Ruby on Rails Tutorial: Understanding Rails Engines
Ruby on Rails is a robust web development framework known for its simplicity and elegance. One of the lesser-known yet powerful features of Rails is its Engines. Engines are mini-applications within a Rails application that can be extracted and reused in multiple projects, providing modularity, code reusability, and encapsulation. In this tutorial, we’ll explore the concept of Rails Engines, how they work, and how you can leverage them to build more maintainable and scalable web applications.
Table of Contents
1. What are Rails Engines?
Rails Engines are self-contained, modular components that act like mini-applications within a Rails application. They encapsulate specific functionality, such as authentication, file uploading, or payment processing, and can be mounted in multiple Rails applications, promoting code reuse and maintainability.
2. Advantages of Using Rails Engines
Using Rails Engines offers several benefits:
- Modularity: Engines allow you to break down a monolithic Rails application into smaller, manageable pieces, making development and maintenance easier.
- Code Reusability: The encapsulated functionality can be shared across multiple projects, saving development time and effort.
- Isolation: Engines can have their own routes, views, and assets, avoiding conflicts with the main application’s codebase.
- Testing: Engines can be unit-tested independently, ensuring robustness and reliability.
- Collaboration: Teams can work independently on different engines, promoting parallel development.
3. Creating a New Rails Engine
To create a new Rails Engine, follow these steps:
3.1. Generating a New Engine
First, install Rails (if not already installed) and create a new Rails engine using the following command:
bash rails plugin new MyEngine --mountable --full
This command creates a new engine named “MyEngine” with the –mountable option indicating that it should be mountable in other applications and the –full option including all possible folders and files for a complete engine.
3.2. Structure of a Rails Engine
A Rails Engine has a structure similar to a regular Rails application. Here are some important directories in the engine’s structure:
- app: Contains the engine’s controllers, models, views, helpers, and assets.
- config: Includes the engine’s configuration files, routes, and initializers.
- lib: Houses any additional libraries or modules that the engine uses.
- spec: Holds the engine’s test files.
3.3. Engine Routes and Mounting
In the engine’s config/routes.rb file, you can define routes specific to the engine. These routes will be isolated from the main application’s routes. To mount the engine in the main application, add the following line to the main application’s config/routes.rb file:
ruby mount MyEngine::Engine, at: '/my_engine'
3.4. Isolating the Engine’s Namespace
By default, the engine’s controllers, models, and views are namespaced under the engine’s name. For example, if your engine is named “MyEngine,” the controllers will be under MyEngine::Controllers, models under MyEngine::Models, and so on. You can isolate the engine further by adding a namespace:
ruby module MyEngine class ApplicationController < ActionController::Base # Your code here end end
4. Sharing Functionality with Mountable Engines
Mountable engines provide a powerful way to share functionality across multiple applications. Let’s create a basic mountable engine that implements user authentication.
4.1. Building a Mountable Engine
In your main Rails application, create a new engine named “AuthEngine” using the following command:
bash rails plugin new AuthEngine --mountable --full
4.2. Mounting the Engine in the Main Application
In the main application’s config/routes.rb file, mount the AuthEngine like this:
ruby mount AuthEngine::Engine, at: '/auth'
4.3. Overriding Engine Functionality
In the main application, you can override engine functionality by defining the corresponding controllers or views. The main application will use these instead of the engine’s default ones.
5. Testing Rails Engines
Testing Rails engines is crucial to ensure they work correctly and independently. Here’s how to test your engines effectively.
5.1. Writing Unit Tests for Engines
In the engine’s spec directory, create separate test files for controllers, models, and other components. Use RSpec or your preferred testing framework to write unit tests for each component.
5.2. Testing Engine Integration with the Main Application
For integration testing, use Rails’ built-in system tests or tools like Capybara to test the engine’s functionality when mounted in the main application.
6. Managing Dependencies in Engines
Managing dependencies in engines is vital to prevent conflicts and ensure smooth integration with the main application.
6.1. Gem Dependencies
Specify gem dependencies in the engine’s .gemspec file, just like in a regular gem. Avoid adding dependencies that conflict with the main application’s gems.
6.2. Engine Dependencies
If your engine relies on functionality from another engine, ensure you declare the dependency explicitly in the engine’s .gemspec file.
7. Packaging and Distributing Rails Engines
To share your engine with others, you can package it as a gem and distribute it through RubyGems or a private gem server.
Conclusion
Understanding Rails Engines unlocks a world of modularity and code reusability in your Rails applications. By encapsulating functionality in engines and leveraging mountable engines, you can build more maintainable and scalable projects. Experiment with engines in your next Rails project and witness the benefits firsthand. Happy coding!
In this comprehensive tutorial, we’ve explored the concept of Rails Engines, their advantages, how to create and mount them, testing strategies, managing dependencies, and packaging for distribution. Armed with this knowledge, you’re ready to take your Ruby on Rails development to the next level with the power of Engines. Happy coding!
Table of Contents
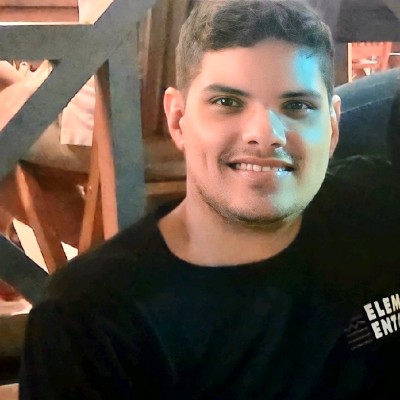
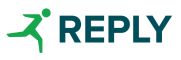