How to Use Ruby Functions for Chatbot Development
Chatbots have become an integral part of modern applications, enhancing user experiences and automating various tasks. While there are many programming languages to build chatbots, Ruby stands out as an excellent choice due to its simplicity, readability, and extensive community support. In this guide, we will delve into the world of Ruby functions and how you can leverage them to create robust chatbots. Whether you’re a seasoned Ruby developer or a beginner looking to explore chatbot development, this tutorial will provide you with the knowledge and tools you need to get started.
Table of Contents
1. Understanding the Basics
1.1. What Are Ruby Functions?
Before diving into chatbot development, let’s establish a fundamental understanding of Ruby functions, also known as methods. Functions in Ruby are reusable blocks of code that perform specific tasks. They take input, process it, and return an output. Functions encapsulate functionality, making code more organized and easier to maintain.
In Ruby, defining a function is straightforward:
ruby def greet(name) puts "Hello, #{name}!" end # Calling the function greet("Alice")
Here, the greet function takes one argument (name) and prints a greeting message with the provided name.
1.2. Advantages of Using Ruby Functions
Using Ruby functions in chatbot development offers several advantages:
- Modularity: Functions break down complex tasks into smaller, manageable pieces, making your chatbot code modular and easier to maintain.
- Reusability: Once defined, functions can be reused throughout your code, reducing redundancy and promoting efficient coding practices.
- Readability: Ruby’s syntax and naming conventions enhance code readability, making it easier to understand and collaborate on chatbot projects.
- Scalability: As your chatbot project grows, Ruby’s flexibility allows you to extend and enhance its functionality with ease.
Now that we’ve covered the basics, let’s explore how to apply Ruby functions in chatbot development.
2. Setting Up Your Chatbot Environment
2.1. Prerequisites
Before you begin, ensure you have Ruby installed on your system. You can download it from the official Ruby website.
2.2. Installing Dependencies
To build a chatbot, you’ll need a framework or library that simplifies interactions with messaging platforms. One popular choice is the telegram-bot-ruby gem, which enables you to create Telegram chatbots in Ruby.
Install the gem using RubyGems:
bash gem install telegram-bot-ruby
2.3. Creating Your First Chatbot
Let’s create a simple chatbot that responds to user messages with a greeting. We’ll define a Ruby function to handle incoming messages and send responses.
3. Setting Up Your Bot on Telegram
Open the Telegram app and search for the “BotFather” to create a new bot. Follow the instructions to obtain your bot’s API token.
Now, let’s write the Ruby code for our chatbot:
ruby require 'telegram/bot' # Replace 'YOUR_API_TOKEN' with your actual bot's API token Telegram::Bot::Client.run('YOUR_API_TOKEN') do |bot| bot.listen do |message| case message.text when '/start' bot.api.send_message(chat_id: message.chat.id, text: "Hello! I am your chatbot.") when '/stop' bot.api.send_message(chat_id: message.chat.id, text: "Goodbye!") else bot.api.send_message(chat_id: message.chat.id, text: "I didn't understand that.") end end end
In this code, we use the telegram-bot-ruby gem to create a Telegram bot. The bot listens to incoming messages and responds with predefined messages based on the user’s input.
4. Running Your Chatbot
Save the code to a file (e.g., simple_chatbot.rb) and run it using the command:
bash ruby simple_chatbot.rb
Your chatbot is now live on Telegram! You can interact with it by sending commands like /start and /stop.
5. Enhancing Your Chatbot with Ruby Functions
While our simple chatbot is functional, it’s just the beginning. Ruby functions can help you enhance its capabilities and make it more interactive.
5.1. Defining Custom Functions
Let’s create a custom function to respond to user greetings:
ruby def respond_to_greeting(message, bot) greetings = ['hi', 'hello', 'hey', 'hola'] if greetings.include?(message.downcase) bot.api.send_message(chat_id: message.chat.id, text: "Hello! How can I assist you?") end end
Now, modify the code inside the bot.listen block to include this function:
ruby bot.listen do |message| case message.text when '/start' bot.api.send_message(chat_id: message.chat.id, text: "Hello! I am your chatbot.") when '/stop' bot.api.send_message(chat_id: message.chat.id, text: "Goodbye!") else respond_to_greeting(message.text, bot) end end
With this custom function, your chatbot can respond to various greetings from users.
5.2. Handling User Input
To make your chatbot more interactive, you can define functions to handle specific user input. For example, if your chatbot provides weather updates, you can create a function like this:
ruby def get_weather(city) # Use a weather API to fetch weather data for the specified city # Parse the data and return a weather report end
Integrate this function into your chatbot to respond to user requests for weather updates in specific cities.
5.3. Storing User Data
You can use Ruby functions to store and retrieve user data. For instance, if your chatbot requires user preferences or settings, you can define functions to save and retrieve this data:
ruby def save_user_preference(user_id, preference) # Store user preference in a database or file end def get_user_preference(user_id) # Retrieve user preference from the database or file end
By implementing these functions, your chatbot can offer a personalized experience for users.
6. Best Practices for Chatbot Development in Ruby
As you continue to develop your chatbot using Ruby, consider these best practices:
6.1. Error Handling
Always include robust error handling in your code. Chatbots should gracefully handle unexpected user inputs and API failures.
ruby begin # Code that may raise an exception rescue StandardError => e # Handle the exception (e.g., log it or send an error message to the user) end
6.2. Regular Updates
Keep your chatbot up to date with the latest Ruby libraries and dependencies. Regular updates ensure that your chatbot remains secure and reliable.
6.3. Testing
Write unit tests to verify the functionality of your Ruby functions. Testing helps identify and fix issues early in the development process.
6.4. User Privacy
Respect user privacy and data security. Handle user data with care and comply with relevant privacy regulations.
Conclusion
Ruby functions are powerful tools for chatbot development, enabling you to create interactive and efficient chatbots. With the right functions in place, your chatbot can handle various user interactions, respond intelligently, and provide a seamless experience. As you explore the world of chatbot development in Ruby, don’t forget to stay updated with the latest trends and continuously improve your bot’s functionality. Happy coding!
Table of Contents
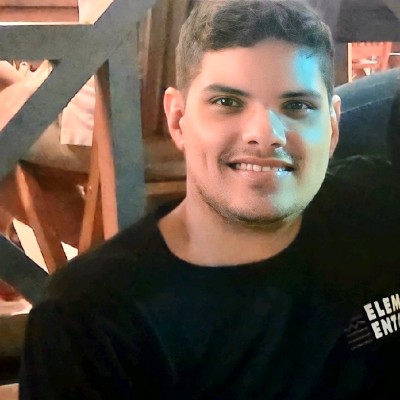
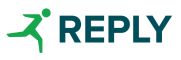