How to handle custom routes in Rails?
In a Rails application, routing determines how URLs are mapped to actions in controllers. While Rails provides conventional routes for resources via the `resources` method, there are cases where you’ll need custom routes to handle unique requirements.
- Defining Custom Routes: Custom routes can be added in the `config/routes.rb` file. To map a URL to a specific controller action, you can use:
```ruby get 'my_page', to: 'controller_name#action_name' ```
In this example, a GET request to `/my_page` would be directed to the specified action within the controller.
- Route Parameters: You can capture parts of the URL as parameters:
```ruby get 'users/:id', to: 'users#show' ```
In this example, visiting `/users/5` would send the request to the `show` action of the `UsersController` with `params[:id]` set to `5`.
- Named Routes: Naming routes can simplify path generation and provide more semantic code:
```ruby get 'login', to: 'sessions#new', as: 'login' ```
You can then use `login_path` or `login_url` in both your views and controllers to refer to this route.
- HTTP Verb Constraints: While `get` is commonly used, Rails supports all HTTP verbs (`get`, `post`, `put`, `patch`, `delete`). Choose the appropriate verb to match the action’s purpose.
- Advanced Constraints: Rails routing allows for constraints based on the request properties. For example, constraining routes to specific subdomains or request methods:
```ruby constraints(subdomain: 'admin') do get 'dashboard', to: 'admin#dashboard' end ```
- Scoping and Namespacing: For grouping routes or applying shared constraints, `scope` and `namespace` can be used. For example, to group admin-related routes under an `admin` namespace:
```ruby namespace :admin do resources :users end ```
Rails provides a powerful and flexible routing system. While the conventional routes cater to many use-cases, custom routes offer the needed granularity for specific needs, ensuring URLs remain intuitive and your application remains organized.
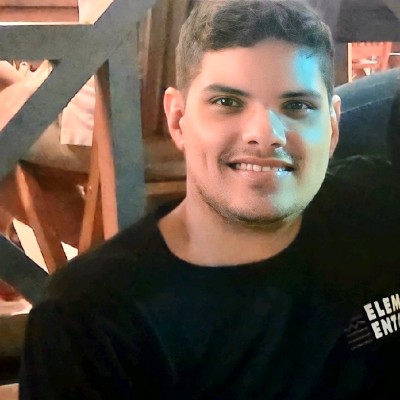
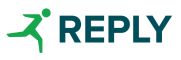