Ruby on Rails Tutorial: Understanding Forms and Input Validation
Forms are an integral part of web applications as they allow users to interact with the system by submitting data. In Ruby on Rails, handling forms and validating user input is made easy with built-in features and conventions. In this tutorial, we will explore the fundamentals of forms and input validation in Ruby on Rails applications. We will cover topics such as form creation, form helpers, input validation techniques, and best practices to ensure secure and user-friendly web applications.
Understanding Forms in Ruby on Rails
Before diving into the intricacies of forms and input validation in Ruby on Rails, it’s essential to have a solid understanding of how forms work in web development. A form is an HTML element used to collect user input and send it to the server for processing. In Rails, forms are created using the form_for or form_with helpers, which generate the necessary HTML and handle form submission.
Creating Forms in Rails Applications
In Ruby on Rails, creating forms is a straightforward process. Let’s consider an example of a blog application where users can submit comments on articles. To create a form for submitting comments, we would start by generating a new comment model and associated migration using the Rails command line:
ruby $ rails generate model Comment content:text article:references $ rails db:migrate
Next, we can create a new comment form in the app/views/comments/new.html.erb file using the form_for helper:
ruby <%= form_for @comment do |f| %> <%= f.text_area :content %> <%= f.submit 'Submit' %> <% end %>
In this example, the form_for helper is bound to the @comment instance variable, which represents a new comment object. The f.text_area helper creates a text area input for the comment content, and f.submit generates a submit button. When the form is submitted, it will be routed to the appropriate controller action for further processing.
Form Helpers for Efficient Development
Ruby on Rails provides a variety of form helpers to simplify form creation and improve development efficiency. These helpers generate HTML input elements with appropriate attributes and handle things like label generation, error messages, and more. Let’s explore a few commonly used form helpers:
- text_field: Creates a single-line text input field.
- password_field: Creates a password input field.
- check_box: Creates a checkbox input field.
- radio_button: Creates a radio button input field.
- select: Generates a dropdown list.
- collection_select: Generates a dropdown list from a collection of objects.
- date_select and time_select: Generate date and time selection fields, respectively.
- file_field: Creates a file upload input field.
These form helpers greatly simplify the process of creating forms by handling HTML generation and providing convenient methods for working with form inputs.
Input Validation with Rails Models
Input validation is crucial to ensure that the data submitted via forms meets certain criteria and is suitable for processing. In Ruby on Rails, input validation is typically handled at the model level using validations. Validations are rules defined within model classes that specify constraints on attribute values. Rails provides numerous validation helpers that can be used to validate input data, such as:
- presence: Ensures that a field is not empty.
- length: Validates the length of a field.
- numericality: Validates that a field is a number.
- format: Validates the format of a field using a regular expression.
For example, to validate that the comment content is not empty and does not exceed a certain length, we can add the following code to the Comment model:
ruby class Comment < ApplicationRecord validates :content, presence: true, length: { maximum: 500 } end
With this validation in place, if a user tries to submit an empty comment or a comment exceeding 500 characters, Rails will prevent the comment from being saved and display appropriate error messages.
Custom Validation Rules and Error Handling
In addition to the built-in validation helpers, Ruby on Rails allows you to define custom validation rules based on your application’s specific requirements. Custom validations are created by adding methods to the model and using the validate or validate_each methods.
For example, let’s say we want to ensure that a comment’s content does not contain any offensive language. We could define a custom validation method in the Comment model like this:
ruby class Comment < ApplicationRecord validates :content, presence: true, length: { maximum: 500 } validate :check_for_offensive_language private def check_for_offensive_language if content.include?('offensive_word') errors.add(:content, 'contains offensive language') end end end
In this example, the check_for_offensive_language method checks if the comment’s content includes the word ‘offensive_word’. If it does, an error message is added to the :content attribute. Custom validation methods provide flexibility and allow you to enforce specific business rules.
Protecting Against Cross-Site Request Forgery (CSRF)
Cross-Site Request Forgery (CSRF) is a security vulnerability where an attacker tricks a user into performing unwanted actions on a web application. Ruby on Rails provides protection against CSRF attacks out of the box. When using the form_for or form_with helpers, a CSRF token is automatically included in the form. The token ensures that the form submission originates from the application and not an external source.
To enable CSRF protection in Rails, the protect_from_forgery method is added to the base controller class. This method generates a unique token for each user session and verifies it on form submission.
Best Practices for Forms and Input Validation
When working with forms and input validation in Ruby on Rails, it’s essential to follow certain best practices to ensure secure and user-friendly applications. Here are some recommendations:
- Use appropriate form helpers and validation helpers to simplify development.
- Perform server-side validation to ensure data integrity.
- Sanitize user input to prevent potential security vulnerabilities.
- Provide meaningful error messages to guide users in fixing input errors.
- Implement client-side validation to enhance the user experience.
- Utilize CSRF protection to guard against malicious attacks.
- Regularly update and patch your Rails application to benefit from security enhancements and bug fixes.
Conclusion
In this tutorial, we explored the fundamentals of forms and input validation in Ruby on Rails applications. We learned how to create forms using the form_for helper, leverage form helpers for efficient development, and implement input validation with Rails models. Additionally, we covered custom validation rules, error handling, and CSRF protection.
By understanding and applying these concepts, you’ll be able to create robust and secure web applications using Ruby on Rails. Remember to adhere to best practices and continuously enhance your knowledge to stay up-to-date with the latest developments in Rails and web application security. Happy coding!
Table of Contents
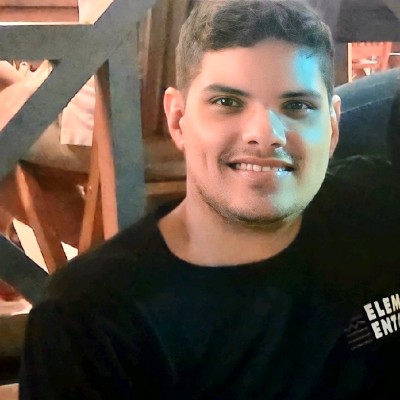
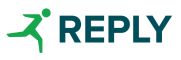