Exploration of useTimeout in ReactJS for managing setTimeout in functional components
ReactJS is a popular JavaScript library for building user interfaces. It provides a declarative and component-based approach to developing interactive web applications. React allows developers to create reusable UI components that efficiently update in response to changes in data or state.
Functional components are a key feature of React. They are JavaScript functions that return JSX (a syntax extension for JavaScript), representing the structure and content of a component. Functional components have become the preferred way of writing React components due to their simplicity, reusability, and compatibility with React hooks.
Introduction to the useTimeout Custom Hook:
The useTimeout custom hook is a specialized tool in React that simplifies the management of timeouts within functional components. It encapsulates the logic of setTimeout and provides an intuitive interface for handling delayed actions.
With the useTimeout hook, developers can schedule the execution of a callback function after a specified delay. The hook handles the timing and synchronization with component rendering, ensuring proper execution even during updates or state changes.
The Need for Managing setTimeout in Functional Components:
Managing setTimeout in functional components becomes necessary when dealing with timed actions, delays, or asynchronous operations. While setTimeout is a built-in JavaScript function, using it directly within functional components can lead to timing issues, memory leaks, and complex code.
By utilizing the useTimeout custom hook, developers can overcome these challenges. It simplifies the management of timeouts, synchronizes with component rendering, handles cleanup and cancellation automatically, and improves code readability and organization.
The useTimeout hook addresses the need for a more controlled and efficient approach to managing setTimeout in functional components, allowing developers to build robust and responsive applications with ease.
Understanding setTimeout and its Limitations:
setTimeout is a built-in JavaScript function that allows you to introduce a delay before executing a specified block of code or a callback function. While it is widely used for various purposes, it has certain limitations that developers should be aware of. Let’s explore some of these limitations:
- Lack of Synchronization: setTimeout operates asynchronously, meaning that it does not synchronize with other code execution. This can lead to timing issues, especially in scenarios where you rely on the completion of setTimeout before executing subsequent code. As a result, there may be inconsistencies in the order of operations or unintended race conditions.
- No Guarantees on Timing: Although you specify a delay duration for setTimeout, it does not guarantee precise timing. The actual execution time may be slightly longer or shorter than the specified delay, depending on various factors such as system load and browser performance. This lack of accuracy can be problematic in applications that require precise timing.
- Difficulty in Managing Cleanup: When using setTimeout directly, it becomes the responsibility of the developer to manage cleanup. If a component unmounts before the timeout completes, it is crucial to cancel the pending timeout to prevent memory leaks. This manual cleanup process can be error-prone and tedious, especially when dealing with multiple timeouts.
- Limited Reset and Control: setTimeout does not provide an easy way to reset or modify the delay once it has been initiated. If you need to change the delay or cancel the timeout altogether, you need to keep track of the timeout ID and manually handle these modifications. This can introduce complexity and make the code less maintainable.
- Difficulty in Testing: When setTimeout is used directly within a component, it can make testing more challenging. Since the timing of setTimeout is asynchronous, it can complicate the process of asserting and verifying expected behavior during unit testing. Mocking and controlling the timing of setTimeout calls becomes more involved.
Despite these limitations, setTimeout is still a valuable tool for introducing delays and executing code after a specified time. However, it’s important to be aware of its shortcomings and consider alternative solutions, such as using custom hooks like useTimeout, which provide a more controlled and reliable approach to managing timeouts in React functional components.
Introducing the useTimeout Custom Hook:
Introducing the useTimeout custom hook, a powerful tool in React for managing timeouts in functional components. The useTimeout hook simplifies the handling of delays and timed actions, providing a clean and intuitive interface.
In React applications, timeouts are often used to introduce delays, schedule animations, control user interactions, or manage asynchronous operations. However, dealing with timeouts directly in functional components can lead to synchronization issues, memory leaks, and complex code.
The useTimeout custom hook addresses these challenges by encapsulating the logic and functionality of setTimeout in a reusable function. It provides a higher-level abstraction that handles timeout management, synchronization with component rendering, cleanup, and cancellation.
