Ruby on Rails Tutorial: Understanding Cookies and Sessions
Ruby on Rails, often simply referred to as Rails, is a powerful web application framework known for its elegance and developer-friendly features. One crucial aspect of web development is managing user data and state, and that’s where cookies and sessions come into play. In this tutorial, we’ll explore the fundamentals of cookies and sessions in Ruby on Rails, including how they work, when to use them, and best practices for secure and efficient web applications.
Table of Contents
1. What Are Cookies and Sessions?
Before diving into the implementation details, let’s clarify what cookies and sessions are:
1.1. Cookies
Cookies are small pieces of data sent from a server and stored on the user’s device. They are typically used to remember information about the user, such as login credentials, shopping cart items, or user preferences. Cookies are sent with each HTTP request, allowing the server to recognize the user and provide a personalized experience.
1.2. Sessions
Sessions are similar to cookies but are stored on the server rather than the client’s device. A session is created for each user when they visit a website and is identified by a unique session ID. Session data can store a wide range of information, making it a secure and versatile option for managing user state.
Now that we have a basic understanding of cookies and sessions, let’s see how we can work with them in a Ruby on Rails application.
2. Working with Cookies in Rails
2.1. Setting Cookies
In Rails, setting cookies is straightforward. You can use the cookies object provided by the framework to set a cookie with a name and a value. Here’s an example:
ruby # Set a cookie cookies[:user_id] = 42
In this example, we’re setting a cookie named user_id with a value of 42. This value can be any object, but it’s common to use strings or numbers.
2.2. Reading Cookies
Reading cookies is just as simple as setting them. You can access cookies using the cookies object:
ruby # Read a cookie user_id = cookies[:user_id]
Here, we’re retrieving the value of the user_id cookie that we previously set. If the cookie doesn’t exist, user_id will be nil.
2.3. Deleting Cookies
To delete a cookie, you can use the delete method on the cookies object:
ruby # Delete a cookie cookies.delete(:user_id)
This code will remove the user_id cookie from the user’s browser.
3. Managing Sessions in Rails
Sessions are an excellent choice when you need to store user-specific data that should be kept secure and persistent throughout the user’s interaction with your application. Here’s how you can work with sessions in Ruby on Rails:
3.1. Setting Up Sessions
Ruby on Rails makes it effortless to set up sessions. By default, Rails uses cookies to store the session data. To enable sessions, you need to configure them in your application. In your config/application.rb file, you can find the following line:
ruby # config/application.rb config.session_store :cookie_store, key: '_your_app_session'
This line configures Rails to use the cookie store for sessions and sets the session key. You can customize the key to suit your application’s needs.
3.2. Accessing Session Data
Once sessions are set up, you can easily access session data using the session object in your controllers. For example:
ruby # Storing data in a session session[:user_id] = 42 # Retrieving data from a session user_id = session[:user_id]
In this code, we’re storing and retrieving the user’s ID in the session. This data will persist across multiple requests as long as the session is active.
3.3. Clearing Sessions
Clearing a session is as simple as setting its values to nil or using the reset_session method:
ruby # Clearing a session session[:user_id] = nil # or reset_session
These methods will effectively clear all session data, providing a clean slate for the user.
4. Security Considerations
When working with cookies and sessions, security should be a top priority. Here are some key security considerations to keep in mind:
4.1. Secure Cookies
To ensure the security of your cookies, you can mark them as secure and HttpOnly. Secure cookies are only transmitted over HTTPS, reducing the risk of interception, while HttpOnly cookies cannot be accessed via JavaScript, protecting them from cross-site scripting (XSS) attacks.
ruby # Secure and HttpOnly cookie cookies[:user_id] = { value: 42, secure: true, httponly: true }
4.2. Session Storage
If your application handles sensitive data, consider using a server-based session store instead of the default cookie store. This ensures that session data remains on the server and is not exposed to the client.
4.3. Cross-Site Request Forgery (CSRF) Protection
Rails provides built-in protection against CSRF attacks. Ensure that you use the protect_from_forgery method in your controllers to guard against malicious requests.
ruby # Enable CSRF protection protect_from_forgery with: :exception
6. Best Practices
To ensure smooth and secure operations when working with cookies and sessions in Ruby on Rails, here are some best practices to follow:
6.1. Use Cookies for Non-sensitive Data
Cookies are suitable for storing non-sensitive data, such as user preferences or shopping cart items. Avoid storing sensitive information like passwords or credit card details in cookies.
6.2. Encrypt Sensitive Session Data
If you need to store sensitive data in sessions, make sure to encrypt it before storing it in the session. Rails provides encryption mechanisms to help you achieve this.
6.3. Implement Session Timeout
Set a reasonable session timeout to ensure that inactive sessions are cleared. This reduces the risk of unauthorized access to a user’s session.
6.4. Regularly Rotate Session Tokens
For added security, consider regularly rotating session tokens. This makes it more challenging for attackers to hijack active sessions.
6.5. Keep Sessions Lightweight
Avoid storing excessive data in sessions, as this can impact performance. Store only what’s necessary for the session’s functionality.
Conclusion
Cookies and sessions are essential tools in web development, and Ruby on Rails provides a straightforward way to work with them. In this tutorial, we’ve explored the basics of cookies and sessions in Rails, including how to set and read cookies, manage sessions, and address security concerns.
By following best practices and understanding when to use cookies or sessions, you can build secure and efficient web applications that provide a seamless user experience. Now that you have a solid foundation, go ahead and implement cookies and sessions in your Rails projects with confidence. Your users will thank you for it. Happy coding!
Table of Contents
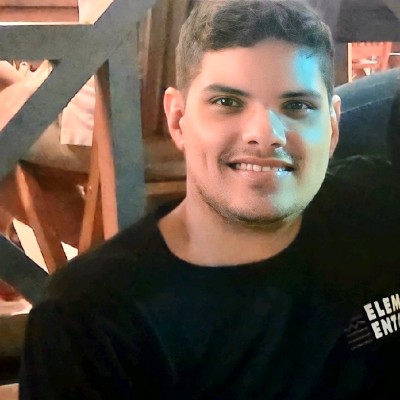
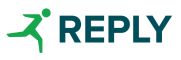