Ruby on Rails Tutorial: Understanding Action Mailer and Email Templates
In the world of web applications, email communication plays a vital role in engaging users and keeping them informed about various events and updates. Ruby on Rails, a powerful and popular web development framework, provides an integrated solution for handling email functionality through Action Mailer. Action Mailer simplifies the process of sending emails from your Rails applications and offers flexibility in customizing email templates to match your branding.
Table of Contents
This tutorial will guide you through the process of setting up Action Mailer, creating email templates, and leveraging its features to send emails effectively. Whether you’re new to Rails or seeking to enhance your understanding of email functionality, this blog will equip you with the necessary knowledge to integrate email communication into your Ruby on Rails applications.
1. Understanding Action Mailer:
1.1. What is Action Mailer?
Action Mailer is a built-in component of Ruby on Rails that enables developers to send emails from their applications effortlessly. It abstracts away the complexities of dealing with email protocols and provides a simple interface for composing and delivering emails. By following the conventions and configuration provided by Action Mailer, you can quickly set up email functionality in your Rails project.
1.2. Advantages of Using Action Mailer:
Action Mailer brings numerous advantages to the table, making it a go-to solution for handling email communication in Rails applications. Some key benefits include:
- Clean Integration: Action Mailer seamlessly integrates with Rails, allowing you to work with emails like any other part of your application.
- Automatic Email Delivery: It takes care of the email delivery process, including handling SMTP settings and ensuring successful delivery.
- Customization Options: Action Mailer offers multiple customization options, such as setting the sender address, email subject, and content type.
- Testing Support: Rails provides built-in support for testing email functionality, making it easier to ensure the correctness of your email templates and deliveries.
1.3. Setting Up Action Mailer in Rails:
Before diving into creating email templates, you need to set up Action Mailer in your Rails project. Fortunately, Rails simplifies this process through its built-in generator. To get started, open your terminal and run the following command:
ruby rails generate mailer Notifier
The above command generates a notifier_mailer.rb file in the app/mailers directory and a corresponding folder containing email templates in app/views/notifier_mailer. This directory structure is where you will define your email templates and the logic for sending emails.
2. Creating Email Templates:
2.1. Default Email Templates:
When you generate a mailer using the Rails generator, it creates default templates for each email action you define. For instance, if you have an email action called welcome_email, Action Mailer will generate the following files:
- welcome_email.html.erb: This is the HTML version of your email template.
- welcome_email.text.erb: This is the plain text version of your email template.
By providing both HTML and plain text versions, Action Mailer ensures that your emails can be properly displayed in different email clients.
2.2. Customizing Email Templates:
While the default email templates are a good starting point, you’ll likely want to customize them to match your application’s branding and style. You can do this by editing the generated template files in the app/views/notifier_mailer directory. Feel free to include your CSS styles, company logo, and other elements to make the emails visually appealing.
2.3. Utilizing HTML and Plain Text Templates:
Action Mailer automatically detects and sends the appropriate email format based on the recipient’s email client. If the client supports HTML emails, it will display the HTML version, and if not, it will fallback to the plain text version. This ensures that your emails reach your users regardless of their email client’s capabilities.
To ensure proper rendering of your email, use well-structured HTML with inline CSS styles. Keep in mind that some email clients may strip out external CSS or have limited support for certain HTML elements, so it’s best to test your templates across different email clients.
2.4. Internationalization of Email Templates:
If your application supports multiple languages, you can leverage Rails’ internationalization (I18n) capabilities to send emails in different languages. This involves setting up translation files for email templates, allowing you to send localized emails based on the recipient’s preferred language. By doing so, you can improve user engagement and make your emails more accessible to a diverse audience.
3. Sending Emails:
3.1. Preparing the Mailer Class:
Before you can send emails, you need to define email actions within the NotifierMailer class generated earlier. Each email action corresponds to a specific email that you want to send. For example, to create a welcome_email, you’ll define a method like this in notifier_mailer.rb:
ruby class NotifierMailer < ApplicationMailer def welcome_email(user) @user = user mail(to: @user.email, subject: 'Welcome to Our Application!') end end
Here, @user is an instance variable that stores the user information needed to personalize the email. The mail method takes care of composing the email with the specified subject and recipient.
3.2. Configuring Email Options:
Action Mailer provides various configuration options that you can set to customize the email behavior. For example, you can define the default sender address for all emails in config/application.rb:
ruby config.action_mailer.default_options = { from: 'noreply@example.com' }
Additionally, you can set the email delivery method and SMTP settings in your environment-specific configuration files (config/environments/development.rb, config/environments/production.rb, etc.):
ruby config.action_mailer.delivery_method = :smtp config.action_mailer.smtp_settings = { address: 'smtp.example.com', port: 587, domain: 'example.com', user_name: 'your_username', password: 'your_password', authentication: 'plain', enable_starttls_auto: true }
3.3. Sending Simple Text Emails:
Sending a simple text email using Action Mailer is straightforward. Let’s modify our welcome_email action to include a simple text message:
ruby class NotifierMailer < ApplicationMailer def welcome_email(user) @user = user mail(to: @user.email, subject: 'Welcome to Our Application!') end end
3.4. Sending HTML Emails with Embedded Images:
To send HTML emails, you can create a corresponding welcome_email.html.erb template in the app/views/notifier_mailer directory. Here’s an example of how you can include an embedded image in your HTML email:
html <!-- app/views/notifier_mailer/welcome_email.html.erb --> <!DOCTYPE html> <html> <head> <title>Welcome to Our Application</title> </head> <body> <h1>Welcome, <%= @user.name %>!</h1> <p>Thank you for joining our application.</p> <%= image_tag('logo.png') %> </body> </html>
In this example, image_tag(‘logo.png’) will embed the logo.png image in your email, provided it exists in the app/assets/images directory.
