How to Use Ruby Functions for Date and Time Manipulation
Manipulating dates and times in your code is a task that you’ll encounter almost invariably while building software applications. In Ruby, several powerful tools and functions make this process straightforward. This post will delve into using Ruby functions for effective date and time manipulation.
Getting Started: Date and Time in Ruby
Before we dig deep into manipulation, it’s crucial to understand that Ruby handles date and time with distinct classes: `Date`, `Time`, and `DateTime`.
– `Date`: The `Date` class is for working with dates only. It doesn’t handle time or timezone.
– `Time`: The `Time` class handles both time and date, and it also considers system timezones.
– `DateTime`: The `DateTime` class is a subclass of `Date` that easily handles both dates and times.
To use these classes, you have to require them in your script:
require 'date' require 'time'
Current Date and Time
To get the current date or time, you use the `now` method for the `Time` class, and the `today` method for the `Date` class:
puts Time.now # 2023-06-21 15:30:00 +0300 puts Date.today # 2023-06-21
Creating a Specific Date or Time
Ruby allows you to create specific dates or times using the `new` method:
date = Date.new(2023, 6, 21) puts date # 2023-06-21 time = Time.new(2023, 6, 21, 15, 30, 0, "+03:00") puts time # 2023-06-21 15:30:00 +0300
In the `Time.new` method, the seventh parameter represents the timezone offset from UTC.
Parsing Dates and Times
When dealing with date or time data in string format, you’ll need to convert or parse it into a `Date` or `Time` object. You can achieve this with the `parse` method:
date = Date.parse('2023-06-21') puts date # 2023-06-21 time = Time.parse('2023-06-21 15:30:00') puts time # 2023-06-21 15:30:00 +0300
Formatting Dates and Times
Ruby offers the `strftime` method for formatting dates and times. The function takes a format string with directives represented by `%` followed by a character:
time = Time.now puts time.strftime('%Y-%m-%d %H:%M:%S') # 2023-06-21 15:30:00
Here, `%Y` represents the four-digit year, `%m` the month, `%d` the day, `%H` the hour, `%M` the minute, and `%S` the second.
Adding and Subtracting Time
Ruby’s `Date` and `Time` classes provide several methods to perform arithmetic operations:
date = Date.new(2023, 6, 21) puts date + 5 # 2023-06-26 puts date - 5 # 2023-06-16 time = Time.new(2023, 6, 21, 15, 30, 0, "+03:00") puts time + 60*60*24*5 # 2023-06-26 15:30: 00 +0300
Here, we add or subtract 5 days from a date. For time, since it’s based on seconds, we add or subtract seconds equivalent to 5 days (60 seconds x 60 minutes x 24 hours x 5 days).
Comparing Dates and Times
Comparison operators can be used to compare `Date` and `Time` objects:
date1 = Date.new(2023, 6, 21) date2 = Date.new(2023, 6, 22) puts date1 < date2 # true time1 = Time.new(2023, 6, 21, 15, 30, 0, "+03:00") time2 = Time.new(2023, 6, 21, 16, 30, 0, "+03:00") puts time1 < time2 # true
Using Time Zones
When creating `Time` objects, you can specify the timezone. However, the system’s local timezone is used if it’s not specified:
time = Time.new(2023, 6, 21, 15, 30, 0, "+03:00") puts time # 2023-06-21 15:30:00 +0300
The `DateTime` Class
The `DateTime` class is a subclass of `Date` that handles dates and times together. It’s not widely used because it doesn’t consider system timezones, but it’s useful when dealing with historical dates due to its broader range:
datetime = DateTime.new(2023, 6, 21, 15, 30, 0) puts datetime # 2023-06-21T15:30:00+00:00
Conclusion
Ruby provides robust tools and functions for date and time manipulation. These methods allow you to perform a multitude of tasks – from retrieving the current date or time to parsing, formatting, arithmetic operations, comparisons, and much more. With these tools in your Ruby arsenal, you’re well-equipped to handle any date and time tasks you encounter in your software projects.
Table of Contents
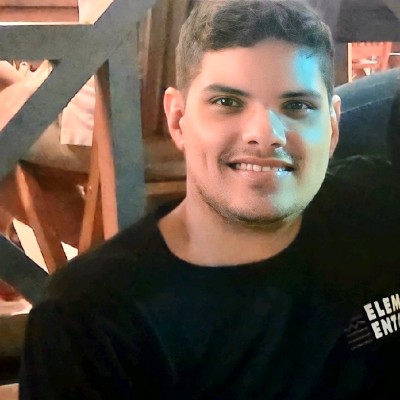
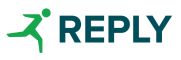