What are callbacks in Rails?
In Rails, callbacks are hooks that allow developers to attach custom methods (or “callbacks”) to be triggered during the lifecycle of an object. Specifically, they’re methods that get called at certain moments before or after key changes in the object’s lifecycle, such as before saving to the database, after updating an attribute, or even before an object is destroyed.
These callbacks can be extremely useful for encapsulating related behaviors or for ensuring that certain conditions are met before an object is saved. For instance, they can be used to normalize data before saving it, check the validity of related objects, or automatically timestamp an object when it’s created.
Commonly used callbacks include `before_save`, `after_save`, `before_create`, `after_create`, `before_update`, `after_update`, `before_destroy`, and `after_destroy`.
Here’s a simple example: imagine a `BlogPost` model where you want to ensure that the title is always title-cased before saving to the database. You could use a `before_save` callback as follows:
```ruby class BlogPost < ApplicationRecord before_save :titleize_title private def titleize_title self.title = title.titleize end end ```
In the above example, every time a `BlogPost` instance is saved, the `titleize_title` method will be triggered, ensuring the title attribute is in title case.
However, while callbacks are powerful, it’s crucial to use them judiciously. Overreliance on callbacks can make models complex and introduce unexpected behaviors, especially if callbacks interact with other parts of the application. It’s often a good practice to keep the callback methods small, single-responsibility, and easy to understand, ensuring that the application remains maintainable and predictable.
Callbacks in Rails are tools in the developer’s arsenal to inject custom behavior during an object’s lifecycle, but, like all tools, they should be used with care and understanding.
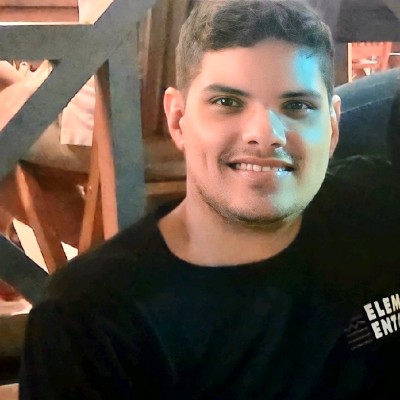
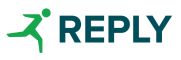