10 Ruby Gems for Data Visualization
Data visualization is a crucial aspect of data analysis and presentation. Whether you are working on a data-driven project, creating dashboards, or simply trying to understand your data better, having the right tools can make a significant difference. Ruby, a versatile programming language, offers a variety of gems (libraries) that can help you create stunning data visualizations. In this blog, we will explore 10 Ruby Gems for Data Visualization, complete with code samples to get you started on your data visualization journey.
Table of Contents
1. Chartkick
Simplify Chart Creation
Chartkick is a gem that makes it incredibly easy to create beautiful charts in your Ruby applications. It supports various charting libraries like Chart.js, Highcharts, and Google Charts, providing flexibility in choosing the charting tool that best suits your needs.
Here’s a quick example of how to create a basic line chart with Chartkick using the Highcharts library:
ruby # Gemfile gem 'chartkick' # app/controllers/my_controller.rb class MyController < ApplicationController def index @data = {'2023-01-01' => 5, '2023-01-02' => 8, '2023-01-03' => 12} end end
In your view:
erb <%= line_chart @data %>
Chartkick takes care of rendering the chart and ensures it’s responsive and visually appealing.
2. LazyHighCharts
Full Control Over Highcharts
If you want more control over your Highcharts visualizations, LazyHighCharts is an excellent choice. It provides a high-level interface for creating Highcharts charts in Ruby.
Here’s an example of creating a bar chart with LazyHighCharts:
ruby # Gemfile gem 'lazy_high_charts' # app/controllers/my_controller.rb class MyController < ApplicationController def index @chart = LazyHighCharts::HighChart.new('bar') do |f| f.title(text: 'Monthly Sales') f.xAxis(categories: ['January', 'February', 'March']) f.series(name: '2023', data: [10, 15, 8]) end end end
In your view:
erb <%= high_chart('my_container', @chart) %>
LazyHighCharts allows you to customize your Highcharts charts with ease.
3. Plotly
Interactive Graphs and Dashboards
Plotly is a gem that provides interactive data visualizations and dashboards. It supports a wide range of chart types and offers extensive customization options.
Here’s an example of creating a simple scatter plot with Plotly:
ruby # Gemfile gem 'plotly' # app/controllers/my_controller.rb class MyController < ApplicationController def index trace = { x: [1, 2, 3, 4], y: [10, 11, 12, 13], mode: 'markers', type: 'scatter' } layout = { xaxis: { title: 'X-axis' }, yaxis: { title: 'Y-axis' }, title: 'Scatter Plot' } @data = [trace] @layout = layout end end
In your view:
erb <%= plotly_chart(@data, layout: @layout) %>
Plotly’s interactivity makes it ideal for building data dashboards and exploring data in-depth.
4. C3-Rails
D3.js-Based Charts
C3-Rails is a gem that wraps the C3.js library, which is built on top of D3.js. It simplifies the creation of D3.js-based charts, making them more accessible to Ruby developers.
Here’s an example of creating a basic bar chart with C3-Rails:
ruby # Gemfile gem 'c3-rails' # app/controllers/my_controller.rb class MyController < ApplicationController def index @data = { bindto: '#chart', data: { columns: [ ['data1', 30, 200, 100, 400, 150, 250], ['data2', 50, 20, 10, 40, 15, 25] ], type: 'bar' } } end end
In your view:
erb <div id="chart"></div> <%= c3_chart(@data) %>
C3-Rails simplifies the integration of D3.js charts into your Ruby applications.
5. GnuplotRB
Scientific Plotting
GnuplotRB is a gem that allows you to create scientific plots using Gnuplot. It’s particularly useful for visualizing complex data in fields like physics, engineering, and data analysis.
Here’s an example of creating a simple 2D plot with GnuplotRB:
ruby # Gemfile gem 'gnuplotrb' # app/controllers/my_controller.rb require 'gnuplotrb' class MyController < ApplicationController def index x = (0..10).collect { |i| i.to_f } y = x.collect { |i| i**2 } plot = GnuplotRB::Plot.new( [ GnuplotRB::DataSet.new(x, y) do |ds| ds.with = 'linespoints' ds.title = 'y = x^2' end ], title: 'Simple 2D Plot', xlabel: 'X-axis', ylabel: 'Y-axis' ) @plot = plot end end
In your view:
erb <%= @plot.to_svg %>
GnuplotRB is an excellent choice for scientific and technical data visualization.
6. RGL – Ruby Graph Library
Visualize Graphs and Networks
RGL is a Ruby library for working with graphs and networks. While it’s not a traditional data visualization gem, it’s incredibly useful for visualizing graph data structures.
