Enabling Two-way Communication in React Components with useWebsocket
ReactJS is an excellent tool for building dynamic and user-friendly web applications. Its power and versatility are some of the reasons to hire ReactJS developers for your projects. The framework becomes even more impressive when combined with real-time communication functionalities. One way of achieving this real-time communication is using WebSockets, a protocol that enables two-way communication between a client and a server. Whether you’re a seasoned developer or someone looking to hire ReactJS developers, this article will guide you on how to integrate WebSockets into your ReactJS components using the `useWebsocket` custom hook.
What are WebSockets?
WebSockets allow for a persistent connection between a client and a server, with both parties being able to start sending data at any time. This is a notable difference from the traditional HTTP protocol where communication is initiated by the client, and the server responds to that specific request. In a WebSocket connection, the server can send data to the client at any moment, and vice versa, making it an excellent tool for real-time applications.
The useWebsocket Custom Hook
Before we dive into the useWebsocket custom hook, it’s important to understand what a custom hook in React is. Custom hooks are a feature introduced in React 16.8 that allow you to extract component logic into reusable functions. `useWebsocket` is a custom hook that encapsulates the WebSocket logic, allowing you to easily integrate real-time communication in your components.
Now, let’s explore a simple implementation of this hook:
```jsx import { useState, useEffect } from 'react'; function useWebsocket(url) { const [socket, setSocket] = useState(null); useEffect(() => { const websocket = new WebSocket(url); setSocket(websocket); return () => { websocket.close(); }; }, [url]); return socket; } ```
The `useWebsocket` custom hook accepts a `url` parameter to establish a WebSocket connection and returns the `socket` object. The `useEffect` hook ensures the WebSocket connection is established when the component mounts and closed when the component unmounts.
UseWebsocket in Action
Let’s illustrate the `useWebsocket` hook usage with a simple chat application component:
```jsx import React, { useState, useEffect } from 'react'; import useWebsocket from './useWebsocket'; function ChatComponent() { const [messages, setMessages] = useState([]); const socket = useWebsocket('ws://localhost:8000'); useEffect(() => { if (socket == null) return; socket.onmessage = (event) => { setMessages((prevMessages) => [...prevMessages, event.data]); }; }, [socket]); return ( <div> {messages.map((message, i) => <p key={i}>{message}</p>)} </div> ); } export default ChatComponent; ```
In this component, we’re using the `useWebsocket` hook to establish a connection to a local WebSocket server. The `socket.onmessage` listener inside the `useEffect` hook listens for any new messages sent by the server and appends them to the `messages` state.
Handling Connection Issues
WebSocket connections may sometimes fail due to network issues. To handle this, we can enhance our `useWebsocket` hook to include connection status:
```jsx import { useState, useEffect } from 'react'; function useWebsocket(url) { const [socket, setSocket] = useState(null); const [status, setStatus] = useState('DISCONNECTED'); useEffect(() => { const websocket = new WebSocket(url); websocket.onopen = () => setStatus('CONNECTED'); websocket.onerror = () => setStatus('ERROR'); websocket.onclose = () => setStatus('DISCONNECTED'); setSocket(websocket); return () => { websocket.close(); }; }, [url]); return [socket, status]; } ```
Now, the `useWebsocket` hook also returns the connection `status`. This can be used in your components to provide feedback to the user about the connection status:
```jsx function ChatComponent() { const [messages, setMessages] = useState([]); const [socket, status] = useWebsocket('ws://localhost:8000'); useEffect(() => { if (socket == null) return; socket.onmessage = (event) => { setMessages((prevMessages) => [...prevMessages, event.data]); }; }, [socket]); return ( <div> <p>Status: {status}</p> {messages.map((message, i) => <p key={i}>{message}</p>)} </div> ); } ```
Sending Messages
Finally, to send messages to the server, we can create a `sendMessage` function:
```jsx function ChatComponent() { const [messages, setMessages] = useState([]); const [messageInput, setMessageInput] = useState(''); const [socket, status] = useWebsocket('ws://localhost:8000'); const sendMessage = () => { if (socket == null) return; socket.send(messageInput); setMessageInput(''); }; useEffect(() => { if (socket == null) return; socket.onmessage = (event) => { setMessages((prevMessages) => [...prevMessages, event.data]); }; }, [socket]); return ( <div> <p>Status: {status}</p> {messages.map((message, i) => <p key={i}>{message}</p>)} <input value={messageInput} onChange={e => setMessageInput(e.target.value)} /> <button onClick={sendMessage}>Send</button> </div> ); } ```
With this, we have a fully functional real-time chat component in ReactJS using the `useWebsocket` custom hook.
Conclusion
The `useWebsocket` custom hook brings real-time, bi-directional communication to web applications. This tool, integrated into React components, is a solid reason to hire ReactJS developers. It encapsulates complex WebSocket logic into a cleaner, maintainable structure, adding to the appeal of hiring expert ReactJS developers. The simplified implementation provided in this article offers an introduction to building real-time communication into your applications, a must-have knowledge for those looking to hire ReactJS developers. Embrace the power of hooks in React, like `useWebsocket`, to achieve intuitive, reusable components – an excellent insight for businesses aiming to hire ReactJS developers. Master real-time coding with ReactJS!
Table of Contents
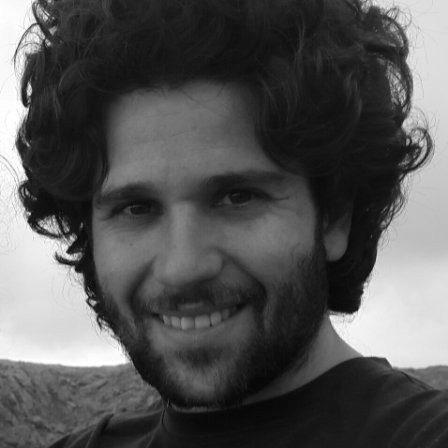
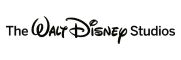