How to Use Ruby Functions for Data Encryption and Decryption
In the digital age, data security has become a paramount concern. Whether it’s sensitive user information or confidential business data, safeguarding it from prying eyes and potential threats is essential. One of the most effective ways to protect data is through encryption and decryption. Ruby, a versatile and powerful programming language, offers robust functionalities for data encryption and decryption. In this blog, we’ll explore how to leverage Ruby functions to secure your data and ensure it remains confidential and protected.
Table of Contents
1. Understanding Data Encryption and Decryption
Data encryption is the process of converting plaintext into a ciphertext, making it unintelligible to unauthorized users. Decryption, on the other hand, is the reverse process of converting the ciphertext back into plaintext. The encryption and decryption processes involve the use of cryptographic keys, which serve as the foundation for secure communication.
In Ruby, you can utilize various encryption algorithms and techniques to secure your data. Let’s dive into setting up Ruby for data encryption.
2. Setting Up Ruby for Encryption
Before we proceed with data encryption and decryption, ensure you have Ruby installed on your system. Ruby has built-in libraries, such as OpenSSL and Digest, that facilitate encryption tasks.
To check your Ruby version, open your terminal and type:
ruby ruby --version
Make sure you have Ruby 2.0 or above to take advantage of the latest encryption functionalities.
3. Symmetric Encryption with Ruby
Symmetric encryption uses a single secret key for both encryption and decryption. It’s a faster encryption method and is well-suited for scenarios where the same key can be kept secure between the communicating parties.
3.1 Generating a Secret Key
In Ruby, you can use the OpenSSL library to generate a random secret key for symmetric encryption. Here’s an example of how to generate a 256-bit AES secret key:
ruby require 'openssl' def generate_secret_key key = OpenSSL::Cipher.new('AES-256-CBC').random_key File.write('secret.key', key) end
The above function will generate a secret key using the AES-256-CBC algorithm and save it to a file named secret.key.
3.2 Encrypting Data
Once you have the secret key, you can use it to encrypt your data. Here’s a sample function to encrypt data using symmetric encryption:
ruby require 'openssl' def symmetric_encrypt(data, secret_key) cipher = OpenSSL::Cipher.new('AES-256-CBC') cipher.encrypt cipher.key = secret_key encrypted_data = cipher.update(data) + cipher.final encrypted_data end
In this example, the symmetric_encrypt function takes the data to be encrypted and the secret key as inputs. It uses the AES-256-CBC cipher to perform the encryption.
3.3 Decrypting Data
To decrypt the data, you’ll need the same secret key used for encryption. Here’s a function to decrypt data using symmetric encryption:
ruby require 'openssl' def symmetric_decrypt(encrypted_data, secret_key) cipher = OpenSSL::Cipher.new('AES-256-CBC') cipher.decrypt cipher.key = secret_key decrypted_data = cipher.update(encrypted_data) + cipher.final decrypted_data end
The symmetric_decrypt function takes the encrypted data and the secret key as inputs and uses the AES-256-CBC cipher to decrypt the data.
4. Asymmetric Encryption with Ruby
Asymmetric encryption uses a pair of keys: a public key for encryption and a private key for decryption. This technique is slower compared to symmetric encryption but offers better security, especially when you need to communicate securely with multiple parties.
4.1 Generating Key Pairs
To use asymmetric encryption in Ruby, you need to generate a key pair consisting of a public key and a private key. Here’s how you can generate RSA key pairs:
ruby require 'openssl' def generate_key_pair key_pair = OpenSSL::PKey::RSA.new(2048) File.write('public_key.pem', key_pair.public_key.to_pem) File.write('private_key.pem', key_pair.to_pem) end
The above function generates a 2048-bit RSA key pair and saves the public key to public_key.pem and the private key to private_key.pem.
4.2 Encrypting Data using Public Key
Once you have the public key, you can use it to encrypt the data. Here’s a sample function for asymmetric encryption:
ruby require 'openssl' def asymmetric_encrypt(data, public_key) public_key = OpenSSL::PKey::RSA.new(File.read(public_key)) encrypted_data = public_key.public_encrypt(data) encrypted_data end
The asymmetric_encrypt function takes the data to be encrypted and the public key file path as inputs. It loads the public key, performs the encryption, and returns the encrypted data.
4.3 Decrypting Data using Private Key
To decrypt the data encrypted with the public key, you’ll need the corresponding private key. Here’s a function to decrypt data using asymmetric encryption:
ruby require 'openssl' def asymmetric_decrypt(encrypted_data, private_key) private_key = OpenSSL::PKey::RSA.new(File.read(private_key)) decrypted_data = private_key.private_decrypt(encrypted_data) decrypted_data end
The asymmetric_decrypt function takes the encrypted data and the private key file path as inputs. It loads the private key, performs the decryption, and returns the original data.
