Ruby on Rails Tutorial: Understanding Error Handling and Exception
Ruby on Rails is a powerful and popular web development framework that allows developers to build robust and scalable web applications quickly. Like any other software, Rails applications are not immune to errors and exceptions. These unexpected issues can disrupt the normal flow of your application and negatively impact user experience. To ensure your Rails application runs smoothly, it’s essential to understand error handling and exception management.
Table of Contents
In this tutorial, we will delve deep into error handling and exception management in Ruby on Rails. We’ll explore why error handling is crucial, the different types of errors and exceptions you may encounter, and best practices for effectively managing them. By the end of this tutorial, you’ll be equipped with the knowledge and tools to handle errors gracefully and improve the reliability of your Rails applications.
1. Why Error Handling Matters
Before we dive into the specifics of error handling in Ruby on Rails, let’s understand why it matters. Error handling is an integral part of software development for several reasons:
1.1. Enhancing User Experience
When an error occurs in your Rails application, how you handle it can greatly impact the user experience. A well-handled error provides users with meaningful feedback and guidance on how to proceed, reducing frustration and improving overall satisfaction.
1.2. Debugging and Maintenance
Effective error handling aids in the debugging process. It provides developers with valuable information about what went wrong and where, making it easier to diagnose and fix issues. Properly handled exceptions also make your codebase more maintainable.
1.3. Security
In some cases, errors can expose sensitive information or vulnerabilities in your application. Proper error handling can help prevent these security risks by ensuring that error messages don’t leak sensitive details.
1.4. Reliability
Robust error handling makes your application more reliable and resilient. It allows your application to recover gracefully from unexpected issues, minimizing downtime and ensuring a smoother user experience.
Now that we understand the importance of error handling, let’s explore the different types of errors and exceptions you may encounter in a Ruby on Rails application.
2. Types of Errors and Exceptions in Rails
Rails applications can encounter various types of errors and exceptions during their lifecycle. Understanding these categories will help you handle them effectively. Here are some common types:
2.1. Syntax Errors
Syntax errors are the most basic type of error and occur when there is a problem with the code’s structure. These errors are caught during the code compilation phase and must be fixed before the application can run.
ruby # Syntax Error Example def missing_end puts "This is missing an 'end'" end
2.2. Runtime Errors
Runtime errors occur during the execution of the code and are often caused by unexpected data or conditions. These errors can include division by zero, undefined method calls, or attempting to access a nil object.
ruby # Runtime Error Example def divide_by_zero 10 / 0 end
2.3. Standard Errors
Standard errors are a broad category of exceptions that inherit from the StandardError class. These include common exceptions like ArgumentError, NameError, and NoMethodError. You can rescue these exceptions to handle specific issues in your code.
ruby # Handling a Standard Error begin # Code that may raise an exception rescue StandardError => e puts "An error occurred: #{e.message}" end
2.4. Custom Exceptions
In addition to standard exceptions, you can define custom exceptions in your Rails application. These are useful when you want to handle specific errors unique to your application’s domain.
ruby # Defining a Custom Exception class CustomError < StandardError def initialize(message) super(message) end end
2.5. Database Errors
Rails applications often interact with databases, and errors can occur during database operations. These errors can include database connection failures, record not found, or data integrity issues.
ruby # Handling a Database Error begin # Database operation that may raise an exception rescue ActiveRecord::RecordNotFound => e puts "Record not found: #{e.message}" rescue ActiveRecord::StatementInvalid => e puts "Database error: #{e.message}" end
Now that you have a better understanding of the types of errors and exceptions in Rails, let’s move on to exploring how to handle them effectively.
