10 Ruby Gems for Deployment and Hosting
Deploying and hosting web applications can be a daunting task, especially for developers new to the process. Thankfully, the Ruby community offers a plethora of powerful gems to simplify deployment and hosting procedures. In this blog, we will explore ten essential Ruby gems that streamline your web application’s journey from development to production. These gems will enhance your development workflow, save time, and ensure a smooth deployment process, allowing you to focus on building exceptional web applications. Let’s dive in!
Table of Contents
1. Capistrano: Streamlined Deployment Automation
Simplify your deployment process with Capistrano
Capistrano is a popular deployment automation tool designed to make the deployment process more manageable. It allows developers to write deployment scripts in Ruby and execute them on remote servers. Capistrano’s strength lies in its ability to handle various tasks, including copying files, running database migrations, and restarting services. It also supports multi-stage deployments, enabling you to deploy to different environments (e.g., development, staging, production) effortlessly.
Installation:
ruby gem install capistrano Sample Deployment Script: ruby Copy code # config/deploy.rb set :application, 'my_app' set :repo_url, 'git@github.com:username/my_app.git' set :deploy_to, '/var/www/my_app' namespace :deploy do task :restart do on roles(:app) do # Restart the application server end end end
2. Heroku: Cloud Platform for Easy Deployment
Deploy and host your Ruby applications with ease on Heroku
Heroku is a cloud platform that simplifies deploying and hosting Ruby applications. With Heroku, you can push your application code using Git and let the platform handle the rest. It automatically scales your app, manages databases, and provides various add-ons for additional functionality. The ease of use and seamless integration with Git make Heroku an excellent choice for small to medium-sized projects.
Installation:
ruby gem install heroku Sample Deployment to Heroku: bash Copy code # Login to Heroku heroku login # Create a new Heroku app heroku create my_app # Deploy the application git push heroku master
3. Capistrano-Rails: Capistrano Tasks for Ruby on Rails
Effortless deployment of Ruby on Rails applications with Capistrano-Rails
Capistrano-Rails is an extension of Capistrano that provides specific tasks for deploying Ruby on Rails applications. It simplifies common Rails deployment tasks, such as asset precompilation, database migration, and running console commands on the server. Capistrano-Rails streamlines the deployment process for Rails projects, ensuring a smooth transition from development to production.
Installation:
ruby # Add to Gemfile gem 'capistrano-rails' # Install with bundler bundle install
Sample Deployment Script:
ruby # config/deploy.rb # Load Capistrano-Rails tasks require 'capistrano/rails' # …
4. Puma: High-Performance Web Server
Boost your application’s performance with Puma
Puma is a high-performance concurrent web server designed for Ruby applications. It can handle multiple requests concurrently, making it an excellent choice for applications with high traffic and demanding performance needs. Puma can be easily integrated into Ruby on Rails and other Rack-based applications, providing better response times and improved concurrency.
Installation:
ruby # Add to Gemfile gem 'puma' # Install with bundler bundle install
Configuring Puma:
ruby # config/puma.rb workers ENV.fetch('WEB_CONCURRENCY') { 2 } threads_count = ENV.fetch('RAILS_MAX_THREADS') { 5 } threads threads_count, threads_count preload_app! rackup DefaultRackup port ENV['PORT'] || 3000 environment ENV['RACK_ENV'] || 'development' on_worker_boot do # Worker specific setup end
5. Unicorn: Rack-Based Web Server
A reliable and flexible web server for Ruby applications
Unicorn is a popular and battle-tested web server for Rack-based applications. It is known for its stability and ability to handle multiple concurrent requests efficiently. Unicorn works well with Ruby on Rails and other Rack frameworks, providing a seamless integration experience. With its simple configuration and ease of use, Unicorn is a solid choice for hosting Ruby applications.
Installation:
ruby # Add to Gemfile gem 'unicorn' # Install with bundler bundle install
Configuring Unicorn:
ruby # config/unicorn.rb worker_processes 4 timeout 30 preload_app true before_fork do |server, worker| # Actions to perform before a worker is forked end after_fork do |server, worker| # Actions to perform after a worker is forked end
6. Capistrano-SSH-Kit: SSH Automation for Capistrano
Enhanced SSH automation with Capistrano-SSH-Kit
Capistrano-SSH-Kit is an essential gem to enhance the capabilities of Capistrano in handling SSH operations. It provides additional tasks and features for executing commands on remote servers, copying files securely, and managing SSH settings. Capistrano-SSH-Kit makes your deployment scripts more powerful and reliable.