5. Hybrid Encryption: Combining Symmetric and Asymmetric Techniques
In some scenarios, using either symmetric or asymmetric encryption alone may not be sufficient. Hybrid encryption combines the best of both worlds to address such cases. In hybrid encryption, you first use asymmetric encryption to exchange a shared secret key securely, and then you use symmetric encryption to encrypt the actual data with the shared secret key.
Here’s an example of how to implement hybrid encryption in Ruby:
ruby require 'openssl' def hybrid_encrypt(data, public_key) # Step 1: Generate a random symmetric key secret_key = OpenSSL::Cipher.new('AES-256-CBC').random_key # Step 2: Encrypt the data using the symmetric key encrypted_data = symmetric_encrypt(data, secret_key) # Step 3: Encrypt the symmetric key using the recipient's public key encrypted_secret_key = asymmetric_encrypt(secret_key, public_key) { encrypted_data: encrypted_data, encrypted_secret_key: encrypted_secret_key } end def hybrid_decrypt(encrypted_data, encrypted_secret_key, private_key) # Step 1: Decrypt the symmetric key using the recipient's private key secret_key = asymmetric_decrypt(encrypted_secret_key, private_key) # Step 2: Decrypt the data using the symmetric key decrypted_data = symmetric_decrypt(encrypted_data, secret_key) decrypted_data end
The hybrid_encrypt function takes the data to be encrypted and the recipient’s public key file path. It generates a random symmetric key, encrypts the data using the symmetric key, and encrypts the symmetric key using the recipient’s public key. The function returns both the encrypted data and the encrypted symmetric key.
The hybrid_decrypt function takes the encrypted data, encrypted symmetric key, and the recipient’s private key file path. It first decrypts the symmetric key using the recipient’s private key and then decrypts the data using the symmetric key.
6. Salting and Hashing for Added Security
Apart from encryption, other techniques like salting and hashing can further enhance data security.
6.1 Salting
Salting is a technique used to protect passwords and prevent attackers from using precomputed tables (rainbow tables) for quick password recovery. In Ruby, you can use the bcrypt gem for salting passwords:
ruby require 'bcrypt' def create_salted_hash(password) salt = BCrypt::Engine.generate_salt hashed_password = BCrypt::Engine.hash_secret(password, salt) { salt: salt, hashed_password: hashed_password } end def authenticate(password, salt, hashed_password) hashed_attempt = BCrypt::Engine.hash_secret(password, salt) hashed_attempt == hashed_password end
The create_salted_hash function takes a password as input, generates a random salt, and then creates a hashed password using the salt. The function returns both the salt and the hashed password.
The authenticate function takes a password, the salt, and the hashed password as inputs. It hashes the provided password using the salt and checks if it matches the stored hashed password, enabling secure authentication.
6.2 Hashing
Hashing is a one-way encryption technique used to store sensitive data like passwords securely. Ruby’s Digest library provides various hashing algorithms:
ruby require 'digest' def hash_data(data) hashed_data = Digest::SHA256.hexdigest(data) hashed_data end
The hash_data function takes data as input and returns its SHA-256 hash. Note that hashing is a one-way process; you cannot reverse it to obtain the original data.
7. Best Practices for Data Encryption
While using Ruby functions for data encryption and decryption, keep the following best practices in mind:
- Always keep your encryption keys and private keys secure and never hardcode them within your code.
- Regularly update and rotate encryption keys to enhance security.
- Implement strong password hashing techniques like bcrypt to protect user passwords.
- Follow the principle of least privilege while handling encryption keys and user access to data.
- Always use up-to-date versions of Ruby and relevant libraries to leverage the latest security features.
Conclusion
Data encryption and decryption are critical components of modern data security strategies. Ruby offers a powerful set of functions and libraries to implement various encryption techniques effectively. In this blog, we explored symmetric and asymmetric encryption, hybrid encryption, salting, and hashing techniques in Ruby. By adopting best practices and understanding the nuances of encryption, you can ensure that your data remains secure and confidential, even in the face of potential threats. Remember to stay informed about the latest advancements in encryption technologies to stay ahead in the ever-evolving landscape of data security. Happy coding!
By understanding the power of Ruby functions for data encryption and decryption, you can secure your data with confidence. Learn the essential techniques, examples, and best practices for robust data handling in this comprehensive guide.
Table of Contents
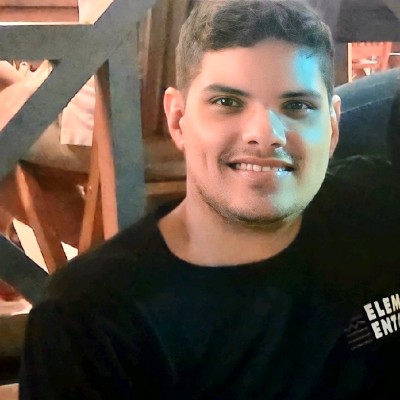
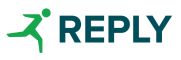