Ruby on Rails Tutorial: Understanding Asset Pipeline and Asset Management
Ruby on Rails is a powerful web application framework known for its convention over configuration (CoC) and don’t repeat yourself (DRY) principles. One of the key components that helps developers adhere to these principles and streamline the development process is the Asset Pipeline. In this tutorial, we will dive deep into understanding the Asset Pipeline and how it simplifies asset management in Rails applications.
Table of Contents
1. What are Assets in a Rails Application?
Before we delve into the Asset Pipeline, let’s clarify what assets are in the context of a Ruby on Rails application. Assets are the static files required for your web application to function correctly. These files include stylesheets (CSS), JavaScript files, images, fonts, and more. Assets play a crucial role in shaping the user experience and aesthetics of your web application.
2. The Need for Asset Management
In a typical web application, you might have multiple CSS files, JavaScript files, and various other assets. Without proper management, this can quickly become chaotic, leading to issues such as increased load times, inefficient use of resources, and code redundancy. This is where the Asset Pipeline comes to the rescue.
3. What is the Asset Pipeline?
The Asset Pipeline is a feature in Ruby on Rails designed to manage and optimize your application’s assets efficiently. It provides a structure and set of tools for organizing, processing, and serving these static assets. By following conventions, Rails simplifies the asset management process, making it easier for developers to work on the front-end without worrying about the nitty-gritty details of asset organization and optimization.
3.1. Key Features of the Asset Pipeline
3.1.1. Concatenation
One of the primary features of the Asset Pipeline is the ability to concatenate multiple CSS and JavaScript files into a single file. This reduces the number of HTTP requests needed to load your web page, resulting in faster load times.
ruby # app/assets/javascripts/application.js //= require jquery //= require bootstrap //= require_tree .
In this example, the //= require directive is used to include jQuery, Bootstrap, and all other JavaScript files within the app/assets/javascripts directory into a single application.js file.
3.1.2. Minification
The Asset Pipeline also minifies your assets by removing unnecessary whitespace and reducing file sizes. Smaller files mean faster downloads and improved page load times.
ruby # app/assets/javascripts/application.js //= require jquery //= require bootstrap.min //= require_tree .
In this snippet, the bootstrap.min.js file is used instead of the regular bootstrap.js, which is minified for optimal performance.
3.1.3. Asset Compression
To further optimize your application, the Asset Pipeline can also compress assets like CSS and JavaScript using gzip or other compression algorithms. This reduces the amount of data transferred over the network, resulting in quicker page loads.
ruby # config/application.rb config.assets.compress = true
By enabling asset compression in the application configuration, Rails will automatically compress your assets when they are served to the client.
3.1.4. Caching
Caching is essential for improving performance. The Asset Pipeline provides built-in support for caching, ensuring that assets are cached by the client’s browser. When assets change, a unique fingerprint is added to their filenames to force the browser to fetch the latest version.
ruby # config/environments/production.rb config.assets.digest = true
Enabling asset digesting ensures that files like application.js become application-6a8f72bc28b32b79bb3ea2a63b3fcf4b.js, making it easy to manage cache updates.
4. Using the Asset Pipeline in Your Rails Application
Now that you have an overview of the Asset Pipeline’s features, let’s see how you can use it in your Ruby on Rails application.
4.1. Organizing Your Assets
Rails follows a specific directory structure for managing assets. Place your JavaScript files in the app/assets/javascripts directory and your CSS files in the app/assets/stylesheets directory. Images and other assets go into the app/assets/images directory.
markdown app/ assets/ javascripts/ application.js stylesheets/ application.css images/ logo.png
This organization ensures that your assets are easily discoverable and well-structured.
4.2. Including Assets in Views
To include CSS and JavaScript assets in your views, you can use Rails helper methods like stylesheet_link_tag and javascript_include_tag. For example:
erb <!DOCTYPE html> <html> <head> <%= stylesheet_link_tag 'application' %> </head> <body> <!-- Your content here --> <%= javascript_include_tag 'application' %> </body> </html>
These helpers generate the appropriate HTML tags to include the assets, taking into account the Asset Pipeline’s optimizations.
4.3. Precompiling Assets
In production environments, you’ll want to precompile your assets to optimize performance. This process generates a single minified and compressed file for each asset type.
To precompile your assets, run the following command:
bash rails assets:precompile
This command will generate precompiled assets in the public/assets directory.
4.4. Asset Manifests
Asset manifests are files that specify the dependencies and order of your assets. For JavaScript, the application.js file acts as the asset manifest by default. You can include other JavaScript files within it using the //= require directive, as shown earlier.
For CSS, the application.css file serves as the manifest. You can include stylesheets using the *= require directive.
4.5. Customizing the Asset Pipeline
The Asset Pipeline provides various configuration options to customize its behavior. You can configure settings such as asset versioning, asset compression, and more in your Rails application’s configuration files.
ruby # config/application.rb config.assets.version = '1.0'
In this example, the asset version is set to ‘1.0’, which can be useful for cache-busting purposes when you update your assets.
5. Debug vs. Production Mode
It’s important to note that the Asset Pipeline behaves differently in development (debug) and production (production) modes.
5.1. Development Mode
In development mode, assets are served individually and uncompressed. This makes it easier to debug and inspect your assets using browser developer tools. However, it may result in slower page load times due to multiple asset requests.
5.2. Production Mode
In production mode, assets are precompiled, concatenated, and compressed. This minimizes the number of requests and reduces asset file sizes, resulting in faster page load times for end-users.
To switch between these modes, you can set the RAILS_ENV environment variable. For example, to run your Rails application in production mode, use:
bash RAILS_ENV=production rails server
6. Asset Pipeline Gotchas
While the Asset Pipeline is a powerful tool, it’s essential to be aware of potential challenges and gotchas when working with it.
6.1. Asset Fingerprinting
Asset fingerprinting, which appends a unique hash to asset filenames, can sometimes lead to issues when using external services or content delivery networks (CDNs). Ensure that your CDN or service supports automatic cache invalidation based on file changes.
6.2. Asset Ordering
The order in which you include assets matters, especially when dealing with JavaScript files with dependencies. Make sure to include them in the correct order within your asset manifests.
6.3. Asset Preprocessing
Custom asset preprocessing can be tricky. If you have unique requirements for your assets, be prepared to configure and test your asset pipeline accordingly.
Conclusion
The Asset Pipeline is a valuable feature in Ruby on Rails that simplifies asset management, improves performance, and streamlines the web development workflow. By organizing, concatenating, minifying, and compressing assets, Rails ensures that your web application delivers a faster and more efficient user experience.
In this tutorial, we’ve explored the key features of the Asset Pipeline, how to organize and include assets in your views, and the differences between development and production modes. Understanding how to leverage the Asset Pipeline effectively will enhance your ability to build robust and performant Rails applications. So, go ahead and make the most of this powerful tool in your next project!
Table of Contents
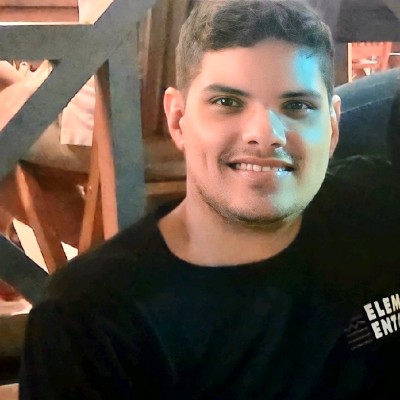
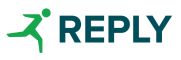