What are decorators in Rails?
Decorators in Rails are a design pattern used to extend the behavior of objects without modifying the actual object or its core logic. Instead of adding additional methods or logic to your model (which can clutter the model and make it less cohesive), you wrap it with a decorator that adds the new behavior. This approach is especially useful when you want to keep your models slim and only concerned with data-related logic, separating presentation and business logic.
A popular gem used in the Rails community for implementing decorators is `draper`. With Draper, each model can have a corresponding decorator which is responsible for adding view-specific logic to it.
For instance, consider a `User` model with attributes `first_name` and `last_name`. If you want a method that displays the user’s full name, instead of adding that method directly to the `User` model, you’d create a `UserDecorator` and define the method there. This way, the model remains lean and focused only on data persistence, while the decorator handles the presentation logic.
Usage with Draper might look something like this:
```ruby class UserDecorator < Draper::Decorator delegate_all def full_name "#{object.first_name} #{object.last_name}" end end ```
To use the decorator, you’d typically do something like:
```ruby @user = User.find(params[:id]).decorate @user.full_name # Uses the decorator's method ```
Decorators in Rails offer a way to better organize your codebase by ensuring that each class has a single responsibility. By separating presentation logic from data logic, decorators help maintain the integrity and simplicity of models while still providing rich functionality when needed.
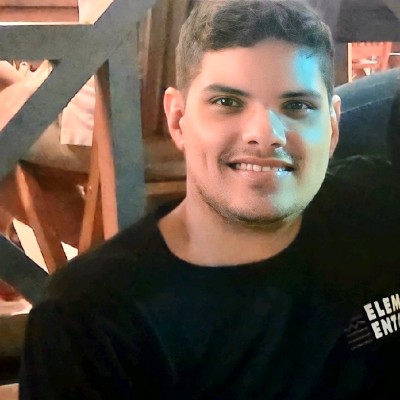
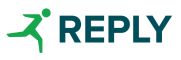