Unleashing the Power of ReactJS useTransition: Building Seamless Animations and Transitions
-
Introduction
ReactJS useTransition is a powerful tool that enables developers to create smooth animations and transitions in their React applications. In this blog, we will explore the ins and outs of useTransition and discover how it can enhance the user experience. We will cover its benefits, installation, implementation, and advanced techniques. So let’s dive in and unleash the potential of ReactJS useTransition!
1.1 What is ReactJS useTransition?
ReactJS useTransition is a React Hook that allows for managing asynchronous state transitions, particularly useful for animations and loading states. It allows you to defer rendering updates and control the timing of component updates, resulting in smooth and uninterrupted user experiences.
1.2 Benefits of using useTransition
By using useTransition, you can achieve several benefits, including improved user perception, reduced jank, and better performance. It helps in creating delightful animations and transitions, enhancing the overall user interface and interactivity of your React applications.
1.3 Overview of the blog content
In this blog, we will explore the various aspects of ReactJS useTransition. We will start by getting started with useTransition, understanding the transition state, and creating smooth animations. We will also discuss optimizing transitions for performance, advanced techniques, best practices, and conclude with a summary and encouragement for further exploration.
-
Getting Started with useTransition
2.1 Installing ReactJS and setting up a new project
Before diving into useTransition, you need to have ReactJS installed and a new project set up. If you haven’t done this already, follow these steps:
# Install ReactJS using npm npm install react # Create a new React project using Create React App npx create-react-app my-app cd my-app # Start the development server npm start
2.2 Importing useTransition and setting up a basic component
Once you have a React project set up, you can import useTransition and start using it in your components. Here’s an example of how you can import it and set up a basic component:
import React, { useState, useTransition } from 'react'; const MyComponent = () => { const [isVisible, setIsVisible] = useState(false); const [startTransition, isPending] = useTransition(); const handleClick = () => { startTransition(() => { setIsVisible(!isVisible); }); }; return ( <div> <button onClick={handleClick}>Toggle Visibility</button> {isPending && <span>Loading...</span>} {isVisible && <div>Content to be animated</div>} </div> ); };
Understanding the Transition State
2.2.1 Explaining the transition state concept
The transition state in ReactJS useTransition allows you to control the timing of rendering updates during state transitions. When you initiate a transition using startTransition, React enters a pending state, deferring rendering updates until the transition is complete. This helps in avoiding interruptions and jank during animations or loading states.
2.2.2 Defining concurrent mode and how it affects transitions
ReactJS useTransition works hand in hand with concurrent mode, a new feature introduced in React 18. Concurrent mode enables React to work on multiple tasks concurrently, prioritizing user interactions and ensuring smooth transitions even when other tasks are being performed. This ensures that your animations and transitions remain responsive and visually appealing.
2.2.3 Code example:
Implementing a transition state using useTransition
Let’s take a look at an example to see how the transition state works:
import React, { useState, useTransition } from 'react'; const MyComponent = () => { const [isVisible, setIsVisible] = useState(false); const [startTransition, isPending] = useTransition(); const handleClick = () => { startTransition(() => { setIsVisible(!isVisible); }); }; return ( <div> <button onClick={handleClick}>Toggle Visibility</button> {isPending && <span>Loading...</span>} {isVisible && <div>Content to be animated</div>} </div> ); };
In this example, when the button is clicked, a transition is initiated by calling startTransition. The state update to toggle visibility of the content is wrapped inside startTransition. While the transition is pending, the loading message is displayed using {isPending && <span>Loading…</span>}. Once the transition completes, the content is either rendered or hidden based on the isVisible state.
-
Creating Smooth Animations with useTransition
3.1 Understanding animation interpolation
To create smooth animations using ReactJS useTransition, it’s crucial to understand animation interpolation. Interpolation allows you to smoothly transition between different states of an animation by calculating intermediate values. This ensures that your animations appear fluid and natural to the user.
3.2 Configuring the transition options
ReactJS useTransition provides various options to configure your transitions. Some common options include timeoutMs to set the duration of the transition, easing to define the animation curve, and delayMs to introduce a delay before the transition starts. Experimenting with these options can help you achieve the desired animation effects.
3.3 Code example:
Creating smooth fade-in and fade-out animations
Let’s explore a code example that demonstrates a fade-in and fade-out animation using useTransition:
import React, { useState, useTransition } from 'react'; const FadeAnimation = () => { const [isVisible, setIsVisible] = useState(false); const [startTransition, isPending] = useTransition({ timeoutMs: 500, }); const handleClick = () => { startTransition(() => { setIsVisible(!isVisible); }); }; return ( <div> <button onClick={handleClick}>Toggle Visibility</button> {isPending && <span>Loading...</span>} {isVisible && ( <div style={{ opacity: isPending ? 0.5 : 1, transition: 'opacity 0.5s', }} > Content with fade-in and fade-out animation </div> )} </div> ); };
In this example, when the button is clicked, the transition is initiated using startTransition, and the visibility state is toggled. The opacity of the content is adjusted based on the isPending value, which provides a fade-in effect when the content appears and a fade-out effect when it disappears. The transition property ensures that the opacity change is animated over a 0.5-second duration.
Optimizing Transitions for Performance
3.3.1 Identifying performance bottlenecks in transitions
While ReactJS useTransition provides a smooth transition experience, it’s important to consider performance optimizations. In complex applications with numerous animations, excessive re-renders or inefficient rendering updates can lead to degraded performance. Identify potential performance bottlenecks in your transitions and take appropriate measures to optimize them.
