Ruby on Rails Tutorial: Understanding ActiveJob and Background Processing
In today’s fast-paced web development landscape, delivering a seamless user experience is paramount. As applications grow in complexity, handling time-consuming tasks synchronously within the request-response cycle can lead to performance bottlenecks and user dissatisfaction. To overcome these challenges, Ruby on Rails offers ActiveJob, a powerful framework for background processing. In this tutorial, we will explore ActiveJob and its benefits, understand how it integrates with background processing libraries, and dive into practical examples to demonstrate how to leverage it effectively.
Table of Contents
1. What is ActiveJob?
ActiveJob is a framework introduced in Ruby on Rails 4.2 that simplifies the process of creating and executing background tasks. It abstracts away the implementation details of different queuing systems, allowing developers to write code once and run it with various backends. This flexibility makes it easy to switch between different background processing libraries without modifying the application code.
2. Advantages of Background Processing
Background processing has become a crucial aspect of modern web development. By moving time-consuming tasks to background jobs, the application can handle incoming requests more efficiently, leading to a better user experience. Some key advantages of background processing include:
- Improved Responsiveness: Background processing frees up the main application thread, ensuring that user interactions are not blocked during resource-intensive tasks.
- Scalability: By offloading tasks to background workers, Rails applications can scale more effectively and handle increased load without performance degradation.
- Fault Tolerance: Background jobs can be retried in case of failures, reducing the chances of losing critical data or functionality.
- Enhanced User Experience: As background jobs work asynchronously, users experience faster response times, resulting in a smoother and more enjoyable application.
3. Setting Up ActiveJob
To use ActiveJob in your Ruby on Rails application, follow these steps:
3.1. Installation:
ActiveJob comes pre-installed with Rails 4.2 and later versions. If you are using an older version, consider upgrading your Rails installation to leverage this powerful feature.
3.2. Configuration:
ActiveJob requires minimal configuration. Ensure that your config/application.rb file contains the following line:
ruby config.active_job.queue_adapter = :<QUEUE_ADAPTER>
Replace <QUEUE_ADAPTER> with the name of your preferred queuing system. By default, Rails uses the :async adapter, which runs jobs immediately in the same process. However, for production use and better performance, it is recommended to use dedicated queuing libraries like Sidekiq or Resque.
4. Creating Your First ActiveJob
Let’s dive into creating a basic ActiveJob to get a better understanding of its structure and functionality.
4.1. Defining the Job:
To define an ActiveJob, run the following command in your terminal:
ruby rails generate job MyExampleJob
This command will create a new file named my_example_job.rb in the app/jobs directory. Open this file to see the job’s skeleton code.
ruby class MyExampleJob < ApplicationJob queue_as :default def perform(*args) # Your background job logic goes here end end
4.2. Enqueuing the Job:
To enqueue the job, you can call the perform_later method on the job class and pass any required arguments.
ruby MyExampleJob.perform_later(arg1, arg2)
The job will be pushed to the queue, and a background processor will execute it when resources are available.
4.3. Executing the Job:
When a background processor picks up the job from the queue, it calls the perform method of the corresponding job class. This is where you define the actual logic of your background task.
5. ActiveJob Callbacks
ActiveJob provides callbacks that allow you to execute code at different stages of job processing. These callbacks can be useful for tasks such as logging, error handling, or sending notifications.
Here are some commonly used callbacks:
- before_enqueue: Executes before the job is added to the queue.
- around_enqueue: Wraps the job’s enqueuing process, allowing you to execute code before and after enqueuing.
- after_enqueue: Executes after the job has been added to the queue.
Example:
ruby class MyExampleJob < ApplicationJob queue_as :default before_enqueue do |job| Rails.logger.info("About to enqueue #{job.class}") end def perform(*args) # Your background job logic goes here end end
6. Parameters and Arguments in ActiveJob
ActiveJob allows you to pass arguments to your background jobs. This flexibility enables you to work with data from the main application and process it asynchronously.