Here’s a simple example of how to create and visualize a directed graph with RGL:
ruby # Gemfile gem 'rgl' # app/controllers/my_controller.rb require 'rgl/dot' class MyController < ApplicationController def index graph = RGL::DirectedAdjacencyGraph[1, 2, 2, 3, 3, 1, 4, 1] File.open('graph.dot', 'w') do |f| f.puts graph.to_dot_graph end system('dot -Tpng graph.dot -o graph.png') @graph_image = 'graph.png' end end
In your view:
erb <%= image_tag @graph_image %>
RGL is indispensable for projects involving graph theory and network analysis.
7. Daru
Data Analysis and Visualization
Daru is a gem for data analysis and visualization in Ruby. It provides a DataFrame class that makes it easy to manipulate and visualize data.
Here’s a simple example of creating a bar chart with Daru:
ruby # Gemfile gem 'daru' # app/controllers/my_controller.rb require 'daru' require 'daru/view' class MyController < ApplicationController def index data = { category: ['A', 'B', 'C', 'D'], values: [10, 20, 15, 30] } df = Daru::DataFrame.new(data) df.plot(type: :bar, x: :category, y: :values, title: 'Bar Chart') end end
Daru simplifies data manipulation and visualization, making it a valuable tool for data scientists and analysts.
8. Gruff
Create Custom Graphs
Gruff is a gem that allows you to create custom graphs and charts in various formats, including PNG, SVG, and more. It provides a high level of customization for your charts.
Here’s an example of creating a line graph with Gruff:
ruby # Gemfile gem 'gruff' # app/controllers/my_controller.rb require 'gruff' class MyController < ApplicationController def index g = Gruff::Line.new g.title = 'Line Graph' g.labels = { 0 => 'Jan', 1 => 'Feb', 2 => 'Mar', 3 => 'Apr', 4 => 'May' } g.data('Example Data', [10, 23, 45, 32, 50]) g.write('line_graph.png') @line_graph = 'line_graph.png' end end
In your view:
erb <%= image_tag @line_graph %>
Gruff gives you fine-grained control over the appearance of your charts.
9. Google Charts
Harness the Power of Google Charts
Google Charts is a popular tool for creating interactive charts and graphs. The googlecharts gem allows you to seamlessly integrate Google Charts into your Ruby applications.
Here’s an example of creating a simple pie chart with Google Charts:
ruby # Gemfile gem 'googlecharts' # app/controllers/my_controller.rb class MyController < ApplicationController def index @data = GoogleVisualr::DataTable.new @data.new_column('string', 'Task') @data.new_column('number', 'Hours per Day') @data.add_rows([ ['Work', 11], ['Eat', 2], ['Commute', 2], ['Watch TV', 2], ['Sleep', 7] ]) @chart = GoogleVisualr::Interactive::PieChart.new(@data, { width: 400, height: 240, title: 'My Daily Activities' }) end end
In your view:
erb <%= render_chart @chart, 'my-chart' %>
Google Charts offers a wide range of chart types and interactivity options.
10. SVG::Graph
Create Scalable Vector Graphics
SVG::Graph is a gem that specializes in creating Scalable Vector Graphics (SVG) charts. SVG charts are ideal for web applications as they can be scaled without loss of quality.
Here’s an example of creating a bar chart with SVG::Graph:
ruby # Gemfile gem 'svg-graph' # app/controllers/my_controller.rb require 'svggraph' class MyController < ApplicationController def index data = { 'January' => 10, 'February' => 20, 'March' => 15, 'April' => 30 } graph = SVG::Graph::Bar.new(width: 300, height: 200, show_graph_title: true, key: true) graph.add_data(data) @svg_chart = graph.burn end end
In your view:
erb <%= raw @svg_chart %>
SVG::Graph is an excellent choice when you need scalable, vector-based charts.
Conclusion
Data visualization is a powerful tool for understanding and presenting data effectively. These 10 Ruby Gems provide a wide range of options for creating stunning data visualizations, whether you need simple charts, interactive dashboards, or complex scientific plots. Choose the gem that best suits your project’s requirements and start visualizing your data like a pro. Happy coding!
Table of Contents
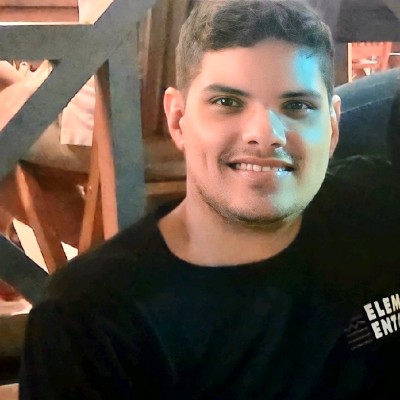
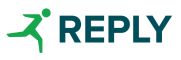