ReactJS and WebSockets: Building Real-time Applications
In today’s fast-paced digital world, real-time applications have become increasingly important. Whether it’s chat applications, live sports updates, or collaborative tools, users expect instant feedback and live data. ReactJS, a popular JavaScript library for building user interfaces, combined with WebSockets, a protocol for real-time communication, offers a powerful solution for developing such applications. This blog explores how to integrate WebSockets with ReactJS to create dynamic, real-time applications.
Understanding WebSockets
WebSockets provide a full-duplex communication channel over a single, long-lived connection. Unlike HTTP, which is request-response-based and stateless, WebSockets allow both the client and server to send data at any time, making them ideal for real-time applications.
How WebSockets Work
1.Connection Establishment: A WebSocket connection starts as an HTTP handshake, which is then upgraded to a WebSocket connection.
- Data Exchange: Once the connection is established, the client and server can exchange messages bidirectionally.
- Connection Termination: Either the client or the server can close the connection when communication is complete.
Setting Up a ReactJS Application
Before diving into WebSocket integration, let’s set up a basic ReactJS application. We’ll use Create React App, a popular boilerplate for React projects.
```bash npx create-react-app real-time-app cd real-time-app npm start ```
This command creates a new ReactJS application and starts the development server. You can see the default React page by navigating to `http://localhost:3000`.
Integrating WebSockets with ReactJS
To integrate WebSockets with React, we’ll use the `WebSocket` API provided by modern browsers. Let’s create a simple component that connects to a WebSocket server and listens for messages.
Creating a WebSocket Component
```jsx import React, { useEffect, useState } from 'react'; const WebSocketComponent = () => { const [messages, setMessages] = useState([]); useEffect(() => { const socket = new WebSocket('ws://localhost:8080'); socket.onmessage = (event) => { setMessages((prevMessages) => [...prevMessages, event.data]); }; return () => socket.close(); }, []); return ( <div> <h2>WebSocket Messages</h2> <ul> {messages.map((message, index) => ( <li key={index}>{message}</li> ))} </ul> </div> ); }; export default WebSocketComponent; ```
Explanation
- State Management: We use the `useState` hook to manage the state of received messages.
- WebSocket Connection: The `useEffect` hook initializes the WebSocket connection when the component mounts. The `onmessage` event handler updates the state with new messages.
- Cleanup: The WebSocket connection is closed when the component unmounts, preventing memory leaks.
Implementing a WebSocket Server
To test our WebSocket client, we’ll need a WebSocket server. For simplicity, we’ll use Node.js with the `ws` library.
Setting Up the Server
First, install the `ws` library:
```bash npm install ws ```
Then, create a simple WebSocket server:
```javascript const WebSocket = require('ws'); const server = new WebSocket.Server({ port: 8080 }); server.on('connection', (socket) => { console.log('Client connected'); socket.send('Welcome to the WebSocket server!'); socket.on('message', (message) => { console.log(`Received: ${message}`); }); socket.on('close', () => { console.log('Client disconnected'); }); }); ```
This server listens for connections on port 8080 and sends a welcome message to each connected client. It also logs messages received from clients.
Real-world Applications
Integrating WebSockets with ReactJS can significantly enhance user experiences in various applications:
Real-time Chat Applications
In chat applications, WebSockets can be used to send and receive messages instantly, providing a seamless experience for users.
Live Data Feeds
For applications like stock trading platforms or sports scoreboards, WebSockets can deliver real-time updates, keeping users informed of the latest changes.
Collaborative Tools
Tools like collaborative text editors or whiteboards can use WebSockets to synchronize changes across multiple users in real-time.
Conclusion
WebSockets and ReactJS together form a powerful combination for building real-time applications. By leveraging WebSockets, developers can create dynamic and responsive user interfaces that react to live data streams. Whether you’re building a chat application, a live data feed, or a collaborative tool, integrating WebSockets with ReactJS can elevate your project to the next level.
Further Reading
Table of Contents
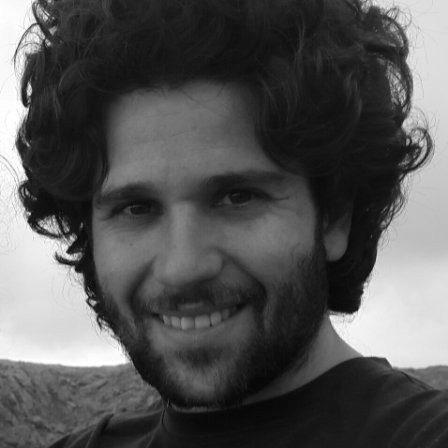
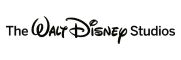