3. Best Practices for Error Handling in Ruby on Rails
Handling errors gracefully in your Rails application is essential for maintaining a positive user experience and ensuring the reliability of your software. Here are some best practices for error handling in Ruby on Rails:
3.1. Use rescue Blocks
The rescue keyword in Ruby allows you to catch and handle exceptions. Use begin and rescue blocks to wrap code that might raise exceptions, ensuring that your application can recover from errors.
ruby begin # Code that may raise an exception rescue SomeException => e # Handle the exception end
3.2. Provide User-Friendly Error Messages
When an error occurs, display clear and user-friendly error messages. Avoid showing technical details to users, as this can confuse and frustrate them. Log the detailed error information for debugging purposes, but show a simplified message to the user.
ruby begin # Code that may raise an exception rescue SomeException => e flash[:error] = "Oops! Something went wrong. Please try again later." # Log the detailed error for debugging Rails.logger.error("Error: #{e.message}") end
3.3. Log Errors
Logging is crucial for diagnosing and fixing errors in a Rails application. Use the Rails logger to record error details, including the stack trace, so you can analyze the issue later.
ruby begin # Code that may raise an exception rescue SomeException => e Rails.logger.error("Error: #{e.message}") # Handle the exception end
3.4. Handle Specific Errors
While it’s essential to handle exceptions gracefully, it’s equally important to differentiate between different types of errors. Handle specific exceptions based on their context and impact on your application.
ruby begin # Code that may raise a specific exception rescue SpecificException => e # Handle this specific exception rescue AnotherException => e # Handle another specific exception end
3.5. Use Custom Exceptions
Create custom exceptions for your application to represent domain-specific errors. This allows you to handle application-specific issues more effectively.
ruby class CustomError < StandardError def initialize(message) super(message) end end begin # Code that may raise a custom exception rescue CustomError => e # Handle the custom exception end
3.6. Implement Error Pages
Create custom error pages for common HTTP error codes like 404 (Not Found) and 500 (Internal Server Error). These pages can provide users with helpful information and navigation options.
ruby # In config/application.rb config.exceptions_app = self.routes # In routes.rb match "/404", to: "errors#not_found", via: :all match "/500", to: "errors#internal_server_error", via: :all
3.7. Test Error Handling
Include tests for error handling in your test suite. Ensure that your application behaves as expected when errors occur and that the appropriate error messages are displayed.
ruby # Example RSpec test for error handling it "displays an error message when login fails" do # Simulate a failed login attempt allow(User).to receive(:authenticate).and_return(nil) post :create, params: { email: "user@example.com", password: "wrong_password" } expect(flash[:error]).to be_present end
4. Handling Errors in Controllers and Views
In a Ruby on Rails application, errors can occur in controllers and views. Here’s how to handle them in both contexts:
4.1. Handling Errors in Controllers
In Rails controllers, you can use the rescue_from method to handle exceptions globally. This allows you to centralize error handling for specific exception types.
ruby class ApplicationController < ActionController::Base rescue_from ActiveRecord::RecordNotFound, with: :record_not_found def record_not_found render plain: "Record not found", status: :not_found end end
4.2. Handling Errors in Views
In your views, you can use conditionals to display error messages based on the presence of flash messages set in your controllers.
erb <% if flash[:error].present? %> <div class="alert alert-danger"> <%= flash[:error] %> </div> <% end %>
By implementing these practices, you can effectively handle errors and exceptions in your Ruby on Rails application, providing a better user experience and making your application more robust.
Conclusion
Error handling and exception management are crucial aspects of building reliable and user-friendly Ruby on Rails applications. By following best practices and understanding the types of errors and exceptions that can occur, you can create applications that gracefully handle unexpected issues, enhance user satisfaction, and simplify debugging and maintenance.
Remember that error handling is an ongoing process. Continuously monitor and improve your application’s error-handling mechanisms to ensure its stability and security. With the knowledge gained from this tutorial, you’re well-equipped to tackle error handling in your Ruby on Rails projects and build robust web applications that stand the test of time.
Table of Contents
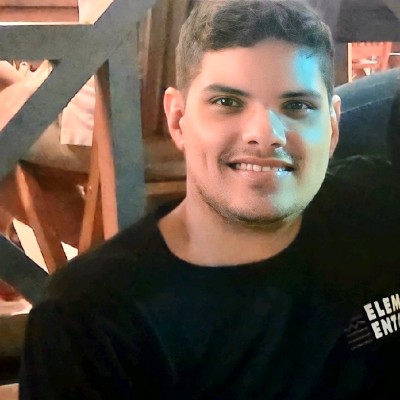
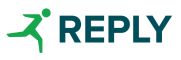