To pass arguments to the job, modify the perform method in your job class to accept the desired parameters.
ruby class MyExampleJob < ApplicationJob queue_as :default def perform(user_id, message) user = User.find(user_id) send_notification(user, message) end end
You can then enqueue the job with the required arguments:
ruby MyExampleJob.perform_later(current_user.id, "Hello, world!")
7. Using Background Processing Libraries
While Rails’ default :async adapter is suitable for development and testing purposes, it is not recommended for production environments. For production use, you should consider using dedicated background processing libraries such as Sidekiq or Resque.
7.1. Sidekiq:
Sidekiq is one of the most popular background processing libraries for Ruby on Rails. It provides robust job scheduling and processing capabilities, backed by Redis. To use Sidekiq, add the sidekiq gem to your Gemfile and run bundle install.
Next, update the queue adapter configuration in config/application.rb:
ruby config.active_job.queue_adapter = :sidekiq
7.2. Resque:
Resque is another widely used background processing library that works well with Rails. It uses Redis to store job data. To use Resque, add the resque gem to your Gemfile and run bundle install.
Update the queue adapter configuration in config/application.rb:
ruby config.active_job.queue_adapter = :resque
8. Error Handling and Retries
In real-world scenarios, background jobs may sometimes fail due to various reasons, such as network issues or service unavailability. ActiveJob provides built-in mechanisms for error handling and retries.
You can define the maximum number of times a job should be retried and specify the conditions under which the retry should occur. By default, ActiveJob retries the job up to 25 times with an exponential backoff delay between retries.
Example:
ruby class MyExampleJob < ApplicationJob queue_as :default retry_on StandardError, wait: :exponentially_longer, attempts: 5 def perform(*args) # Your background job logic goes here end end
9. Job Prioritization and Scheduling
In some applications, it’s essential to prioritize certain jobs over others or schedule jobs to run at specific times. ActiveJob supports both job prioritization and scheduling.
9.1. Job Prioritization:
ActiveJob allows you to assign different priorities to jobs. Higher-priority jobs get dequeued before lower-priority ones. The default priority is set to 0, and you can assign any integer value as a priority.
ruby class MyExampleJob < ApplicationJob queue_as :default queue_with_priority 10 def perform(*args) # Your background job logic goes here end end
9.2. Job Scheduling:
You can also schedule jobs to run at specific times using the perform_later method with a delay or a specific timestamp.
ruby MyExampleJob.set(wait: 1.hour).perform_later(arg1, arg2)
10. Monitoring and Administration
When using background processing libraries like Sidekiq or Resque, they usually come with monitoring and administration tools that allow you to view the state of your queues, track job performance, and handle job retries.
11. Scaling and Deployment Considerations
As your application grows and your background job queue experiences more traffic, you may need to scale your background processing infrastructure. Ensure that your queuing system is running efficiently, and consider using techniques like load balancing and clustering for high availability.
Additionally, consider the resource requirements of your background jobs and allocate system resources accordingly.
Conclusion:
In this tutorial, we explored the power of ActiveJob and background processing in Ruby on Rails. We discussed the advantages of background processing and saw how to set up and use ActiveJob to offload time-consuming tasks from the main application thread. We also delved into practical examples, demonstrating how to pass arguments, use callbacks, and handle errors in background jobs.
By leveraging ActiveJob and background processing libraries like Sidekiq or Resque, you can build efficient, scalable, and high-performance applications that provide a smooth user experience even under heavy loads. So, go ahead and make the most of ActiveJob to optimize your Rails applications!
Table of Contents
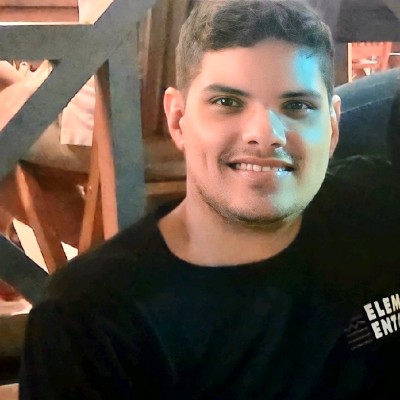
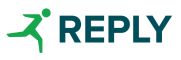