, 1); // Adjust the division value to control the animation range setOpacity(newOpacity); }); return ( <div className="my-element" style={{ opacity }}> {/* Content */} </div> ); }; export default MyComponent;
In this example, we calculate the opacity based on the scroll position divided by a specific value. As the user scrolls, the opacity state is updated accordingly, creating a smooth opacity transition for the my-element component.
5.3 Lazy Loading Content Based on Scroll Position
Lazy loading content can significantly improve the performance of web pages, especially when dealing with large amounts of data or media. By tracking the scroll position, we can selectively load content as the user approaches the relevant sections of the page.
Here’s an example of lazy loading images based on the scroll position:
import { useState } from 'react'; import { useScrollPosition } from 'react-use-scroll-position'; const MyComponent = () => { const [showImage, setShowImage] = useState(false); useScrollPosition(({ scrollY }) => { const imagePosition = document.getElementById('image-section').offsetTop; const windowHeight = window.innerHeight; if (scrollY > imagePosition - windowHeight * 0.75) { setShowImage(true); } }); return ( <div> {/* Other content */} <div id="image-section"> {showImage && <img src="path/to/image.jpg" alt="Lazy Loaded Image" />} </div> {/* Other content */} </div> ); }; export default MyComponent;
In this example, we use the scroll position to determine when the image-section is close to being visible in the viewport. Once the section is within 75% of the viewport’s height, we set the showImage state to true, rendering the lazy-loaded image component.
Conclusion
Tracking the scroll position in ReactJS is a powerful technique that allows developers to create dynamic and interactive components. By utilizing the useScrollPosition hook, we can easily access the scroll position data and build various functionalities such as debouncing scroll events, animating elements based on scroll position, and lazy loading content. Incorporating scroll position tracking in your React applications can enhance user experiences and provide a more engaging interface.
Table of Contents
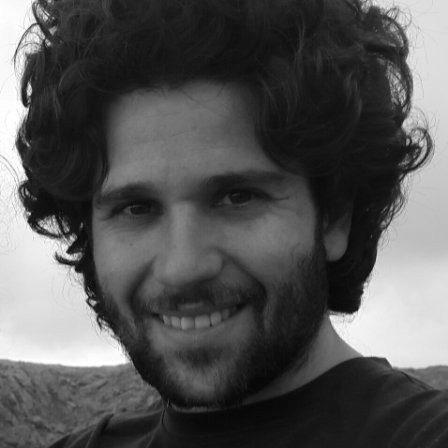
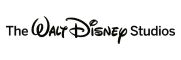