How to Use Ruby Functions for Audio and Video Manipulation
Ruby, a versatile and powerful programming language, is often celebrated for its elegant syntax and developer-friendly approach. Beyond its conventional usage in web development and automation, Ruby also excels in multimedia processing, including audio and video manipulation. In this blog, we will dive into the world of Ruby functions and explore how they can be leveraged to perform various audio and video tasks. Whether you are an aspiring developer or an experienced coder, understanding these techniques will undoubtedly enhance your multimedia projects and open up new creative possibilities.
Table of Contents
1. Getting Started with Ruby and Multimedia:
1.1. Installation and Setup:
To begin our multimedia journey with Ruby, make sure you have Ruby installed on your system. You can download and install Ruby from the official website (https://www.ruby-lang.org/). Additionally, we’ll need some multimedia libraries to handle audio and video processing.
For audio manipulation, we’ll use the “ruby-audio” gem. To install it, open your terminal and execute:
ruby gem install ruby-audio
For video manipulation, we’ll use the “ffmpeg” gem, which is a Ruby binding for the popular FFmpeg multimedia framework:
ruby gem install ffmpeg
1.2. Overview of Ruby Multimedia Libraries:
The “ruby-audio” gem provides a simple and easy-to-use interface for audio processing, supporting various audio formats and functionalities like audio playback, filtering, and feature extraction.
On the other hand, the “ffmpeg” gem offers powerful capabilities for video processing. It enables you to read and write video files, extract frames, apply filters, and much more. The FFmpeg library, which underpins this gem, is widely used in the multimedia industry due to its robustness and extensive codec support.
2. Audio Manipulation with Ruby Functions:
Ruby offers a wealth of functions for working with audio data, making it an excellent choice for audio processing tasks.
2.1. Reading and Writing Audio Files:
Let’s start by reading an audio file and writing it in a different format:
ruby require 'ruby-audio' input_file = "input.wav" output_file = "output.mp3" # Read the audio file input_info = RubyAudio::SoundInfo.new(input_file) input_sound = RubyAudio::Sound.open(input_file) # Write the audio in a different format output_info = RubyAudio::SoundInfo.new(output_file, 'w') output_sound = RubyAudio::Sound.open(output_file, 'w', output_info) # Transfer the audio data from the input file to the output file output_sound.write(input_sound.read)
2.2. Audio Playback and Basic Controls:
You can also play audio directly using the “ruby-audio” gem:
ruby require 'ruby-audio' file_path = "music.wav" # Open the audio file for playback RubyAudio::Sound.open(file_path) do |sound| # Start playing the audio sound.start_playback # Let it play for a few seconds sleep(5) # Pause the playback sound.pause_playback # Resume after a short pause sleep(2) # Stop the playback sound.stop_playback end
2.3. Audio Filtering and Effects:
Ruby offers a variety of signal processing functions that can be used to apply filters and effects to audio data. Let’s demonstrate how to apply a simple low-pass filter to an audio file:
ruby require 'ruby-audio' require 'numo/narray' def low_pass_filter(sound, cutoff_freq) nyquist = sound.info.samplerate / 2.0 num_taps = 51 taps = Numo::SFloat.new(num_taps).map { |i| (Math.sin(2 * Math::PI * cutoff_freq * (i - num_taps / 2)) / (i - num_taps / 2)) * (0.54 - 0.46 * Math.cos(2 * Math::PI * i / (num_taps - 1))) } sound.buffer = RubyAudio::Buffer.float(sound.info.channels, sound.info.frames) filtered_frames = sound.read sound.buffer.from_array(Numo::SFloat.cast(filtered_frames.to_a).map { |frame| Numo::FFTW.fft(frame).ifft(Numo::SComplex.cast(Numo::SFloat.cast(frame).fft * Numo::SFloat.cast(taps).fft).real).to_a }) sound end input_file = "input.wav" output_file = "output_filtered.wav" # Read the audio file input_info = RubyAudio::SoundInfo.new(input_file) input_sound = RubyAudio::Sound.open(input_file) # Apply the low-pass filter with a cutoff frequency of 5000 Hz filtered_sound = low_pass_filter(input_sound, 5000) # Write the filtered audio to a new file output_info = RubyAudio::SoundInfo.new(output_file, 'w', input_info.channels, input_info.samplerate) output_sound = RubyAudio::Sound.open(output_file, 'w', output_info) output_sound.write(filtered_sound.buffer.to_a)
2.4. Extracting Audio Features:
Ruby functions can also be utilized to extract various audio features, such as pitch, amplitude, and spectral characteristics. For example, let’s extract the root mean square (RMS) amplitude of an audio file:
ruby require 'ruby-audio' def calculate_rms_amplitude(sound) frames = sound.read sum_squared = frames.inject(0) { |sum, frame| sum + frame.map { |sample| sample ** 2 }.sum } Math.sqrt(sum_squared / frames.size.to_f) end file_path = "music.wav" # Open the audio file RubyAudio::Sound.open(file_path) do |sound| rms_amplitude = calculate_rms_amplitude(sound) puts "RMS Amplitude: #{rms_amplitude}" end
3. Video Manipulation with Ruby Functions:
Apart from audio, Ruby functions can be employed to process video data as well. The “ffmpeg” gem enables you to perform a wide range of video-related tasks.
