How to Use Ruby Functions for File Manipulation
In the ever-evolving sphere of programming, file manipulation is a common operation that developers often encounter. Ruby, a popular high-level language known for its simplicity and readability, offers numerous built-in functions to handle files, and in this post, we will explore them in detail. Whether you’re a seasoned Rubyist or just getting your feet wet, this guide will equip you with the knowledge to perform basic and complex file manipulations using Ruby.
Basics of File Operations in Ruby
At the heart of file manipulation in Ruby is the `File` class, which provides a plethora of methods to handle files. The core operations you can perform include creating, reading, writing, renaming, and deleting files.
Opening and Closing Files
Before manipulating files, you need to open them, and Ruby provides the `File.open` method. This method accepts two arguments: the file path and the mode (optional).
file = File.open("test.txt", "r") # Your code here file.close
The mode tells Ruby what you want to do with the file. Some common modes are:
– “r”: Read-only mode, starting at the beginning of the file.
– “w”: Write-only mode. If the file exists, it overwrites the file. If it doesn’t, it creates a new file.
– “a”: Write-only mode, starting at the end of the file if it exists. If it doesn’t, it creates a new file.
Remember to close the file after you finish working with it, using the `close` method. Not closing files can lead to data loss or other issues.
Reading Files
Ruby provides several methods for reading files. One simple way is to use the `read` method, which returns the file content as a string.
file = File.open("test.txt", "r") puts file.read file.close
If you want to read the file line by line, you can use the `readlines` or `each_line` method.
file = File.open("test.txt", "r") file.readlines.each { |line| puts line } file.close
Writing to Files
Writing or appending data to a file in Ruby is simple. For writing, you use the “w” mode, and for appending, the “a” mode.
# Writing to a file file = File.open("test.txt", "w") file.puts("Hello, Ruby!") file.close # Appending to a file file = File.open("test.txt", "a") file.puts("Hello again, Ruby!") file.close
File Manipulation Methods in Ruby
Beyond reading and writing, Ruby provides other useful file manipulation methods.
Renaming and Deleting Files
The `rename` method renames a file, and the `delete` method removes it. Both methods are part of the `File` class, not an instance.
# Renaming a file File.rename("old_name.txt", "new_name.txt") # Deleting a file File.delete("test.txt")
Checking File Existence and Details
You can check if a file exists with the `exist?` method and gather details like size, creation time, and modification time using `size`, `ctime`, and `mtime`.
# Checking if a file exists puts File.exist?("test.txt") # Outputs true or false # Getting file size, creation time, and modification time puts File.size("test.txt") puts File.ctime("test.txt") puts File.mtime("test.txt")
Working With Directories in Ruby
Just like files, Ruby allows manipulation of directories. You can create, rename, and delete directories, and read their contents.
Creating, Renaming, and Deleting Directories
Creating a directory uses the `mkdir` method, renaming uses `rename`, and deleting uses `delete` or `rmdir`.
# Creating a directory Dir.mkdir("test_dir") # Renaming a directory File.rename("test_dir", "new_test_dir") # Deleting a directory Dir.delete("new_test_dir")
Reading Directory Contents
The `entries` method provides an array of filenames in a directory, excluding “.” and “..”.
puts Dir.entries("test_dir")
For iterating over directory content, use the `each` method.
Dir.open("test_dir").each { |file| puts file }
A Note on Error Handling
In file manipulation operations, things might not always go as planned. A file or directory might not exist, or a permission issue might occur. It’s crucial to handle these possibilities, and Ruby’s exception handling system provides an excellent way to do this.
begin file = File.open("non_existent_file.txt", "r") rescue Errno::ENOENT puts "File does not exist." end
Conclusion
This guide barely scratches the surface of Ruby’s capabilities for file and directory manipulation. Ruby’s rich set of features makes it a powerful tool for dealing with filesystem operations. Remember to follow best practices such as closing files after use and handling potential errors to ensure your code is robust and reliable.
Table of Contents
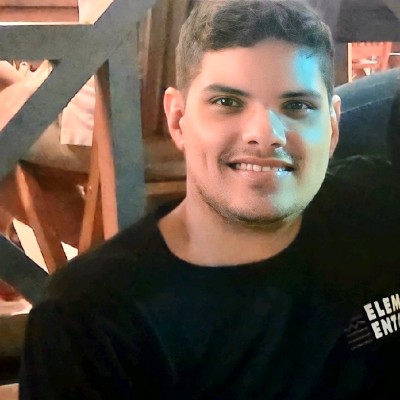
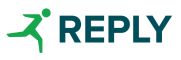