3.3.2 Using the Suspense component to optimize loading states
The Suspense component is another powerful tool provided by ReactJS for optimizing loading states during transitions. By wrapping your async components or code with Suspense, you can handle loading states more efficiently, providing a seamless user experience. This can be particularly useful when fetching data or loading heavy components.
3.3.3 Code example:
Optimizing image loading transitions with Suspense
Let’s consider an example where we optimize image loading transitions using the Suspense component:
import React, { Suspense, useState, useTransition } from 'react'; const ImageComponent = React.lazy(() => import('./ImageComponent')); const ImageLoader = () => { const [isPending, startTransition] = useTransition(); const [isVisible, setIsVisible] = useState(false); const handleClick = () => { startTransition(() => { setIsVisible(!isVisible); }); }; return ( <div> <button onClick={handleClick}>Toggle Visibility</button> <Suspense fallback={<span>Loading...</span>}> {isVisible && <ImageComponent />} </Suspense> {isPending && <span>Loading image...</span>} </div> ); };
In this example, we have an ImageLoader component that toggles the visibility of an ImageComponent. We wrap the ImageComponent with the Suspense component, providing a fallback loading message using the fallback prop. This ensures that during the transition, if the image component is not yet loaded, the loading message is displayed.
-
Advanced Techniques with useTransition
4.1 Implementing complex transitions with multiple stages
ReactJS useTransition allows for implementing complex transitions with multiple stages. You can create sophisticated animations by defining different states and triggering transitions between them. This enables you to build rich and interactive user interfaces.
4.2 Using useTransition with third-party animation libraries
While ReactJS useTransition provides built-in capabilities for creating animations and transitions, you can also integrate it with third-party animation libraries for more advanced effects. Libraries like Framer Motion or React Spring can be used in conjunction with useTransition to achieve even more dynamic and expressive animations.
4.3 Code example:
Building a sliding carousel with useTransition and Framer Motion
Let’s explore an example where we combine useTransition with the Framer Motion library to build a sliding carousel:
import React, { useState, useTransition } from 'react'; import { motion } from 'framer-motion'; const Carousel = () => { const [index, setIndex] = useState(0); const [startTransition, isPending] = useTransition(); const nextSlide = () => { startTransition(() => { setIndex((prevIndex) => (prevIndex + 1) % slides.length); }); }; const prevSlide = () => { startTransition(() => { setIndex((prevIndex) => (prevIndex - 1 + slides.length) % slides.length); }); }; return ( <div> <motion.div initial={{ opacity: 0 }} animate={{ opacity: 1 }} exit={{ opacity: 0 }} > <img src={slides[index]} alt="Carousel Slide" /> </motion.div> <button onClick={prevSlide}>Previous</button> <button onClick={nextSlide}>Next</button> {isPending && <span>Loading...</span>} </div> ); };
In this example, we use the Framer Motion library in combination with useTransition to create a sliding carousel. The carousel slides are represented by an array of image URLs. When the “Next” or “Previous” button is clicked, a transition is initiated, and the index of the current slide is updated accordingly. The transition effect is achieved by using the motion.div component from Framer Motion, which animates the opacity of the image during the transition.
-
Best Practices and Tips
5.1 Keeping transitions simple and intuitive
When using ReactJS useTransition, it’s important to keep your transitions simple and intuitive. Avoid overly complex animations that might confuse or distract users. Aim for smooth and subtle transitions that enhance the user experience without overwhelming the interface.
5.2 Avoiding unnecessary re-renders during transitions
To ensure optimal performance, it’s crucial to avoid unnecessary re-renders during transitions. Evaluate your component dependencies and use techniques like memoization or shouldComponentUpdate to prevent unnecessary updates and re-renders. This helps in maintaining smooth and efficient transitions.
5.3 Handling error cases gracefully during transitions
During transitions, it’s essential to handle error cases gracefully. If an error occurs while transitioning, provide clear and informative error messages to the user. Consider using error boundaries or error handling techniques to ensure a smooth transition flow even in the presence of errors.
-
Conclusion
6.1 Summary of the blog content
In this blog, we explored the powerful capabilities of ReactJS useTransition for building smooth animations and transitions in React applications. We covered the basics of useTransition, its benefits, and how to get started. We dived into advanced techniques such as complex transitions and integrating third-party animation libraries. Additionally, we discussed best practices for keeping transitions simple and performant, as well as gracefully handling error cases.
6.2 Encouragement to explore more useTransition possibilities
ReactJS useTransition opens up a world of possibilities for creating engaging and dynamic user interfaces. As you continue your journey with React, I encourage you to experiment with different transition effects, explore more advanced techniques, and leverage the rich ecosystem of animation libraries available. With useTransition, you have the power to bring your React applications to life with smooth animations and delightful user experiences.
Table of Contents
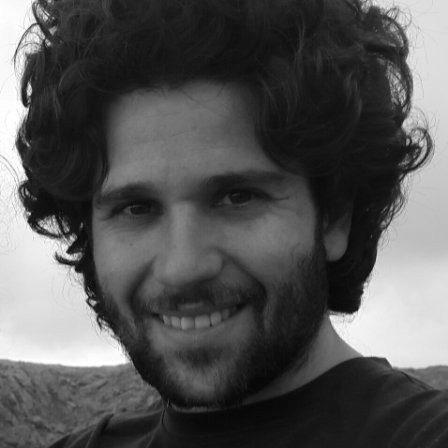
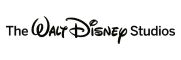