Ruby on Rails Tutorial: Understanding ActionPack and Middleware
Ruby on Rails is a popular web application framework that follows the Model-View-Controller (MVC) architectural pattern. It provides developers with a robust set of tools and conventions for building web applications efficiently. Two essential components of Rails that play a significant role in handling incoming requests, processing them, and generating responses are ActionPack and Middleware. In this tutorial, we will delve deep into these components, exploring their functionalities and how they fit into the Rails framework.
Table of Contents
1. What is ActionPack?
ActionPack is a key component of the Ruby on Rails framework responsible for handling incoming HTTP requests, routing them to the appropriate controller actions, and generating HTTP responses. It encapsulates the controller and view layers of the MVC pattern.
1.1. The Controller Layer
The controller layer in ActionPack is responsible for receiving HTTP requests from clients (usually web browsers) and routing them to the appropriate controller action. Each controller action corresponds to a specific URL route and is responsible for processing the request and generating an appropriate response.
Let’s take a look at a simple example of a controller action:
ruby class UsersController < ApplicationController def index @users = User.all end end
In this example, the index action of the UsersController retrieves a list of users from the database and renders an associated view.
1.2. The View Layer
The view layer in ActionPack is responsible for generating HTML responses that will be sent back to the client. Views typically use embedded Ruby (ERb) templates to mix Ruby code with HTML markup.
Here’s an example of a view template:
html <!DOCTYPE html> <html> <head> <title>Users</title> </head> <body> <h1>List of Users</h1> <ul> <% @users.each do |user| %> <li><%= user.name %></li> <% end %> </ul> </body> </html>
In this example, the view generates an HTML page listing all the users retrieved by the index action.
1.3. Routing
Routing is a crucial part of the controller layer in ActionPack. It determines which controller action should handle a specific incoming request based on the request’s URL. Rails uses a configuration file called routes.rb to define these routes.
Here’s a simple route definition:
ruby Rails.application.routes.draw do get '/users', to: 'users#index' end
In this example, a GET request to the /users URL will be routed to the index action of the UsersController.
2. What is Middleware?
Middleware is a concept in web development that refers to software components placed between a client and a server. In the context of Ruby on Rails, middleware sits between the web server (e.g., Puma or Unicorn) and the Rails application, intercepting and processing requests and responses.
Middleware components are organized into a stack, and each component in the stack has the opportunity to modify the request or response before passing it along to the next middleware or the application itself.
2.1. Rack Middleware
Rails is built on top of the Rack web server interface, which is a minimalistic web server interface for Ruby. Rack middleware is a set of components that conform to the Rack interface and can be used with any Rack-compatible web framework, including Ruby on Rails.
In a Rails application, middleware components are defined in the config/application.rb file under the config.middleware configuration block. Here’s an example of how middleware components are added to the stack:
ruby config.middleware.use MyCustomMiddleware config.middleware.insert_before AnotherMiddleware, MyCustomMiddleware config.middleware.insert_after YetAnotherMiddleware, MyCustomMiddleware
2.2. Common Uses of Middleware in Rails
Middleware in Ruby on Rails serves various purposes, and you can even create custom middleware to suit your application’s specific needs. Here are some common use cases for middleware in Rails:
2.2.1. Authentication and Authorization
Middleware can be used to perform user authentication and authorization checks before allowing access to certain parts of your application. For example, you might use the popular Devise gem to handle user authentication as middleware.
2.2.2. Request Logging
You can create custom middleware to log incoming requests, helping you monitor and troubleshoot your application’s behavior.
2.2.3. CORS Handling
Middleware can handle Cross-Origin Resource Sharing (CORS) headers to control which domains can access your API.
2.2.4. Caching
Middleware can implement caching mechanisms to improve the performance of your application by serving cached responses for frequently accessed resources.
