Ruby on Rails Tutorial: Understanding ActionController and Filters
Ruby on Rails is a popular web application framework known for its simplicity, convention over configuration, and rapid development capabilities. At the core of Rails lies ActionController, which handles HTTP requests and renders responses. Additionally, Filters play a crucial role in intercepting and modifying requests and responses, enabling developers to execute pre and post-processing logic. In this tutorial, we will explore ActionController and dive deep into the world of Filters to understand how they enhance the power and flexibility of Ruby on Rails applications.
Table of Contents
1. What is ActionController?
ActionController is a fundamental part of the Rails framework responsible for handling incoming HTTP requests and generating appropriate responses. It forms the bridge between the application and the web server, allowing developers to build robust and interactive web applications efficiently.
2. Key Components of ActionController:
2.1. Actions:
In ActionController, actions represent individual endpoints that handle specific HTTP requests. Each action corresponds to a method within a controller class. When a request is received, ActionController identifies the appropriate action based on the URL and HTTP method, then executes the corresponding method, and finally renders the appropriate response.
ruby class ArticlesController < ApplicationController def index @articles = Article.all end def show @article = Article.find(params[:id]) end end
2.2. Parameters:
Parameters in Rails are the data sent by the client to the server as part of the HTTP request. These parameters can be accessed within actions and filters to perform specific tasks, such as retrieving data from a database or updating a record.
ruby class UsersController < ApplicationController def create @user = User.create(user_params) # ... end private def user_params params.require(:user).permit(:name, :email, :password) end end
2.3. Rendering Views:
After an action has been processed, it usually renders a view that represents the response to the client. Rails conventionally looks for the view template corresponding to the action and renders it, making it easy to present dynamic content to users.
ruby class PagesController < ApplicationController def home # Some logic here render :home end end
2.4. Session Management:
Rails provides built-in support for session management, allowing you to store and retrieve user-specific data throughout their interaction with your application.
ruby class SessionsController < ApplicationController def create user = User.find_by(email: params[:email]) if user&.authenticate(params[:password]) session[:user_id] = user.id redirect_to root_path, notice: 'Logged in successfully!' else flash.now[:alert] = 'Invalid email or password' render :new end end end
3. Understanding Filters in Ruby on Rails:
Filters are an essential part of ActionController as they provide a way to execute code before, after, or around an action. Filters help in centralizing common pre and post-processing tasks, such as authentication, logging, and data manipulation. There are three types of filters in Rails: Before Filters, After Filters, and Around Filters.
3.1. Before Filters:
Before Filters are executed before the designated action and are commonly used for authentication and authorization purposes. They allow developers to ensure that certain conditions are met before processing the requested action.
ruby class AdminController < ApplicationController before_action :authenticate_admin def dashboard # Admin dashboard logic end private def authenticate_admin redirect_to root_path unless current_user.admin? end end
3.2. After Filters:
After Filters are executed after the designated action and are useful for tasks like logging or sending notifications. They are helpful when you want to execute specific code regardless of the action’s outcome.
ruby class OrdersController < ApplicationController after_action :log_order_status def create # Order creation logic end private def log_order_status logger.info("Order ID: #{params[:id]} created successfully.") end end
3.3. Around Filters:
Around Filters wrap the entire action and execute both before and after the action. This type of filter is handy when you need to apply pre and post-processing logic together.
ruby class TransactionsController < ApplicationController around_action :wrap_in_transaction def transfer_funds # Transfer funds logic end private def wrap_in_transaction ActiveRecord::Base.transaction do yield end end end
3.4. Skip and Only Filters:
Rails provides the flexibility to specify filters to be skipped or applied exclusively to specific actions using the skip_before_action, skip_after_action, skip_around_action, only, and except options.
ruby class UsersController < ApplicationController before_action :authenticate_user, except: [:login, :register] before_action :authorize_admin, only: :admin_dashboard # Actions and other code here end
4. Code Samples and Examples:
4.1. Implementing Before Filters:
Consider a scenario where you want to allow only authenticated users to access certain parts of your application. You can achieve this using a Before Filter.
ruby class AdminController < ApplicationController before_action :authenticate_admin def dashboard # Admin dashboard logic end private def authenticate_admin redirect_to root_path unless current_user.admin? end end
4.2. Utilizing After Filters:
Let’s say you want to log the status of an order after it has been successfully created. You can use an After Filter for this purpose.
ruby class OrdersController < ApplicationController after_action :log_order_status def create # Order creation logic end private def log_order_status logger.info("Order ID: #{params[:id]} created successfully.") end end
4.3. Leveraging Around Filters:
Imagine you need to wrap the entire transactional logic for transferring funds between accounts. You can use an Around Filter to encapsulate this functionality.
ruby class TransactionsController < ApplicationController around_action :wrap_in_transaction def transfer_funds # Transfer funds logic end private def wrap_in_transaction ActiveRecord::Base.transaction do yield end end end
4.4. Combining Filters:
You can combine multiple filters to perform various tasks, such as first authenticating the user using a Before Filter, then wrapping the entire action in a transaction using an Around Filter.
ruby class PaymentsController < ApplicationController before_action :authenticate_user around_action :wrap_in_transaction def process_payment # Payment processing logic end private def wrap_in_transaction ActiveRecord::Base.transaction do yield end end end
5. Best Practices for Using ActionController and Filters:
While ActionController and Filters provide a powerful set of tools to handle requests and responses in Rails, it’s essential to follow some best practices:
- Keep actions focused and follow the Single Responsibility Principle (SRP).
- Use filters to handle repetitive tasks and avoid code duplication.
- Utilize skip and only options in filters judiciously to control their application.
- Avoid using Before Filters for actions that don’t need it, as it might impact performance.
- Be cautious with Around Filters, as they may complicate the code and lead to unexpected behavior.
Conclusion
In this tutorial, we explored the fundamental components of ActionController and the versatility of Filters in Ruby on Rails. ActionController plays a pivotal role in handling incoming requests and generating responses, while Filters provide a way to execute code before, after, or around actions, allowing for easy organization and management of common tasks. Understanding how to leverage ActionController and Filters efficiently will empower you to build robust, secure, and high-performing web applications with ease using Ruby on Rails. Happy coding!
Table of Contents
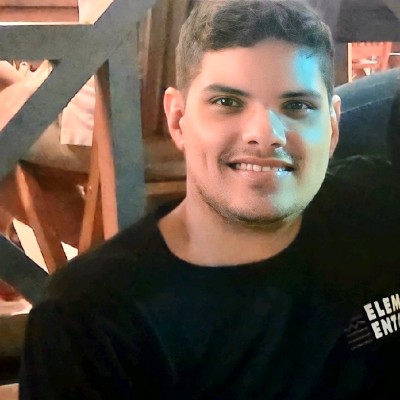
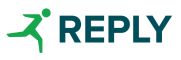