What is the difference between `find` and `where` in Rails?
In Rails’ ActiveRecord, both `find` and `where` are methods used to retrieve data from the database. However, they serve different purposes and have distinct behaviors:
- Usage:
– find: This method is primarily used to retrieve records by their primary key. For instance, `User.find(1)` will return the user with an ID of 1.
– where: This method allows for more general queries and returns an ActiveRecord relation. For example, `User.where(first_name: ‘John’)` retrieves all users with the first name ‘John’.
- Return Value:
– find: It returns a single record when given a single ID or multiple records when provided with an array of IDs. For instance, `User.find(1, 2)` returns the users with IDs 1 and 2.
– where: Always returns an ActiveRecord::Relation, even if only one record matches the query. This means you can chain additional query methods onto it.
- Handling No Matches:
– find: If no record is found, it raises an `ActiveRecord::RecordNotFound` exception. This often translates to a 404 error in a Rails application.
– where: If no records match the criteria, it returns an empty ActiveRecord::Relation, not an error.
- Flexibility:
– find: Primarily suited for lookups by primary key.
– where: Offers flexibility in forming conditions. For instance, you can use SQL-like fragments such as `User.where(“created_at > ?”, Time.now – 7.days)`.
- Chaining:
– find: Cannot be chained with other query methods because it doesn’t return an ActiveRecord relation (unless you’re using `find_by` which is a different method but similar in behavior to `where`).
– where: Easily chainable with other ActiveRecord query methods like `order`, `limit`, and `group`.
While both `find` and `where` help retrieve data in Rails, they have different use cases. `find` is specific and direct, ideal for retrieving by ID, whereas `where` provides a flexible and chainable approach suitable for more complex queries.
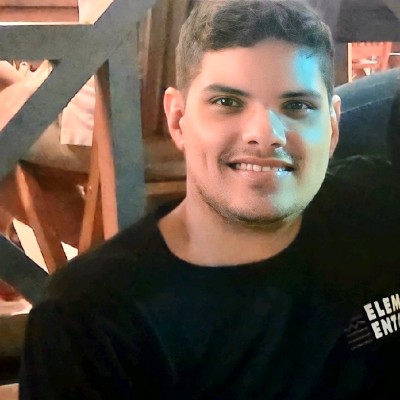
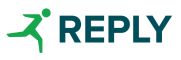