2.3. An Example Middleware
Let’s create a simple middleware to log incoming requests. This middleware will intercept requests, log information about them, and then pass the request along to the application.
ruby class RequestLoggerMiddleware def initialize(app) @app = app end def call(env) # Log request information Rails.logger.info("Request: #{env['REQUEST_METHOD']} #{env['REQUEST_URI']}") # Pass the request along to the application response = @app.call(env) # Log response information Rails.logger.info("Response: #{response[0]}") # Return the response response end end
In this example, the RequestLoggerMiddleware logs information about incoming requests and responses using the Rails logger. To use this middleware in your Rails application, you would add it to the middleware stack in config/application.rb:
ruby config.middleware.use RequestLoggerMiddleware
Now, every incoming request will be logged with its method and URI, and the response status code will also be logged.
3. How ActionPack and Middleware Work Together
ActionPack and middleware work together seamlessly in a Rails application to handle incoming requests and generate responses. Here’s how the process typically flows:
- Incoming Request: A client sends an HTTP request to the Rails application, which is running on a web server like Puma.
- Middleware Stack: The request first enters the middleware stack. Middleware components in the stack have the opportunity to modify the request or perform actions such as authentication, logging, or CORS handling.
- Routing (ActionPack): After passing through any relevant middleware, the request reaches the ActionPack layer. ActionPack uses the routing configuration (config/routes.rb) to determine which controller and action should handle the request.
- Controller Action (ActionPack): The request is dispatched to the appropriate controller action, which is responsible for processing the request’s logic and preparing data for the response.
- View Rendering (ActionPack): Once the controller action has completed its work, it typically renders a view. The view is an HTML template that generates the final HTML response by combining data from the controller with an HTML template.
- Outgoing Response: The final HTML response is sent back through the middleware stack, allowing middleware to perform any additional actions on the response.
- Client Response: The processed response is sent back to the client, usually a web browser, which renders the page for the user.
4. Code Sample: Combining ActionPack and Middleware
Let’s put together what we’ve learned by creating a simple middleware that adds a custom response header to every HTTP response generated by a Rails application. This header will provide information about the server’s response time.
ruby class ResponseTimeMiddleware def initialize(app) @app = app end def call(env) start_time = Time.now response = @app.call(env) end_time = Time.now response[1]['X-Response-Time'] = "#{(end_time - start_time).to_f} seconds" response end end
In this middleware, we record the start time when the request enters the middleware, and the end time after the application has processed the request. We then calculate the response time and add it as a custom header (X-Response-Time) to the response.
To use this middleware, add it to your config/application.rb:
ruby config.middleware.use ResponseTimeMiddleware
Now, every response generated by your Rails application will include the X-Response-Time header with the server’s response time.
Conclusion
Understanding ActionPack and Middleware is essential for any Ruby on Rails developer. ActionPack forms the core of Rails, handling incoming requests and generating responses by routing requests to controllers and rendering views. Middleware, on the other hand, provides a powerful mechanism to intercept and process requests and responses, allowing you to add custom functionality to your application.
By combining ActionPack’s controller and view layers with middleware, you can create robust and feature-rich web applications that efficiently handle a wide range of tasks, from authentication and authorization to logging and performance monitoring.
As you continue to explore Ruby on Rails, mastering these components will empower you to build web applications that are not only functional but also maintainable and extensible. So, dive deeper, experiment with different middleware, and leverage ActionPack’s capabilities to create web applications that meet your users’ needs effectively. Happy coding!
Table of Contents
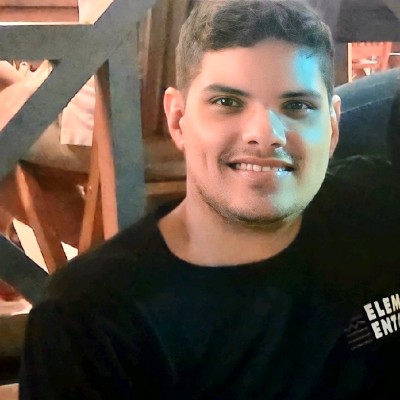
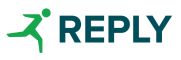