Ruby on Rails Tutorial: Understanding Rails Console and IRB
Ruby on Rails is a popular web application framework that makes web development efficient and enjoyable. As a Rails developer, you’ll often find yourself working with the Rails Console and IRB (Interactive Ruby) to test code, debug issues, and interact with your application’s data. In this tutorial, we’ll delve into the fundamentals of Rails Console and IRB and explore how they can boost your productivity during the development process.
1. What is Rails Console?
Rails Console is an essential tool for Ruby on Rails developers. It provides an interactive command-line interface that allows you to interact with your Rails application in real-time. Think of it as a sandbox environment where you can test and run Ruby code directly within your application’s context.
To launch the Rails Console, open your terminal and navigate to the root directory of your Rails application. Then, simply type rails console or rails c, and hit Enter. Within seconds, you’ll be presented with the Rails Console prompt, where you can start executing Ruby code and exploring your application’s models and data.
2. Getting Familiar with IRB (Interactive Ruby)
Before diving deep into the Rails Console, let’s briefly touch upon IRB (Interactive Ruby). IRB is a standalone Ruby shell that enables you to experiment with Ruby code outside the context of your Rails application. It serves as an excellent tool for learning Ruby syntax and experimenting with code snippets.
To launch IRB, open your terminal and type irb. You’ll enter the IRB prompt, where you can start typing Ruby code and observe the results instantly. While IRB is not specific to Rails, it shares similarities with Rails Console, making it a useful tool to practice your Ruby skills.
3. Rails Console: A Developer’s Best Friend
3.1. Working with Your Application’s Data
One of the primary use cases of Rails Console is to interact with your application’s data. You can query the database, create, update, or delete records, and perform various data-related operations with ease.
Let’s say you have a “User” model in your Rails application, and you want to fetch all users whose names start with “John.” You can achieve this with the following Rails Console command:
ruby john_users = User.where("name LIKE ?", "John%")
The Rails Console allows you to experiment with such queries in real-time, providing a convenient way to test complex data retrieval logic before incorporating it into your application code.
3.2. Debugging with Ease
Rails Console also serves as an excellent tool for debugging purposes. When you encounter an issue in your application, you can use the Rails Console to investigate the problem interactively.
For example, if you’re facing an unexpected behavior related to a specific user, you can examine the user’s attributes, associations, and related data directly within the console. This hands-on approach can save you valuable time in understanding and resolving the issue.
ruby user = User.find_by(email: "john@example.com") puts user.inspect puts user.posts.inspect
3.3. Manipulating Data in the Database
Sometimes, you might need to perform bulk updates on your data or modify certain records based on specific criteria. With Rails Console, you can easily achieve this.
Let’s say you want to update the email domain for all users whose emails end with “@old_domain.com” to “@new_domain.com.” You can use the following Rails Console command:
ruby User.where("email LIKE ?", "%@old_domain.com").update_all("email = REPLACE(email, '@old_domain.com', '@new_domain.com')")
Rails Console empowers you to interact with your application’s data intelligently and execute operations efficiently.
4. Rails Console Tips and Tricks
4.1. Reloading the Console
As you make changes to your Rails application code, you’ll need to reload the Rails Console to reflect those modifications. You can do this by typing reload! within the Rails Console prompt.
4.2. Shortcut for Last Result
In Rails Console, you can access the result of the last executed command by typing an underscore _. This feature comes in handy when you want to use the output of one command as an argument for the next.
For instance, if you find a user with the name “John” and want to assign them a new role, you can do the following:
ruby user = User.find_by(name: "John") user.update(role: "admin")
Or, using the shortcut:
ruby User.find_by(name: "John").update(role: "admin")
4.3. Sandbox Mode
In Rails Console, you’re working with live data from your development database. However, sometimes you might want to test code without altering the data. To do this, you can enter the Rails Console in sandbox mode by typing rails console –sandbox or rails c -s.
In sandbox mode, any changes you make to the data won’t be saved to the database when you exit the console.
Conclusion
In this tutorial, we’ve explored the significance of Rails Console and IRB in the Ruby on Rails development environment. The Rails Console offers a powerful way to interact with your application’s data, test code, and debug issues seamlessly. IRB, on the other hand, allows you to experiment with Ruby outside of your Rails application’s context.
By mastering these tools, you’ll enhance your productivity and gain valuable insights into your application’s behavior. Whether you’re a seasoned Rails developer or just getting started, incorporating Rails Console and IRB into your workflow can significantly boost your development skills and efficiency. So, start experimenting, exploring, and leveraging the full potential of these indispensable tools in your Ruby on Rails journey!
Table of Contents
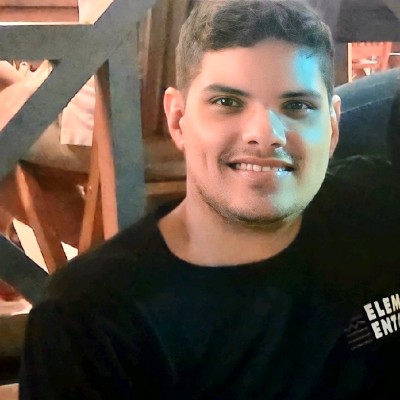
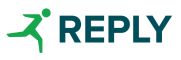