How to Use Ruby Functions for Email Sending and Receiving
Ruby is a powerful and elegant programming language that excels in simplicity and productivity. It has a rich ecosystem of libraries and frameworks, making it an excellent choice for various development tasks, including email handling. In this blog, we will explore how to use Ruby functions to streamline email sending and receiving processes. Whether you’re a beginner or an experienced Ruby developer, this guide will provide you with the knowledge and code samples to master email communications in your Ruby projects.
Table of Contents
1. Setting Up Your Ruby Environment:
Before we dive into email handling, ensure you have Ruby installed on your system. You can check the version using the following command in your terminal:
ruby ruby --version
If Ruby is not installed, download and install the latest version from the official website (https://www.ruby-lang.org/).
2. Sending Emails with Ruby Functions:
2.1. Configuring SMTP Settings:
To send emails, we need to set up Simple Mail Transfer Protocol (SMTP) settings. Ruby provides the “Net::SMTP” library to achieve this. Below is an example of configuring SMTP settings for Gmail:
ruby require 'net/smtp' def configure_smtp_settings Net::SMTP.start('smtp.gmail.com', 587, 'your_domain', 'your_email', 'your_password', :plain) do |smtp| smtp.enable_starttls_auto end end
Make sure to replace ‘your_domain’, ‘your_email’, and ‘your_password’ with your actual Gmail credentials. Additionally, if you’re using a different email service, modify the SMTP server and port accordingly.
2.2. Creating the Email:
Next, let’s create a function to compose the email using the “Mail” gem, a widely used library for email manipulation in Ruby:
ruby require 'mail' def create_email mail = Mail.new do from 'sender@example.com' to 'recipient@example.com' subject 'Hello from Ruby!' body 'This is the content of the email sent via Ruby function.' end return mail end
In this example, we set the sender’s email address, recipient’s email address, the subject of the email, and the body content.
2.3. Sending the Email:
Now that we have our email ready, let’s send it using the SMTP settings we configured earlier:
ruby def send_email(email) begin configure_smtp_settings email.deliver! puts 'Email sent successfully!' rescue StandardError => e puts "An error occurred: #{e.message}" end end email = create_email send_email(email)
The above code snippet first configures the SMTP settings and then sends the email using the “deliver!” method of the “Mail” gem. If everything is set up correctly, you should see a success message. If any errors occur during the process, the rescue block will catch them and display an error message.
3. Receiving Emails with Ruby Functions:
3.1. Receiving Emails via IMAP:
To receive emails, we use the Internet Message Access Protocol (IMAP). Ruby provides the “net/imap” library, which allows us to interact with the mail server and fetch emails. Let’s create a function to connect to the server and fetch the latest email:
ruby require 'net/imap' def fetch_latest_email imap = Net::IMAP.new('imap.example.com', 993, true) imap.login('your_email', 'your_password') imap.select('INBOX') latest_email = imap.search(['ALL']).last if latest_email email = imap.fetch(latest_email, 'RFC822')[0].attr['RFC822'] imap.store(latest_email, '+FLAGS', [:Seen]) # Mark the email as read return email end imap.logout end
Replace ‘imap.example.com’, ‘your_email’, and ‘your_password’ with your IMAP server, email credentials, and password, respectively. The function connects to the server, selects the INBOX, fetches the latest email using IMAP search, and marks it as read.
3.2. Processing Received Emails:
Now that we can fetch emails, let’s process them. We can use the “mail” gem again to parse and extract relevant information from the email:
ruby def process_email(email_content) mail = Mail.read_from_string(email_content) # Extract information from the email from = mail.from.first subject = mail.subject body = mail.body.decoded # Do something with the extracted data puts "Received an email from #{from} with subject: #{subject}" puts "Email Body:\n#{body}" end email_content = fetch_latest_email process_email(email_content)
The “process_email” function takes the email content fetched earlier and uses the “Mail.read_from_string” method to parse the email. We can then extract various details like sender, subject, and body from the email and perform actions based on the data.
