How to create custom validators in Rails?
In Rails, while there are several built-in validators for common tasks, there are situations where you’ll need custom validation logic. Creating custom validators in Rails is both straightforward and flexible.
- Custom Validator Class:
The most structured way to create a custom validator is by defining a new validator class. This class should inherit from `ActiveModel::Validator`.
```ruby class MyCustomValidator < ActiveModel::Validator def validate(record) unless record.attribute_meets_condition? record.errors[:attribute_name] << "Error message." end end end ```
You can then use this validator in your model:
```ruby class MyModel < ApplicationRecord validates_with MyCustomValidator end ```
- Inline Custom Validators:
For simpler validation logic, Rails allows you to define validations directly in the model using the `validate` method:
```ruby class MyModel < ApplicationRecord validate :my_custom_validation_method def my_custom_validation_method errors.add(:attribute_name, "Error message.") unless attribute_meets_condition? end end ```
- Custom Validator Modules:
If you want to reuse a validation logic across multiple models, you can create custom validators as modules:
```ruby module MyValidator extend ActiveSupport::Concern included do validate :my_custom_validation_method end def my_custom_validation_method errors.add(:attribute_name, "Error message.") unless attribute_meets_condition? end end class MyModel < ApplicationRecord include MyValidator end ```
- Error Messages:
When adding errors in custom validators, you can also utilize Rails’ I18n features for internationalization of error messages. This ensures your application remains flexible for multiple locales.
Rails provides versatile tools for creating custom validation logic. Whether you choose to implement a dedicated validator class, an inline method, or a reusable module, you have the power to ensure your data integrity with ease and maintainability.
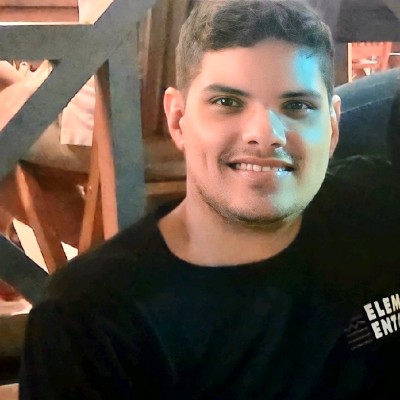
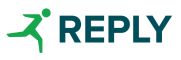