Using the useTimeout hook is straightforward. Simply import it into your functional component and define the delay duration and the callback function to be executed after the specified time has elapsed. The hook takes care of scheduling the timeout, executing the callback, and managing cleanup when the component unmounts or when the timeout is reset or canceled.
One of the key advantages of the useTimeout hook is its ability to synchronize with React rendering and state updates. It ensures that timeouts are triggered at the appropriate times, aligning with the component’s lifecycle and preventing timing inconsistencies.
Additionally, the useTimeout hook promotes code reusability and maintainability. By encapsulating timeout logic in a separate hook, you can easily reuse it across multiple components, leading to cleaner and more modular code.
With the useTimeout custom hook, you can enhance the functionality of your React applications by managing timeouts effectively, simplifying code, and improving the overall user experience. It provides a reliable and convenient solution for handling delays and timed actions, empowering you to create more dynamic and responsive applications with ease.
How to Use the useTimeout Hook ?
With a solid understanding of the useTimeout hook, we’ll dive into its usage. We’ll begin by setting up a basic functional component and proceed to import and implement the useTimeout hook. Through practical examples, we’ll demonstrate how the useTimeout hook can be used for delayed rendering of content, delayed state updates, and debouncing user input. These examples will highlight the versatility and usefulness of the useTimeout hook.
- Install the use-timeout-hook package by running the following command in your project directory using npm or Yarn:
npm install use-timeout-hook
- Import the useTimeout hook at the top of your component file:
import { useTimeout } from 'use-timeout-hook';
- Inside your functional component, invoke the useTimeout hook and provide the necessary parameters. The hook takes two main arguments: the callback function to be executed after the timeout, and the delay duration in milliseconds. Here’s an example:
function MyComponent() { const handleTimeout = () => { // Code to be executed after the timeout console.log('Timeout complete!'); }; // Invoking the useTimeout hook with a callback function and a delay of 2000 milliseconds (2 seconds) useTimeout(handleTimeout, 2000); return ( // JSX content of your component ); }
- Customize the callback function and the delay duration based on your requirements. The callback function can perform any actions you need after the specified delay. You can also utilize variables or states within the callback function to create dynamic behavior.
- Render the JSX content of your component as usual within the return statement.
With these steps, the useTimeout hook is successfully integrated into your React functional component. It will manage the timeout and execute the provided callback function after the specified delay. You can customize the behavior and leverage the power of timeouts in your application.
Examples of using the useTimeout hook for delayed actions
Certainly! Here are a few examples of how you can use the useTimeout hook for delayed actions in your functional components:
- Delayed Rendering of Content:
import React, { useState } from 'react';
import { useTimeout } from 'use-timeout-hook';
function DelayedContent() {
const [showContent, setShowContent] = useState(false); useTimeout(() => {
setShowContent(true);
}, 2000);
return (
<div>
{showContent ? <p>Delayed content is now visible!</p> : <p>Loading...</p>}
</div>
);
}
export default DelayedContent;
In this example, the content is initially hidden (showContent is set to false), and after a delay of 2000 milliseconds (2 seconds), the content becomes visible by updating the showContent state to true.
- Delayed State Updates:
import React, { useState } from 'react';
import { useTimeout } from 'use-timeout-hook';
function DelayedStateUpdate() {
const [message, setMessage] = useState('');
useTimeout(() => {
setMessage('Delayed state update!');
}, 3000);
return <p>{message}</p>;
}
export default DelayedStateUpdate;
In this example, the initial state of the message variable is empty. After a delay of 3000 milliseconds (3 seconds), the useTimeout hook updates the state by setting the message to ‘Delayed state update!’. The updated state triggers a re-render, and the new message is displayed in the component.