3.5. Sending Emails with Attachments:
To send emails with attachments, you can use the attachments method within your mailer action. Let’s modify our welcome_email action to include an attachment:
ruby class NotifierMailer < ApplicationMailer def welcome_email(user, attachment) @user = user attachments['document.pdf'] = File.read(attachment.path) mail(to: @user.email, subject: 'Welcome to Our Application!') end end
In the above example, we’re sending a document.pdf file as an attachment. The attachment parameter represents the file you want to attach to the email.
4. Email Interactivity:
4.1. Adding Hyperlinks and Buttons:
To make your emails interactive, you can include hyperlinks and buttons that direct users to specific pages or actions. In your email template, use standard HTML tags to create links and style them using inline CSS:
html <!-- app/views/notifier_mailer/welcome_email.html.erb --> <!DOCTYPE html> <html> <head> <title>Welcome to Our Application</title> </head> <body> <h1>Welcome, <%= @user.name %>!</h1> <p>Thank you for joining our application.</p> <a href="<%= root_url %>">Visit our website</a> <button style="background-color: #007bff; color: #fff; padding: 10px 20px; border: none;">Get Started</button> </body> </html>
4.2. Personalizing Emails with User-Specific Data:
Personalization is key to engaging users. Action Mailer allows you to pass user-specific data to your email templates and use it to customize the content. For instance, you might want to greet users by their names or provide personalized recommendations:
ruby class NotifierMailer < ApplicationMailer def welcome_email(user) @user = user mail(to: @user.email, subject: "Welcome, #{@user.name}!") end end
In this example, we’ve customized the email subject to include the user’s name.
4.3. Handling Email Replies:
Action Mailer also allows you to set up incoming email processing. This enables your application to handle email replies and perform actions based on the content of those emails. To achieve this, you’ll need to configure your mail server to forward incoming emails to your Rails application and set up an incoming email processor using gems like Mailman.
5. Testing Action Mailer:
5.1. Writing Unit Tests for Mailers:
Testing email functionality is crucial to ensure that your emails are being generated and sent correctly. Rails provides built-in support for testing mailers using the ActionMailer::TestCase. You can write unit tests to check whether emails are being generated and sent as expected:
ruby # test/mailers/notifier_mailer_test.rb require 'test_helper' class NotifierMailerTest < ActionMailer::TestCase test "welcome_email sends successfully" do user = users(:one) email = NotifierMailer.welcome_email(user) assert_emails 1 do email.deliver_now end assert_equal [user.email], email.to assert_equal "Welcome, #{user.name}!", email.subject assert_match "Welcome, #{user.name}!", email.body.to_s end end
In this example, we test the welcome_email action to ensure that it sends the correct email to the user.
5.2. Integration Testing for Emails:
Integration tests for emails help verify that your application correctly sends emails in response to user actions. You can use testing libraries like Capybara to simulate user interactions and check if emails are triggered as expected.
5.3. Using Gems for Simplified Testing:
Rails offers several gems that simplify the testing of email functionality. Gems like email_spec provide matchers to verify the content of sent emails easily. These gems enhance the readability of your tests and help you catch potential email-related issues more efficiently.
Conclusion:
Action Mailer is a powerful tool for handling email communication in Ruby on Rails applications. Its seamless integration with Rails, ease of customization, and support for various email formats make it a go-to choice for developers. By understanding how to set up Action Mailer, create email templates, and send personalized emails, you can enhance user engagement and keep your users informed about important events within your application. With proper testing in place, you can ensure that your emails are delivered accurately and efficiently, providing a smooth and interactive experience for your users. Happy coding!
Table of Contents
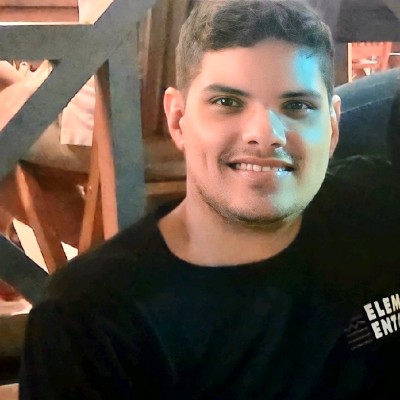
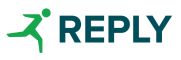