3.3. Marking Emails as Read or Unread:
In the previous section, we marked the fetched email as read. However, you can also mark emails as unread or perform other actions, such as deleting or moving them to specific folders. Here’s how you can mark an email as unread:
ruby def mark_email_as_unread(email_uid) imap = Net::IMAP.new('imap.example.com', 993, true) imap.login('your_email', 'your_password') imap.select('INBOX') imap.store(email_uid, '-FLAGS', [:Seen]) # Mark the email as unread imap.logout end # Suppose you want to mark the second-to-last email as unread second_to_last_email_uid = imap.search(['ALL'])[-2] mark_email_as_unread(second_to_last_email_uid)
4. Building Email Communication Applications:
4.1. Creating a Newsletter Sender:
One practical application of email handling is creating a newsletter sender. Suppose you have a list of subscribers, and you want to send them regular newsletters. You can use Ruby functions to automate this process:
ruby require 'csv' def load_subscribers_from_csv(csv_file) subscribers = [] CSV.foreach(csv_file, headers: true) do |row| subscribers << row['email'] end return subscribers end def send_newsletter_to_subscribers(newsletter_content, subscribers) subscribers.each do |subscriber| email = Mail.new do from 'newsletter@example.com' to subscriber subject 'Monthly Newsletter' body newsletter_content end send_email(email) end end newsletter_content = 'Your monthly newsletter content here...' subscribers = load_subscribers_from_csv('subscribers.csv') send_newsletter_to_subscribers(newsletter_content, subscribers)
In this example, we load subscriber emails from a CSV file using the “load_subscribers_from_csv” function. Then, we iterate through the list of subscribers and send the newsletter to each of them.
4.2. Building an Email Ticketing System:
Another useful application is creating an email-based ticketing system to manage customer inquiries or support tickets. When customers send an email to a designated email address, the system automatically generates tickets and assigns them to appropriate staff:
ruby def create_ticket(sender, subject, body) # Logic to create a ticket in your system based on the email content end def process_ticket_email(email_content) mail = Mail.read_from_string(email_content) create_ticket(mail.from.first, mail.subject, mail.body.decoded) end # This function could be running as a daemon, continuously checking for new emails def email_ticketing_system loop do email_content = fetch_latest_email process_ticket_email(email_content) if email_content sleep(30) # Check for new emails every 30 seconds end end email_ticketing_system
The “process_ticket_email” function reads the email content and creates a ticket based on the sender, subject, and body. The “email_ticketing_system” function continuously checks for new emails, processes them, and creates tickets in your system accordingly.
5. Best Practices for Email Handling in Ruby:
5.1. Securing Email Credentials:
When handling email credentials, it’s essential to follow security best practices. Avoid hardcoding passwords in your code. Instead, use environment variables or a configuration file to store sensitive information securely.
5.2. Error Handling and Logging:
Robust error handling is crucial when dealing with email communications. Implement proper error handling and log any failures to help with troubleshooting and debugging.
5.3. Testing and Debugging:
Always thoroughly test your email functions to ensure they work as expected. Use debugging tools and log information to identify and fix issues effectively.
Conclusion:
In this blog, we explored how to use Ruby functions to simplify email sending and receiving processes. We covered configuring SMTP settings, composing and sending emails, fetching and processing received emails via IMAP, and creating practical email communication applications. By leveraging Ruby’s powerful libraries and frameworks, you can streamline email handling in your projects, improving communication with users and clients.
Email handling in Ruby provides endless possibilities, and this blog only scratched the surface. As you continue to explore the world of Ruby development, remember to follow best practices and continuously enhance your skills to create efficient and reliable email communication solutions.
Happy coding!
Table of Contents
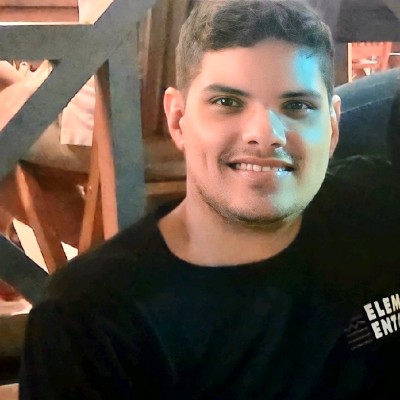
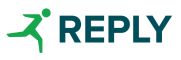