- Debouncing User Input:
import React, { useState } from 'react';
import { useTimeout } from 'use-timeout-hook';
function DebouncedInput() {
const [inputValue, setInputValue] = useState('');
const [debouncedValue, setDebouncedValue] = useState('');
const handleInputChange = (event) => {
const value = event.target.value;
setInputValue(value);
// Debounce the input changes by updating the debounced value after a delay of 500 milliseconds
useTimeout(() => {
setDebouncedValue(value);
}, 500);
};
return (
<div>
<input type="text" value={inputValue} onChange={handleInputChange} />
<p>Debounced Value: {debouncedValue}</p>
</div>
);
}
export default DebouncedInput;
In this example, the useTimeout hook is used to debounce user input. As the user types in the input field, the input value is updated in real-time (inputValue state). However, the debouncedValue state is updated with a delay of 500 milliseconds using the useTimeout hook. This helps to reduce unnecessary updates and provides a debounced value that reflects the input after a short delay.
These examples demonstrate how the useTimeout hook can be used to introduce delays and manage delayed actions in various scenarios. You can customize the delay durations and the actions performed within the timeout callback to suit your specific requirements.
Advanced features of the useTimeout hook
The useTimeout hook provides several advanced features that enhance its flexibility and functionality when managing timeouts in React functional components. Some of these advanced features include:
- Custom Delay Duration and Callback Function: The useTimeout hook allows you to specify the delay duration and the callback function as parameters. You can customize the delay duration dynamically based on component state or other variables. Additionally, you can provide a callback function that executes after the specified delay. This feature enables you to have fine-grained control over the timing and actions performed when the timeout completes.
- Timeout Reset and Cancellation: The useTimeout hook provides functions to reset and cancel the timeout. The reset function allows you to restart the timeout, effectively extending the delay duration. This can be useful when you want to reset the countdown or delay based on certain events or user interactions. On the other hand, the clear function allows you to cancel the timeout altogether, preventing its execution. This flexibility gives you the ability to control the timing and lifecycle of the timeout as needed.
- Cleanup and Component Unmounting: The useTimeout hook takes care of automatic cleanup when the component unmounts. If a timeout is pending and the component is about to be unmounted, the hook automatically cancels the timeout to prevent memory leaks and unexpected behavior. This ensures the proper cleanup of resources associated with the timeout and promotes the stability and reliability of your application.
- Callback with Previous State and Props: The useTimeout hook offers an optional third parameter that allows you to access the previous state and props within the callback function. By providing an array of dependencies as the third parameter, the hook will execute the callback with the previous state and props values as arguments. This feature can be useful when you need to perform computations or comparisons based on the previous state or props values.
These advanced features of the useTimeout hook empower you to customize the behavior of timeouts within your React functional components. You can adjust the delay duration, provide custom callback functions, reset or cancel timeouts, and ensure proper cleanup during component unmounting. These capabilities enhance the control and flexibility of managing timeouts, enabling you to create more robust and dynamic React applications.
Best practices and tips for using the useTimeout hook
When using the useTimeout hook in your React functional components, it’s helpful to follow some best practices and utilize certain tips to ensure efficient and effective usage. Here are some recommended best practices and tips:
Use useCallback for the Timeout Callback: To optimize performance and prevent unnecessary re-renders, use the useCallback hook to memorize the timeout callback function. This ensures that the callback is only created once, even if the component re-renders. For example:
const handleTimeout = useCallback(() => {
// Timeout callback logic
}, []);
Leverage useRef for Mutable Values: If you need to access mutable values within the timeout callback or other parts of your component, consider using the useRef hook. useRef provides a way to maintain mutable references without triggering re-renders. This can be useful for managing flags or other variables related to the timeout. For example:
const timeoutRef = useRef(null);
Optimize Dependencies: When using the useTimeout hook, be mindful of the dependencies array passed as the third parameter. Ensure that you include all necessary dependencies that may cause the timeout to be recalculated or re-triggered. This helps avoid unnecessary recalculations and improves performance. For example:
useTimeout(callback, delay, [dependency1, dependency2]);
Use clearTimeout for Cleanup: When performing cleanup within your component, make sure to use clearTimeout explicitly if needed. The useTimeout hook internally uses setTimeout, so it’s essential to cancel any pending timeouts to prevent memory leaks. For example, you can use a useEffect hook to cancel the timeout on component unmount:
useEffect(() => {
return () => {
clearTimeout(timeoutRef.current);
};
}, []);
- Combine with Other Hooks: The useTimeout hook can be combined with other React hooks to enhance functionality. For instance, you can use it in conjunction with useState to manage state updates after a delay or with useEffect to trigger side effects after the timeout completes.
