10 Common Ruby Functions Every Beginner Should Know
As a beginner in programming, getting acquainted with the core functions of a language forms a crucial part of the learning curve. Today, we will explore Ruby, a dynamic, open-source programming language with a focus on simplicity and productivity. Here are ten common Ruby functions that every beginner should know.
1. Print and Puts
To begin with, let’s start with the basics. ‘print’ and ‘puts’ are two commonly used functions in Ruby for outputting to the console. The difference? ‘puts’ adds a new line at the end of its output, while ‘print‘ does not.
puts "Hello, World!" print "Hello, World!"
2. Length
The ‘length’ method returns the number of elements in an array of characters in a string. It’s a handy function for quickly determining the size of your data.
array = [1, 2, 3, 4, 5] puts array.length # Outputs: 5 string = "Hello, World!" puts string.length # Outputs: 13
3. Split
The ‘split’ method is utilized to divide a string into an array of substrings based on a delimiter, which can be a space, comma, or any other character.
string = "Hello, World!" array = string.split(",") # Outputs: ["Hello", " World!"]
4. Join
The ‘join’ method does the reverse of ‘split’. It merges the elements of an array into a single string, separated by a given delimiter.
array = ["Hello", "World!"] string = array.join(" ") # Outputs: "Hello World!"
5. Map
The ‘map’ method is a powerful function in Ruby that allows you to transform an array. It applies a given block of code to each element in the array and returns a new array with the results.
array = [1, 2, 3, 4, 5] new_array = array.map {|num| num * 2} # Outputs: [2, 4, 6, 8, 10]
6. Select
The ‘select’ method in Ruby allows you to filter elements from an array that meet specific criteria. It executes the provided block of code for each element and returns a new array containing elements for which the block returned true.
array = [1, 2, 3, 4, 5] new_array = array.select {|num| num.even?} # Outputs: [2, 4]
7. Each
The ‘each’ method in Ruby is used to iterate over each item in an array or a hash. Unlike ‘map’ and ‘select’, it doesn’t return a new array, but simply executes the block of code for each element.
array = [1, 2, 3, 4, 5] array.each {|num| puts num} # Outputs each number on a new line
8. Sort
The ‘sort’ method is used to organize the elements in an array in a specific order. By default, ‘sort’ orders elements in ascending order. However, you can customize this by providing a block of code.
array = [5, 2, 3, 1, 4] sorted_array = array.sort # Outputs: [1, 2, 3, 4, 5]
9. Gsub
The ‘gsub’ method stands for Global Substitution. It replaces all occurrences of a specified substring or pattern within a string with a replacement string or result of a block if provided.
string = "Hello, World!" new_string = string.gsub("World", "Ruby") # Outputs: "Hello, Ruby!"
10. Include?
Finally, the ‘include?’ method checks whether a specified element exists in an array or substring exists in a string. It returns true if the element or substring is found, and false otherwise.
array = [1, 2, 3, 4, 5] puts array.include?(3) # Outputs: true string = "Hello, World!" puts string.include?("Ruby") # Outputs: false
These are just the tip of the iceberg in terms of the rich functionality that Ruby provides. However, they are some of the most frequently used and versatile functions that will greatly assist you in your coding journey. As you become more comfortable with these, explore the Ruby documentation for more methods and delve deeper into the world of programming with Ruby.
Conclusion
In conclusion, mastering these ten common Ruby functions forms an integral part of any beginner’s Ruby programming journey. They provide a fundamental understanding of the language’s functionality and capabilities, allowing for an efficient and productive programming experience. From basic output functions like ‘print’ and ‘puts’ to more complex methods like ‘map’ and ‘gsub‘, these tools equip beginners to effectively manipulate and handle data in Ruby.
As you gain proficiency in these core functions, it opens the door to more advanced programming concepts, ultimately enhancing your coding skill set. Remember, practice is key in mastering any programming language, so keep experimenting and applying these functions in your code. The world of Ruby programming awaits!
Table of Contents
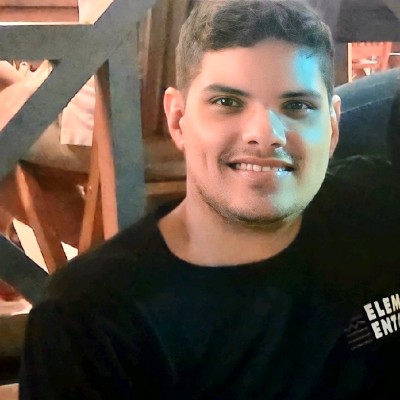
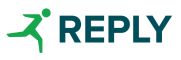