Ruby on Rails Tutorial: Understanding Associations and Relationships
Ruby on Rails is a powerful web application framework that follows the Model-View-Controller (MVC) architectural pattern. One of the key features of Rails is its seamless support for managing associations and relationships between different models using ActiveRecord. Understanding how to establish and utilize these associations is crucial for building robust and efficient applications.
In this tutorial, we will delve into the world of associations and relationships in Ruby on Rails. We will explore the different types of associations, discuss the appropriate use cases for each, and provide comprehensive examples to solidify your understanding. So, let’s get started!
What are Associations?
In Rails, associations represent relationships between different models. By defining associations, we can easily navigate and query related records, eliminating the need for complex SQL joins. There are several types of associations in Rails, each serving a specific purpose depending on the nature of the relationship between models.
One-to-One Associations
A one-to-one association is established when each record in one model corresponds to exactly one record in another model. To illustrate this, let’s consider an example where we have a User model and an Address model.
ruby class User < ApplicationRecord has_one :address end class Address < ApplicationRecord belongs_to :user end
In this example, a user has one address, and an address belongs to a user. Rails provides methods like build_association and create_association to facilitate working with one-to-one associations.
One-to-Many Associations
A one-to-many association is established when a record in one model is associated with multiple records in another model. For instance, let’s assume we have a User model and a Post model.
ruby class User < ApplicationRecord has_many :posts end class Post < ApplicationRecord belongs_to :user end
In this example, a user can have multiple posts, while each post belongs to a single user. Rails automatically generates helpful methods such as user.posts to retrieve all posts associated with a user, and post.user to access the user who owns a specific post.
Many-to-Many Associations
A many-to-many association is established when multiple records in one model can be associated with multiple records in another model. Consider a scenario where we have a User model and a Group model, and each user can belong to multiple groups, while each group can have multiple users.
ruby class User < ApplicationRecord has_many :memberships has_many :groups, through: :memberships end class Group < ApplicationRecord has_many :memberships has_many :users, through: :memberships end class Membership < ApplicationRecord belongs_to :user belongs_to :group end
Here, we introduce a join model called Membership, which connects the User and Group models. Through the use of the has_many :through association, Rails enables seamless querying and manipulation of many-to-many relationships.
Polymorphic Associations
Polymorphic associations allow a model to belong to more than one other model on a single association. This is useful when a model can be associated with multiple types of other models. For example, consider a Comment model that can be associated with both a Post model and an Image model.
ruby class Comment < ApplicationRecord belongs_to :commentable, polymorphic: true end class Post < ApplicationRecord has_many :comments, as: :commentable end class Image < ApplicationRecord has_many :comments, as: :commentable end
In this example, the commentable association in the Comment model can refer to either a Post or an Image. Rails handles the association resolution behind the scenes, providing a clean and flexible way to handle polymorphic relationships.
Through Associations
Through associations allow you to create associations that traverse other associations. It provides a convenient way to access data indirectly through intermediary models. Let’s consider an example where we have a User model, a Group model, and a Membership model connecting the two.
ruby class User < ApplicationRecord has_many :memberships has_many :groups, through: :memberships end class Group < ApplicationRecord has_many :memberships has_many :users, through: :memberships end class Membership < ApplicationRecord belongs_to :user belongs_to :group end
By defining the through option, Rails allows us to access the groups that a user is a part of directly using the user.groups association.
Self-Referential Associations
Self-referential associations occur when a model has a relationship with itself. This type of association is commonly used in scenarios like hierarchical structures or friend/follower relationships. Let’s consider an example of a User model with a self-referential association called friends.
ruby class User < ApplicationRecord has_many :friendships has_many :friends, through: :friendships has_many :inverse_friendships, class_name: 'Friendship', foreign_key: 'friend_id' has_many :inverse_friends, through: :inverse_friendships, source: :user end class Friendship < ApplicationRecord belongs_to :user belongs_to :friend, class_name: 'User' end
In this example, a user can have many friends, and the inverse association allows us to retrieve the users who consider the current user as their friend.
Conclusion
Associations and relationships play a vital role in building complex and interconnected applications with Ruby on Rails. Understanding the different types of associations, including one-to-one, one-to-many, many-to-many, polymorphic, through, and self-referential associations, empowers developers to efficiently manage their data models and query related records effortlessly.
By leveraging the power of ActiveRecord associations, Rails provides a seamless experience for managing relationships, abstracting away the complexities of SQL joins and enabling developers to focus on building great applications.
In this tutorial, we covered the fundamental concepts of associations in Rails, along with detailed examples for each type. Armed with this knowledge, you are now equipped to build sophisticated applications with rich relationships and leverage the full potential of Ruby on Rails. Happy coding!
Table of Contents
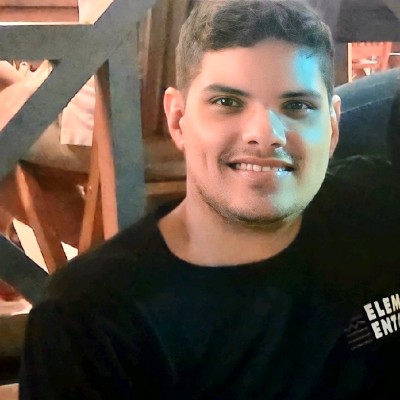
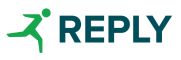