Installation:
ruby # Add to Gemfile gem 'capistrano-sshkit' # Install with bundler bundle install
Sample Usage:
ruby # config/deploy.rb require 'capistrano/sshkit' # …
7. Whenever: Schedule Cron Jobs with Ease
Simplify cron job management with Whenever
Whenever is a Ruby gem that simplifies the process of defining and updating cron jobs. It provides a clear and easy-to-read DSL for defining recurring tasks in your Ruby application. With Whenever, you can schedule tasks to run at specific intervals, making it a valuable tool for automating repetitive tasks during deployment.
Installation:
ruby # Add to Gemfile gem 'whenever' # Install with bundler bundle install
Defining Cron Jobs:
ruby # config/schedule.rb every 1.day, at: '4:30 am' do rake 'my_app:task' end every 1.hour do runner 'MyModel.my_method' end
8. Nginx: High-Performance Web Server and Reverse Proxy
Optimize your Ruby app with Nginx
Nginx is a powerful web server and reverse proxy that can significantly improve your application’s performance and security. It excels at handling static assets and efficiently distributing requests to application servers like Puma or Unicorn. Nginx’s configuration is highly customizable, making it a top choice for deploying and hosting Ruby applications.
Installation:
bash # Install Nginx (depending on your OS) sudo apt-get install nginx # Ubuntu/Debian sudo yum install nginx # CentOS/RHEL
Sample Nginx Configuration:
nginx # /etc/nginx/sites-available/my_app upstream app { server unix:/var/www/my_app/shared/tmp/sockets/puma.sock; } server { listen 80; server_name my_app.com; root /var/www/my_app/public; location / { try_files $uri @app; } location @app { proxy_pass http://app; proxy_set_header Host $host; proxy_set_header X-Real-IP $remote_addr; proxy_set_header X-Forwarded-For $proxy_add_x_forwarded_for; } }
9. Rack-Cache: HTTP Caching for Rack Applications
Improve performance with Rack-Cache
Rack-Cache is a middleware that adds HTTP caching to your Rack-based application. Caching can significantly improve performance by reducing the load on the application server. Rack-Cache supports various caching strategies, including in-memory caching, disk caching, and caching with external stores like Memcached or Redis.
Installation:
ruby # Add to Gemfile gem 'rack-cache' # Install with bundler bundle install
Enabling Rack-Cache:
ruby # config.ru require 'rack/cache' use Rack::Cache, metastore: 'file:/var/cache/rack/meta', entitystore: 'file:/var/cache/rack/body'
10. Net-SSH: SSH Library for Ruby
Establish SSH connections programmatically with Net-SSH
Net-SSH is a versatile Ruby library for establishing SSH connections and executing commands on remote servers programmatically. It provides a simple and powerful API for SSH automation, making it a useful tool for deploying and managing applications in various environments.
Installation:
ruby gem install net-ssh
Sample Usage:
ruby require 'net/ssh' Net::SSH.start('example.com', 'deploy_user') do |ssh| result = ssh.exec!('ls -al') puts result end
Conclusion
In this blog, we explored ten powerful Ruby gems that streamline the deployment and hosting of web applications. From Capistrano’s automated deployment scripts to Heroku’s cloud platform, each gem plays a vital role in simplifying the development-to-production journey. Whether you need high-performance web servers like Puma or Unicorn, SSH automation with Capistrano-SSH-Kit, or the convenience of scheduling cron jobs with Whenever, these gems will undoubtedly enhance your development workflow. By leveraging these tools, you can focus more on building exceptional web applications and less on the complexities of deployment and hosting. Happy coding!
Table of Contents
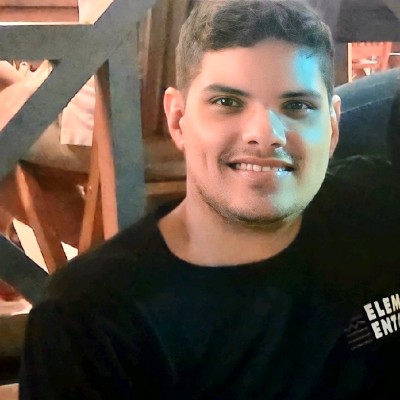
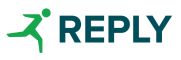