Building Responsive React Components with useWindowSize Hook
In today’s digital landscape, creating responsive web applications is crucial to deliver a seamless user experience across different devices. ReactJS, a popular JavaScript library, offers various tools and hooks to build responsive components. One such hook is the useWindowSize hook, which allows developers to access and utilize the window size in their React components. In this blog, we will explore the useWindowSize hook and learn how to build responsive components based on the window size in ReactJS.
Understanding the Importance of Responsive Components:
Responsive components adapt their layout and behavior based on the available screen space, ensuring optimal user experience across different devices and screen sizes. By leveraging the useWindowSize hook in ReactJS, developers can dynamically adjust component styles and functionalities based on the window dimensions. This enables the creation of versatile and flexible user interfaces that automatically adapt to varying screen sizes.
How to Use the useWindowSize Hook:
Installing and Importing the useWindowSize Hook:
To use the useWindowSize hook in your React project, you need to install it first. Open your terminal and navigate to your project directory. Then, run the following command to install the hook:
npm install react-use-window-size
After successful installation, import the hook into your component file:
import { useWindowSize } from 'react-use-window-size';
Accessing Window Size with the useWindowSize Hook:
Now that we have the useWindowSize hook installed and imported, we can utilize it in our React components. The useWindowSize hook returns an object with the current window width and height. Let’s see an example of how to access the window size using the hook:
import { useWindowSize } from 'react-use-window-size'; const MyComponent = () => { const { width, height } = useWindowSize(); // Use the width and height values in your component logic // ... return ( <div> <p>Window width: {width}</p> <p>Window height: {height}</p> </div> ); };
Implementing Responsive Behavior with useWindowSize:
Once we have access to the window size using the useWindowSize hook, we can implement responsive behavior in our components. For example, let’s say we want to change the background color of a component when the window width is less than 600 pixels. We can achieve this using conditional rendering:
import { useWindowSize } from 'react-use-window-size'; const MyComponent = () => { const { width } = useWindowSize(); const isMobile = width < 600; return ( <div style={{ backgroundColor: isMobile ? 'red' : 'blue' }}> <p>Responsive Component</p> </div> ); };
Building Responsive Components:
Creating a Responsive Component:
To build a responsive component, we need to consider the desired behavior based on different screen sizes. For instance, we might want to show or hide certain elements, change the layout, or adjust the styling. Let’s create a simple example of a responsive component that displays different content based on the window width:
import { useWindowSize } from 'react-use-window-size'; const ResponsiveComponent = () => { const { width } = useWindowSize(); return ( <div> {width < 600 ? ( <p>Mobile Content</p> ) : ( <div> <h2>Desktop Content</h2> <p>This is the content for larger screens.</p> </div> )} </div> ); };
Using Window Size to Adapt Component Layout:
In addition to content changes, we can use the window size to adapt the layout of our components. For example, we might want to change the number of columns in a grid layout based on the available screen space. Here’s an example of using the window size to determine the number of columns in a CSS grid layout:
import { useWindowSize } from 'react-use-window-size'; const GridComponent = () => { const { width } = useWindowSize(); const numColumns = width < 800 ? 2 : 4; return ( <div style={{ display: 'grid', gridTemplateColumns: `repeat(${numColumns}, 1fr)` }}> {/* Grid items */} </div> ); };
Applying Media Queries with useWindowSize:
The useWindowSize hook can also be used in conjunction with CSS media queries to apply responsive styles. We can dynamically add or remove CSS classes based on the window size. Here’s an example of applying media queries using the useWindowSize hook:
import { useWindowSize } from 'react-use-window-size'; import './MyComponent.css'; const MyComponent = () => { const { width } = useWindowSize(); const isMobile = width < 600; return ( <div className={`my-component ${isMobile ? 'mobile' : 'desktop'}`}> <p>Responsive Component</p> </div> ); };
In addition to content changes and layout adjustments, we can also use the window size to dynamically modify component behavior. For example, we might want to toggle certain functionality or trigger specific actions based on the available screen space. Let’s explore an example of how to achieve this using the useWindowSize hook:
import { useWindowSize } from 'react-use-window-size'; const ToggleComponent = () => { const { width } = useWindowSize(); const isMobile = width < 600; const [isActive, setIsActive] = useState(false); const handleToggle = () => { setIsActive(!isActive); }; useEffect(() => { // Perform specific actions based on the window size if (isMobile) { // Do something for mobile screens } else { // Do something for larger screens } }, [isMobile]); return ( <div> <button onClick={handleToggle}>{isActive ? 'Deactivate' : 'Activate'}</button> {isActive && <p>Toggleable Content</p>} </div> ); };
In the above example, we use the useWindowSize hook to determine if the component is being rendered on a mobile screen. Then, we use the isActive state variable and handleToggle function to toggle the visibility of the content. Additionally, we utilize the useEffect hook to perform specific actions based on the window size, updating them whenever the isMobile value changes.
Conclusion:
In this blog post, we explored the useWindowSize hook in ReactJS and learned how to build responsive components based on the window size. We saw how to access the window dimensions using the useWindowSize hook and use them to create versatile and adaptive components.
By leveraging the useWindowSize hook, we can dynamically adjust component styles, layouts, behavior, and functionality based on the available screen space. Whether it’s modifying content, adapting layouts, applying media queries, or toggling functionality, the useWindowSize hook provides a powerful tool for building responsive React components.
Remember to consider responsiveness as an integral part of your web development process. With the proliferation of various devices and screen sizes, creating responsive components is essential for delivering a seamless user experience. By embracing the useWindowSize hook and other responsive design techniques, you can ensure that your ReactJS applications cater to a diverse range of users and devices.
As you continue your journey with ReactJS, keep exploring the vast ecosystem of hooks, libraries, and techniques available to enhance your development process. Experiment with different responsive strategies, test your components on various devices, and always strive to create user-friendly and adaptive interfaces.
Happy coding, and may your ReactJS applications be truly responsive and enjoyable for your users!
Table of Contents
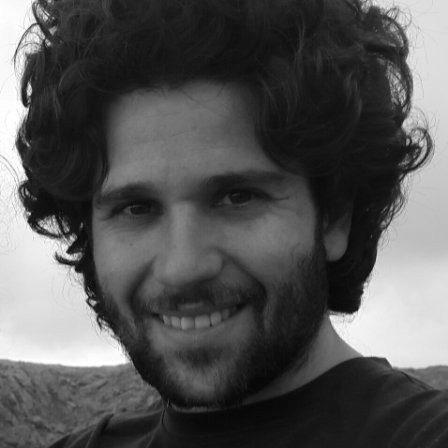
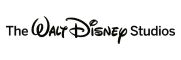