3.1. Working with Video Files:
Let’s start by reading and writing video files using the “ffmpeg” gem:
ruby require 'ffmpeg' input_file = "input.mp4" output_file = "output.avi" # Read the video file movie = FFMPEG::Movie.new(input_file) # Write the video in a different format movie.transcode(output_file)
3.2. Video Playback and Frame Manipulation:
The “ffmpeg” gem also allows you to play video and manipulate individual frames:
ruby require 'gtk3' require 'ffmpeg' file_path = "video.mp4" Gtk.init window = Gtk::Window.new("Video Playback") window.signal_connect("destroy") { Gtk.main_quit } # Create a video player widget video_player = Gtk::Video.new # Read the video file movie = FFMPEG::Movie.new(file_path) # Set the video player source to the video file video_player.set_file(file_path) # Add the video player widget to the window window.add(video_player) window.show_all # Start playing the video video_player.play # Wait for the video to finish Gtk.main
3.3. Video Editing and Concatenation:
With Ruby functions and the “ffmpeg” gem, you can easily perform video editing tasks, such as trimming and concatenating videos:
ruby require 'ffmpeg' input_file_1 = "video1.mp4" input_file_2 = "video2.mp4" output_file = "output_concatenated.mp4" # Create movie objects for both input videos movie1 = FFMPEG::Movie.new(input_file_1) movie2 = FFMPEG::Movie.new(input_file_2) # Concatenate the videos concatenated_movie = FFMPEG::Movie.concat([movie1, movie2]) # Save the concatenated video to a new file concatenated_movie.transcode(output_file)
3.4. Extracting Video Frames:
Using Ruby functions, you can also extract frames from a video and save them as image files:
ruby require 'ffmpeg' input_file = "video.mp4" output_directory = "frames/" # Read the video file movie = FFMPEG::Movie.new(input_file) # Extract frames at specified intervals frame_interval = 5000 # milliseconds frame_number = 0 while (frame = movie.screenshot(frame_interval * frame_number)) frame.save("#{output_directory}frame_#{frame_number}.png") frame_number += 1 end
4. Combining Audio and Video: Creating Multimedia Magic:
Now that we know how to manipulate audio and video separately, let’s explore how to combine them for multimedia projects.
4.1. Adding Audio to Video:
To add audio to a video, we can leverage the “ffmpeg” gem to perform the task:
ruby require 'ffmpeg' video_file = "video.mp4" audio_file = "music.wav" output_file = "video_with_audio.mp4" # Create movie objects for both the video and audio video = FFMPEG::Movie.new(video_file) audio = FFMPEG::Movie.new(audio_file) # Combine the video and audio video.with_audio(audio).transcode(output_file)
4.2. Video with Subtitles: Synchronized Text Overlays:
Subtitles can enhance the viewer’s experience in videos. With Ruby functions and the “ffmpeg” gem, adding subtitles becomes straightforward:
ruby require 'ffmpeg' video_file = "video.mp4" subtitle_file = "subtitles.srt" output_file = "video_with_subtitles.mp4" # Create movie objects for the video and subtitle video = FFMPEG::Movie.new(video_file) subtitle = FFMPEG::Input.from_path(subtitle_file) # Combine the video and subtitle video.filter('subtitles', subtitle).output(output_file).run
4.3. Video Effects with Audio Reactivity:
You can create captivating video effects that synchronize with audio using Ruby functions. Here’s a simple example that increases the brightness of the video when the audio amplitude is high:
ruby require 'ffmpeg' video_file = "video.mp4" audio_file = "music.wav" output_file = "video_with_effects.mp4" # Create movie objects for the video and audio video = FFMPEG::Movie.new(video_file) audio = FFMPEG::Movie.new(audio_file) # Get the audio amplitude and normalize it between 0 and 1 max_amplitude = audio.audio_channels.map { |channel| channel.max }.max normalized_amplitude = audio.audio_channels.map { |channel| channel.max.to_f / max_amplitude } # Apply the brightness filter based on the audio amplitude video = video.filter('eq', "brightness=#{0.5 + normalized_amplitude[0]}") # Combine the video and audio video.with_audio(audio).transcode(output_file)
5. Advanced Techniques and Best Practices:
5.1. Real-time Audio and Video Processing:
For real-time audio and video processing, consider using libraries like “PortAudio” or “GStreamer” for audio and “GTK” or “Qt” for video. These libraries provide interfaces to interact with multimedia streams efficiently.
5.2. Performance Optimization:
When working with large multimedia files, optimize performance by using buffer streaming techniques and parallel processing for computationally intensive tasks.
5.3. Error Handling and Exception Management:
Ensure robustness in your multimedia projects by implementing proper error handling and exception management. Audio and video data processing may encounter various issues, such as unsupported formats or missing files.
Conclusion
Ruby’s vast array of functions and its ability to interface with powerful multimedia libraries make it an excellent choice for audio and video manipulation. From basic tasks like reading and writing multimedia files to more advanced techniques like synchronization and real-time processing, Ruby offers developers a flexible and creative playground. By exploring the world of Ruby functions for audio and video manipulation, you can unleash your creativity and elevate your multimedia projects to new heights. Happy coding and enjoy creating multimedia magic with Ruby!
Table of Contents
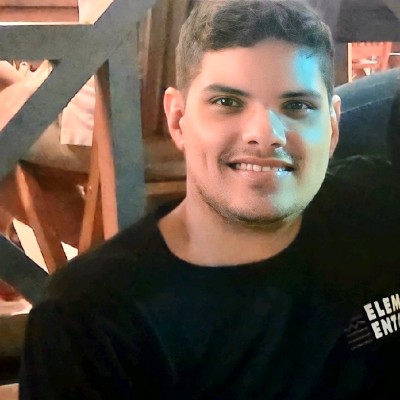
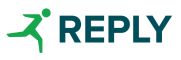