Unleashing Performance Potential: Integrating Web Workers into Functional Components using ReactJS’s useWorker Hook
This introduction presents the concept of ReactJS’s useWorker, a custom hook that allows easy integration of Web Workers into functional components, enhancing application performance. Web Workers enable multi-threading in JavaScript, handling heavy computations without blocking the UI. This post aims to provide a comprehensive guide to understanding useWorker, its integration with Web Workers, and its application in ReactJS functional components. It will cover setting up the environment, basic and advanced usage, debugging, and real-world applications. This guide is beneficial for beginners exploring ReactJS and experienced developers looking to optimize their applications.
What are Web Workers
Web Workers are a JavaScript feature enabling background script execution, separate from a web application’s main thread, which improves performance and prevents UI blocking during heavy computations or data processing. Introduced in HTML5, there are two types of Web Workers: Dedicated Workers, tied to their creators, and Shared Workers, which can be used by multiple scripts from various windows, IFrames, or even domains. Although they enhance performance, concurrency, and responsiveness, Web Workers lack access to the DOM or certain web APIs due to their isolated context. Communication with the main thread happens through a messaging system. In ReactJS, the useWorker hook simplifies the integration and management of Web Workers within functional components.
The concept of ReactJS useWorker
ReactJS’s useWorker is a custom hook that simplifies the incorporation of Web Workers into functional components, thereby enhancing efficiency and performance. It handles the complexities of creating and managing Web Workers, allowing developers to concentrate on application functionality. UseWorker works by returning a pair [workerStatus, workerFunction] upon initialization. The worker function performs expensive or time-consuming tasks on a different thread, and worker status gives information about the function’s status, which can be used to manage the UI. The simplicity of useWorker eliminates the need for developers to understand the complexities of Web Workers. This hook handles the creation, messaging, and cleanup of the worker, making it a significant contributor to the performance optimization of ReactJS applications by ensuring the main thread remains responsive to user interactions.
Setting up the ReactJS environment for useWorker
To utilize the useWorker custom hook in your ReactJS environment, you’ll need to follow a few preliminary steps. This guide assumes that you have Node.js and npm installed on your system. If not, download and install them first. Here’s a step-by-step guide to setting up your environment.
- Create a new ReactJS project: If you don’t already have a project to work on, you can create one using Create React App. Open your terminal and run the following command:
npx create-react-app useworker-demo
- Navigate to your project directory: Use the following command to navigate to your new project:
cd useworker-demo
- Install useWorker: Now that you’re inside your project folder, it’s time to install useWorker. Run the following command:
cd useworker-demo
- Create a Web Worker Function: Inside your `src` folder, create a new file called `workerFunction.js`. This is where you will define the functions you wish to run in the web worker.
- Import useWorker: In the component where you wish to utilize the web worker, import the useWorker function like this:
import { useWorker } from 'react-use-worker';
- Initialize useWorker: In the same component, inside the functional component body, you can now initialize useWorker. Pass the worker function that you defined in `workerFunction.js` as an argument.
- Run the application: Finally, you can run your ReactJS application by using the following command:
npm start
By following these steps, you’ll be able to set up your ReactJS environment to effectively use the useWorker custom hook, thereby integrating Web Workers in your functional components. The subsequent sections will detail how to properly use useWorker in your ReactJS components.
Basic usage of useWorker in ReactJS
After setting up the environment for useWorker, let’s discuss how you can leverage it in your ReactJS functional components.
For the sake of this explanation, assume we have a computationally intensive task, such as finding the nth Fibonacci number, that we want to offload to a web worker.
- Creating a worker function: Create a new file `workerFunction.js` in your `src` directory and define the function.
export const fibonacci = (num) => { let a = 1, b = 0, temp; while (num >= 0){ temp = a; a = a + b; b = temp; num--; } return b; }
2. Using useWorker in your component: Inside the functional component body, import and initialize useWorker.
import React from 'react'; import { useWorker } from 'react-use-worker'; import { fibonacci } from './workerFunction'; const MyComponent = () => { const [workerStatus, runFibonacci] = useWorker(fibonacci); const handleClick = async () => { const result = await runFibonacci(40); // any large number console.log(result); } return ( <div> <button onClick={handleClick} disabled={workerStatus === 'RUNNING'}> Calculate Fibonacci </button> {workerStatus === 'RUNNING' && <p>Calculating...</p>} </div> ); } export default MyComponent;
In this example, we’re passing the `fibonacci` function to `useWorker`, which returns the current worker status and a function to run the task (`runFibonacci`).
When the button is clicked, `handleClick` is triggered, which in turn triggers `runFibonacci` with the number as an argument. This will start executing the Fibonacci function in a web worker, not blocking the main thread.
The `workerStatus` can be used to manage the UI based on the worker function’s status. Here, the button is disabled and a “Calculating…” message is shown while the Fibonacci function is running.
That’s the basic usage of the `useWorker` hook in ReactJS. In the next section, we will discuss some advanced usage of `useWorker` including error handling, dependency management, and using with other hooks like `useEffect`.
Advanced usage of useWorker in ReactJS
Let’s move on to some of the advanced concepts of using the useWorker hook in ReactJS.
