Working with ActiveRecord and Databases on Ruby on Rails
Ruby on Rails, popularly known as Rails, is a robust web application framework, designed to facilitate software development with a lot less effort. This tutorial is designed to walk you through the steps of working with ActiveRecord, a powerful ORM (Object-Relational Mapping) provided by Rails, and databases.
Introduction to ActiveRecord
ActiveRecord is the M (Model) in MVC (Model-View-Controller), the architectural pattern Rails uses. It provides a high-level interface between Ruby and the underlying database.
Setting up a new Rails Application
Before we delve deeper, let’s set up a simple Rails application. If you haven’t installed Rails on your system, you can follow the instructions from the official [Ruby on Rails guide](https://guides.rubyonrails.org/v6.0/getting_started.html).
Once you’ve set up Rails, create a new Rails application:
```bash rails new MyDatabaseApp ``` Navigate to the new directory: ```bash cd MyDatabaseApp
Database Configuration
By default, Rails uses SQLite3 as the database. To check your database settings, open the `config/database.yml` file. This file is used to define your database connection parameters for different environments (development, test, production).
ActiveRecord Basics
ActiveRecord works by mapping database tables to Ruby classes, rows to objects, and columns to object attributes. If you have a table named `users`, ActiveRecord would relate to a class `User`. Each row in the `users` table is an instance of the `User` class, and the table’s columns correspond to the attributes of the `User` objects.
Generating a Model
Let’s generate a `User` model:
```bash rails generate model User name:string email:string
This creates a new model named `User` with attributes `name` and `email`. It also generates a database migration to create the corresponding `users` table.
Database Migrations
Migrations are a way to alter the database schema over time. Rails generates a timestamped migration file each time you generate a model or scaffold. You can run migrations using:
```bash rails db:migrate
This will create a `users` table with `name` and `email` columns, plus an additional `id` column which is auto-incremented and used as the primary key. It also adds `created_at` and `updated_at` columns automatically to record timestamps.
CRUD Operations
With our `User` model, we can perform CRUD operations: Create, Read, Update, and Delete.
Create: To create a new user:
```ruby user = User.create(name: 'John Doe', email: 'john.doe@example.com')
Read: To fetch users from the database:
```ruby users = User.all # Fetch all users user = User.find(1) # Fetch user with ID 1 user = User.find_by(email: 'john.doe@example.com') # Fetch user by email ```
Update: To update a user’s details:
```ruby user = User.find(1) user.update(email: 'new.email@example.com')
Delete: To delete a user:
```ruby user = User.find(1) user.destroy ```
Advanced ActiveRecord
Beyond these basic operations, ActiveRecord offers advanced features such as validations, associations, scopes, and more.
Validations
ActiveRecord validations provide an easy way to ensure that only valid data is saved into your database. Here’s an example of adding validations to the `User` model:
```ruby class User < ApplicationRecord validates :name, presence: true, length: { maximum: 50 } validates :email, presence: true, length: { maximum: 255 } end ```
Associations
ActiveRecord associations are used to define relationships between models. There are six types of associations: `belongs_to`, `has_one`, `has_many`, `has_many :through`, `has_one :through`, and `has_and_belongs_to_many`.
Assume we have another model `Article` that is associated with `User` as a user has many articles. Here’s how we’d define this:
```ruby class User < ApplicationRecord has_many :articles end class Article < ApplicationRecord belongs_to :user end
Scopes
Scopes are custom queries that you define in your model. They improve code readability and help avoid code repetition. Here’s an example:
```ruby class User < ApplicationRecord scope :recent, -> { order(created_at: :desc) } end
To fetch recently created users:
```ruby User.recent
Conclusion
In conclusion, Ruby on Rails, coupled with ActiveRecord, offers a robust and easy-to-use framework for developing database-driven web applications. This tutorial provided an overview of working with databases in Rails, from setting up a new Rails application to performing basic and advanced ActiveRecord operations.
We touched upon database configuration, creating models, performing CRUD operations, and understanding migrations. We also delved into some advanced ActiveRecord features such as validations, associations, and scopes. These concepts are fundamental in Rails development, and understanding them would empower you to leverage the power of ActiveRecord effectively.
While this tutorial provided a solid foundation, the world of ActiveRecord is expansive, and there’s always more to learn. I encourage you to continue exploring and experimenting with ActiveRecord, as real-world Rails applications often require complex queries and data manipulation. Always remember, practice makes perfect. Happy Rails coding!
Table of Contents
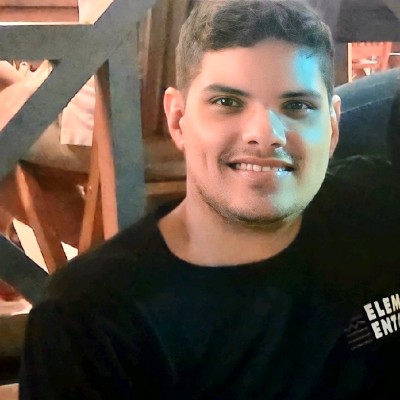
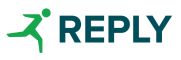