Ruby on Rails Tutorial: Understanding ActionView and Template Rendering
Ruby on Rails is a robust web development framework that follows the Model-View-Controller (MVC) architecture, allowing developers to build powerful and maintainable web applications. One of the essential components of the Rails framework is ActionView, responsible for rendering templates and handling the presentation logic of your application. In this tutorial, we will dive deep into ActionView and template rendering in Ruby on Rails, exploring key concepts, best practices, and code samples to help you master this crucial aspect of Rails development.
Table of Contents
1. What is ActionView?
Before we delve into the details of ActionView and template rendering, let’s start by understanding what ActionView is.
ActionView is the component of Ruby on Rails that deals with the user interface and presentation layer of your web application. It provides a structured way to generate HTML and other types of markup, making it easier to create dynamic and interactive web pages.
ActionView’s primary responsibilities include:
1.1. Template Rendering
At its core, ActionView is responsible for rendering templates. Templates are files that contain a mix of HTML and Ruby code. These templates serve as the blueprints for generating the final HTML that gets sent to the user’s browser.
1.2. Layouts
ActionView also manages layouts, which are templates that define the overall structure of your application’s pages. Layouts often include common elements like headers, footers, and navigation menus, providing a consistent look and feel across your site.
1.3. Helpers
ActionView comes with a set of built-in helper methods that simplify the generation of HTML elements, links, forms, and more. Helpers are a crucial part of Rails development as they promote code reusability and maintainability.
1.4. Partials
Partials are reusable template fragments that can be included within other templates. They are handy for reducing code duplication and promoting the DRY (Don’t Repeat Yourself) principle in your views.
Now that we have a basic understanding of ActionView, let’s explore the core concepts related to template rendering in Ruby on Rails.
2. The Template Rendering Process
When a user accesses a page in your Ruby on Rails application, several steps occur behind the scenes to render the appropriate template and deliver the HTML response. Let’s break down the template rendering process step by step:
2.1. Routing
The journey begins with the Rails router. The router maps incoming requests (URLs) to specific controller actions. Each controller action is responsible for handling a particular request and deciding which template to render.
2.2. Controller
Once the router determines the appropriate controller and action, the controller code is executed. In the controller action, you can fetch data from the database, perform calculations, and set variables that will be used in the view.
Here’s a simplified example:
ruby class UsersController < ApplicationController def show @user = User.find(params[:id]) end end
In this example, the show action fetches a user from the database and assigns it to the @user instance variable.
2.3. View
After the controller action has run its course, Rails automatically looks for a corresponding view template. By convention, the view template is named after the controller action (e.g., show.html.erb for the show action in the UsersController).
2.4. Template Rendering
The template rendering process is where ActionView comes into play. It takes the view template (usually written in HTML with embedded Ruby code) and processes it, replacing Ruby code with actual values. This results in a complete HTML page that can be sent to the user’s browser.
Here’s a simplified view template:
erb <h1>User Profile</h1> <p>Name: <%= @user.name %></p> <p>Email: <%= @user.email %></p>
In this template, <%= @user.name %> and <%= @user.email %> are placeholders for the actual user data fetched in the controller. ActionView replaces these placeholders with the corresponding values, producing a dynamic HTML page.
2.5. Layout
In most cases, the rendered view template is embedded within a layout. The layout provides the overall structure for the page and often includes headers, footers, and navigation menus. The view template is inserted into the layout using a special yield command.
Here’s a simplified layout example:
erb <!DOCTYPE html> <html> <head> <title>My Rails App</title> </head> <body> <%= yield %> </body> </html>
The <%= yield %> statement indicates where the content of the view template should be inserted.
2.6. Response
Once the view template is rendered within the layout, Rails generates a complete HTML response and sends it to the user’s browser.
3. ERB Templates in ActionView
Ruby on Rails primarily uses ERB (Embedded Ruby) templates for rendering views. ERB templates are a combination of HTML and Ruby code, making it easy to embed dynamic content within static HTML.
Let’s take a closer look at some common elements and techniques used in ERB templates within ActionView:
3.1. Interpolation
Interpolation allows you to insert Ruby code into your HTML templates using <%= … %> tags. This is how you dynamically display data from your controller in the view.
erb <h1>Welcome, <%= @user.name %>!</h1>
In this example, @user.name is interpolated into the HTML, producing a personalized greeting.
3.2. Conditionals
You can use Ruby’s if statements to conditionally render HTML elements based on certain criteria. For instance, you can display different content to logged-in and logged-out users:
erb <% if current_user %> <p>Welcome, <%= current_user.name %>!</p> <% else %> <p>Please log in to access your account.</p> <% end %>
In this snippet, the content displayed depends on whether current_user is present.