- Consider Debouncing and Throttling: The useTimeout hook can be useful for implementing debouncing or throttling behavior by delaying the execution of a function. This can be particularly helpful in scenarios where you want to limit the rate of function calls based on user input or other events.
By following these best practices and tips, you can maximize the benefits of the useTimeout hook and ensure clean, efficient, and performant code when managing timeouts in your React functional components.
Real-world examples and use cases
The useTimeout hook in React can be applied to various real-world examples and use cases. Here are a few practical scenarios where the useTimeout hook can be beneficial:
Toast Notifications:
In a web application, you might want to display toast notifications that automatically disappear after a certain duration. The useTimeout hook can be used to manage the timeout for hiding the toast notification. After the specified delay, the hook can update the state to hide the notification, providing a smooth and automated user experience.
Debounced Search:
Implementing a search functionality with a debounce effect can improve performance by reducing the number of API requests or function invocations. The useTimeout hook can be utilized to introduce a delay before executing the search logic after the user has finished typing. By resetting the timeout on subsequent keystrokes, the search action is only triggered once the user pauses typing, providing more efficient search functionality.
Delayed Animations or Transitions:
When working with animations or transitions, you may want to delay their execution for a smoother visual effect. The useTimeout hook can be used to introduce a delay before triggering the animation or transition, allowing for a controlled and timed visual experience. This can be useful for creating loading spinners, fade-in effects, or other visually appealing UI interactions.
Auto-Saving Drafts:
In an application that supports drafting content (such as emails, blog posts, or form submissions), you can use the useTimeout hook to automatically save drafts after a period of inactivity. By resetting the timeout on every user action, the draft-saving action is delayed until the user pauses their input, reducing unnecessary saves and improving the overall user experience.
Feedback Messages:
Providing feedback messages to users after completing an action (e.g., submitting a form) can be enhanced with the useTimeout hook. After performing the action, you can set a timeout to display a success message or an error message. The useTimeout hook helps manage the timing of showing and hiding these feedback messages, providing a clear and temporary visual indication to the user.
These examples demonstrate how the useTimeout hook can be applied to real-world scenarios to manage delays, improve user experiences, and enhance the functionality of React applications. By leveraging the hook’s capabilities, you can introduce timed actions, automate processes, and create more interactive and responsive user interfaces.
Conclusion
In conclusion, managing setTimeout in functional components is made significantly easier and more efficient with the useTimeout hook in ReactJS. We’ve explored its features, usage patterns, and best practices. By leveraging this custom hook, you can handle delays seamlessly, enhance user experiences, and write cleaner, more maintainable code. Empower yourself with the useTimeout hook and elevate your React development skills.
Table of Contents
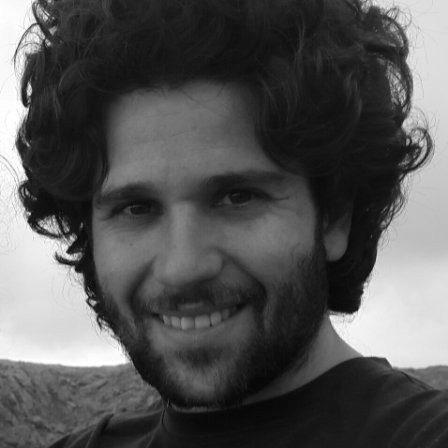
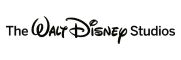