- Error Handling: The useWorker hook makes it easy to handle any errors that might occur within the worker function. When you call the function returned by useWorker, it returns a Promise. If an error is thrown within the worker function, the Promise will be rejected with that error.
Here’s an example:
const handleClick = async () => { try { const result = await runFibonacci(40); // any large number console.log(result); } catch (err) { console.error(err); } }
In this example, if an error occurs within the Fibonacci function, it will be caught in the catch block, and the error message will be logged to the console.
- Dependency Management: Just like with the useEffect hook, you can provide an array of dependencies to the useWorker hook. When any of these dependencies change, a new Worker will be created.
For example, suppose you have a worker function that depends on some external state or prop. You can ensure the worker is always using the latest value by including it in the dependencies array:
const [workerStatus, runFibonacci] = useWorker(fibonacci, [someProp]);
- Using with useEffect: You can use the useWorker hook in conjunction with other hooks, such as useEffect.
This can be useful when you want to automatically run the worker function when your component mounts or some dependency changes:
useEffect(() => { const fetchData = async () => { const result = await runFibonacci(40); // any large number console.log(result); } fetchData(); }, [runFibonacci]);
Here, the Fibonacci function will run whenever the `runFibonacci` function changes. Because we’re including it in the dependencies array of `useEffect`, this will happen whenever any of the dependencies of `useWorker` change.
By mastering these advanced useWorker techniques, you can ensure optimal performance in your ReactJS applications and handle any complexities associated with background tasks seamlessly.
Debugging and Error Handling
While using the useWorker hook in ReactJS can enhance your application’s performance, you may run into issues that need debugging or errors that need handling. Here are some tips on how you can go about this:
- Debugging Web Workers: Debugging code running inside a Web Worker can be more challenging than debugging regular JavaScript code. However, modern browsers provide tools for this.
For instance, in Chrome DevTools, under the ‘Sources’ tab, there’s a section for Workers where you can find your worker scripts, set breakpoints, step through the code, inspect variables, and so forth.
- Handling Errors: As mentioned in the advanced usage, useWorker returns a Promise when the worker function is called. If an error occurs within the worker function, this Promise will be rejected with that error, and you can catch this error using a try/catch block.
const handleClick = async () => { try { const result = await runFibonacci(40); // any large number console.log(result); } catch (err) { console.error(err); // handle the error accordingly } }
In this example, if an error occurs within the Fibonacci function, it will be caught and logged to the console. From there, you can decide on the best course of action to handle this error.
- Using Worker Status: The useWorker hook provides the status of the worker. This can be helpful for debugging as well. If your worker function is not completing or returning a result, you can check the status of the worker. If it’s stuck on ‘RUNNING’, it might mean that your worker function is not returning or it’s caught in an infinite loop.
- Worker Function Constraints: Keep in mind that worker functions have some limitations. They don’t have access to the DOM or the global scope, and they can’t import modules using import statements. If you’re seeing errors, make sure your worker function is not trying to do something it can’t.
By understanding how to debug and handle errors when using the useWorker hook, you can build more robust React applications that leverage the power of Web Workers.
Case Study: Real-World useWorker Application
This real-world case study showcases the application of the useWorker hook in a photo editing application built with ReactJS. The application faced performance issues due to heavy computation during the application of filters to high-resolution images, causing the main thread to block and the application to become unresponsive. The solution involved offloading these tasks to a separate thread using Web Workers and the useWorker hook. A worker function, `applyFilter`, was created to apply filters to images and integrated into the application with useWorker. Any errors were handled with a try/catch block, and worker status was monitored for debugging. The implementation of useWorker led to significant performance improvement, with image filtering operations now running in the background, resulting in a more responsive user interface. This illustrates the effectiveness of useWorker in offloading heavy tasks in ReactJS applications, enhancing performance and user experience.
Conclusion
In summary, ReactJS’s useWorker hook provides an efficient method for integrating Web Workers into functional components, thereby significantly improving the performance of applications handling heavy computations or lengthy tasks. By transferring these tasks to a separate thread, useWorker ensures a smoother, more responsive application by keeping the main thread free. This blog post delved into Web Workers, the useWorker hook, and its usage within a ReactJS environment, including setup, debugging, error handling, and a practical case study. Mastery of useWorker allows developers to fully utilize Web Workers in their React applications, enhancing performance without the need to manually manage Web Workers. This showcases the flexibility and power offered by the React ecosystem to developers.
Table of Contents
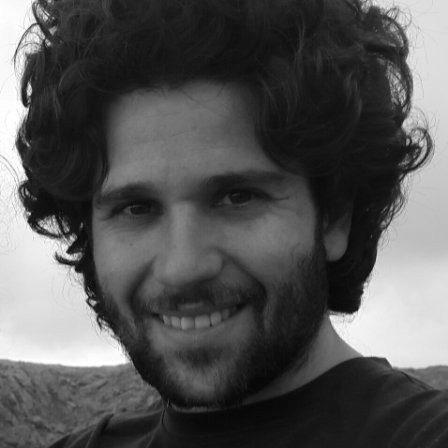
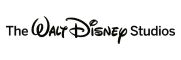