3.3. Loops
ERB templates also allow you to iterate over collections using Ruby’s each method. This is useful for displaying lists of items, such as blog posts or comments:
erb <ul> <% @posts.each do |post| %> <li><%= post.title %></li> <% end %> </ul>
Here, each post in the @posts collection is iterated through and displayed in a list item.
3.4. Partials
To keep your templates DRY, you can use partials to encapsulate reusable pieces of HTML and Ruby code. Partial templates are prefixed with an underscore (e.g., _post.html.erb), and they can be included in other templates using the render method:
erb <%= render partial: 'post', locals: { post: @post } %>
In this example, the _post.html.erb partial is rendered with the @post variable passed as a local.
4. Layouts and Views
In Ruby on Rails, layouts and views work together to create a structured and consistent user interface for your web application. Let’s explore the role of layouts and views in more detail:
4.1. Layouts
Layouts provide the overall structure for your web pages. They define the common elements that appear on every page of your application, such as headers, footers, and navigation menus. Layouts ensure a consistent look and feel throughout your site.
Here’s an example of a simple layout:
erb <!DOCTYPE html> <html> <head> <title>My Rails App</title> </head> <body> <header> <h1>Welcome to My App</h1> <%= yield %> </header> <footer> © <%= Time.now.year %> My App </footer> </body> </html>
In this layout, the <%= yield %> statement indicates where the content of the view template should be inserted. This allows each page to have unique content while sharing the same structure.
4.2. Views
Views, on the other hand, are responsible for the content of a specific page. They are templates that contain the HTML and embedded Ruby code for displaying data and generating the page’s content.
Here’s an example of a view template:
erb <h2>About Us</h2> <p>Lorem ipsum dolor sit amet, consectetur adipiscing elit. Vestibulum faucibus accumsan odio, eget sollicitudin nisl tincidunt nec.</p>
This view template, when combined with the layout, produces a complete web page with a consistent header and footer.
5. Helper Methods
Ruby on Rails provides a wide range of built-in helper methods that make it easier to generate HTML and other markup. These helpers simplify common tasks, such as creating links, forms, and complex HTML elements.
Let’s explore some of the most commonly used helper methods in ActionView:
5.1. link_to
The link_to helper generates HTML links. It’s especially useful for creating links to other pages within your application.
erb <%= link_to 'Home', root_path %> <%= link_to 'Profile', user_path(current_user) %>
In these examples, link_to generates links to the home page and the user’s profile page, respectively.
5.2. form_for
The form_for helper simplifies the creation of HTML forms for creating, editing, and deleting records in your database. It automatically generates the necessary HTML and sets up the form to submit data to the appropriate controller action.
erb <%= form_for @user do |f| %> <%= f.label :name %> <%= f.text_field :name %> <%= f.submit 'Update' %> <% end %>
In this example, form_for generates a form for updating a user’s name.
5.3. image_tag
The image_tag helper generates HTML <img> tags for displaying images. It simplifies the process of including images in your views.
erb <%= image_tag 'logo.png' %>
This example generates an HTML <img> tag to display a logo image named ‘logo.png’.
5.4. content_tag
The content_tag helper allows you to create HTML tags with custom content. This is useful when you need to generate HTML elements that don’t have dedicated helpers.
erb <%= content_tag :div, 'This is a custom div', class: 'custom-div' %>
In this snippet, content_tag generates a <div> element with custom content and a specified CSS class.
These are just a few examples of the many helper methods available in ActionView. Helpers make it easier to write clean and maintainable views by encapsulating common HTML generation tasks.
Conclusion
In this tutorial, we’ve explored the fundamental concepts of ActionView and template rendering in Ruby on Rails. We’ve learned how ActionView handles the presentation layer of your application, including template rendering, layouts, helper methods, and more.
Understanding ActionView is essential for building dynamic and interactive web applications with Ruby on Rails. By mastering the concepts and techniques discussed in this tutorial, you’ll be well-equipped to create rich and engaging user interfaces for your Rails projects.
As you continue your Rails journey, don’t hesitate to dive deeper into ActionView’s documentation and explore more advanced topics, such as custom helpers, view partials, and internationalization (i18n) for building applications that can reach a global audience. Happy coding!
Table of Contents
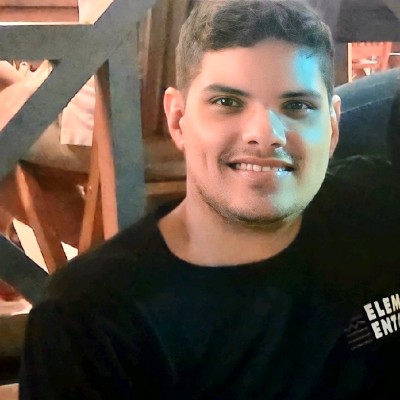
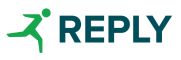