Ruby on Rails Q & A
What are the best practices for Rails code organization?
Organizing code efficiently in a Rails application is paramount to ensure maintainability, readability, and scalability. Here are the best practices for Rails code organization:
- Follow Rails Conventions: Rails has a strong emphasis on convention over configuration. Adhering to the default directory structure (e.g., models in `app/models`, controllers in `app/controllers`) simplifies codebase navigation and reduces the cognitive load for developers new to the project.
- Skinny Controllers, Fat Models: This principle advocates for keeping the controllers thin – primarily handling the HTTP layer, while the business logic resides within the models. Controllers should be used to handle user requests and render responses, while models should enforce business rules, validations, and data relations.
- Use Concerns: If a model or controller starts to grow large, consider using concerns to extract reusable pieces of code. Located in the `app/models/concerns` or `app/controllers/concerns` directories, they allow for modular and DRY code.
- Service Objects: For complex business logic that doesn’t neatly fit within a model, consider using service objects. These plain Ruby objects encapsulate specific business operations, keeping your models and controllers clean.
- Decorators & Presenters: If you find views or helpers becoming cluttered, use decorators or presenters. Tools like the Draper gem can assist in encapsulating view-specific logic.
- Avoid Callback Hell: While callbacks (e.g., `before_save`, `after_create`) can be handy, overusing them can make code unpredictable and hard to follow. Consider using service objects or form objects when the logic becomes convoluted.
- Namespacing: As your application grows, you might find related sets of models, controllers, or services. Organize these under namespaces. For instance, admin-related controllers might reside under an `Admin` namespace.
- Utilize Modules: For shared functionality across multiple classes, use modules. They can be mixed into classes as needed and promote DRY (Don’t Repeat Yourself) principles.
- Regular Refactoring: As the application evolves, always be on the lookout for opportunities to refactor. Tools like RuboCop can help in maintaining a consistent code style.
Keeping a Rails codebase organized requires a combination of adhering to Rails conventions and applying object-oriented design principles. By doing so, you’ll ensure that your application remains maintainable and scalable over time.
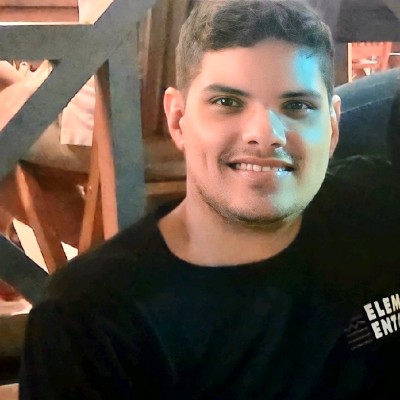
Previously at
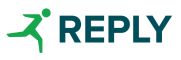
Senior Software Engineer with a focus on remote work. Proficient in Ruby on Rails. Expertise spans y6ears in Ruby on Rails development, contributing to B2C financial solutions and data engineering.