Ruby on Rails Q & A
What is ActiveRecord in Ruby on Rails?
ActiveRecord is the Object-Relational Mapping (ORM) system built into Ruby on Rails. It’s a bridge between the relational database and the object-oriented world of your Rails application. Here’s an explanation of its essence and significance:
- ORM Explained: At a high level, ORMs allow developers to interact with their databases, like querying or persisting data, without writing SQL queries directly. Instead, they work with objects and methods in the programming language they are using, in this case, Ruby.
- ActiveRecord’s Conventions: One of the standout features of ActiveRecord is its emphasis on convention over configuration. By following naming conventions, a lot of the configuration is done automatically. For instance, if you have a `User` model in Rails, ActiveRecord will automatically look for a `users` table in the database.
- CRUD Operations: ActiveRecord simplifies the process of creating, reading, updating, and deleting database records. Instead of hand-writing SQL, you can use Ruby methods. For example, to retrieve all records from the `users` table, you’d use `User.all`. To create a new record, you’d build a new user object (e.g., `user = User.new`) and then call `user.save`.
- Query Interface: ActiveRecord provides a rich query interface. Queries are chainable and written in Ruby, making them readable and concise. For example, `User.where(first_name: ‘John’).order(created_at: :desc)` fetches all users named John and orders them by their creation date.
- Validations and Callbacks: Beyond database interaction, ActiveRecord supports data validations and callbacks. Validations ensure data integrity (e.g., making sure an email is present and unique), while callbacks are hooks into the lifecycle of an object (e.g., sending a welcome email after a new user is created).
- Associations: ActiveRecord simplifies defining relationships between models (like one-to-many or many-to-many) using simple conventions. If a `User` has many `Articles`, you can declare `has_many :articles` in the User model and `belongs_to :user` in the Article model.
ActiveRecord is a core component of Rails that provides an intuitive interface for database interactions. It encapsulates a lot of the boilerplate and intricacies of database communication, allowing developers to focus on application logic, maintain cleaner code, and optimize productivity.
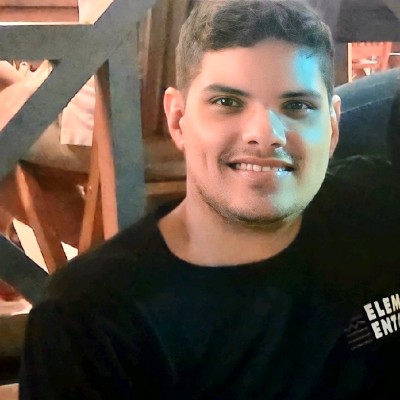
Previously at
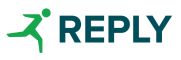
Senior Software Engineer with a focus on remote work. Proficient in Ruby on Rails. Expertise spans y6ears in Ruby on Rails development, contributing to B2C financial solutions and data engineering.